Student Record Management System in C++
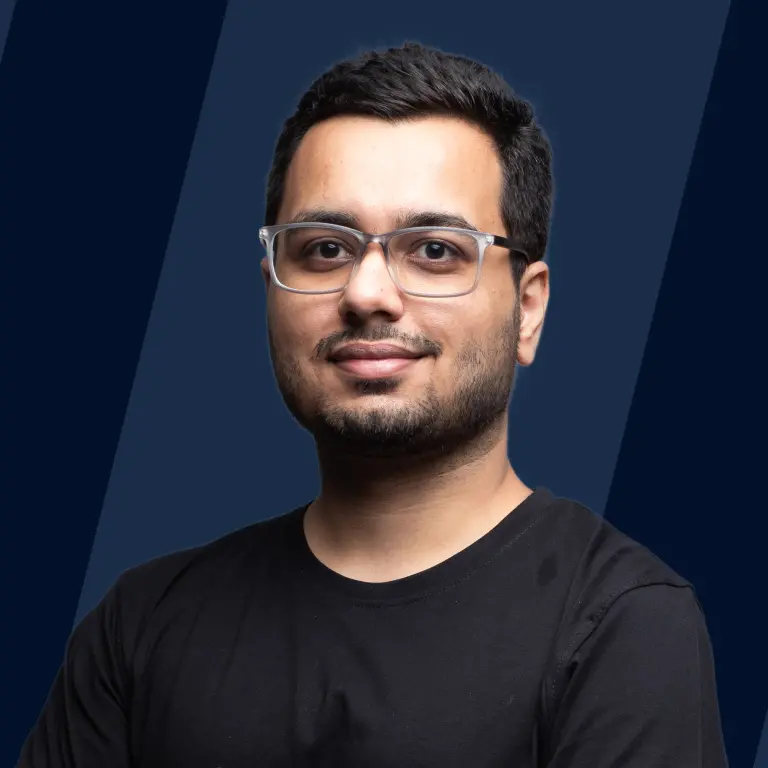
As we know, the internet cannot exist without databases, which makes databases a topic of most importance. These days, databases store millions of bytes of data on servers distributed all around the world. In the student record management system in C++, we will explore different aspects of this database and implement methods to manage it.
What are we Building?
Here, we are trying to build a console application, which is nothing but an application that accepts input and outputs it to a command-line console. This console application is used to manage the database of students, and we will be able to perform tasks like adding a student to the database, updating details of an existing student in the database, searching for a student in the database, etc.
a) Pre-requisites
b) Key Features of the project
-
Add student details: Get information from the user and add it to the database. Verify that each student’s roll number is distinct before adding them to the database.
-
Find the student by the given roll number: This method takes a roll number as input from the user and searches for the student with that number in the database.
-
Find the student by the given first name: This method takes the first name as input from the user and searches for the student with that first name in the database.
-
Find the students registered in a course: This method takes the user's course ID as input and searches the database for students enrolled in that particular course.
-
Count of Students: Print the total number of students in the database.
-
Delete a student: This function deletes the student record for the specified roll number.
-
Update Student: This feature is used to update student records. The user should be allowed to pick and choose whatever fields they wish to update because this method does not request updated information for every field.
c) How are we going to build the survey form? We will use an incremental approach to build this project; below are the steps:
- First, we define the C++ structure, which contains information such as the student's name, roll number, CGPA, etc.
- We define functions that help us manage the database. These functions involve adding new students, updating student information, searching students based on roll number, counting the total number of students in the database, etc.
- Finally, we use the above-defined functions through the main function, our driver code, a menu-driven program displaying choices to the user when performing a particular task.
d) Final Output This image represents our complete application. It displays all the tasks that we can perform on our student database.
Requirements
- We need a C++ environment, i.e. code editor to write the code and a compiler to compile and run the code.
- String library in C++ which can be included using #include<string>.
- Library for input and output in C++ which can be included using #include<iostream>.
Building Student Record Management System in C++
Steps to make the project:
1. Defining the C++ Structure to store the information of students and a global variable number_of_students to store the count of students in the database.
Explanation : The above structure contains string first_name, string last_name, int roll_number, double cgpa, and integer array of course ids of the courses that a particular student is enrolled in. The array students[110] is an array of student structures that can store a maximum of 110 students in the database. Integer number_of_students stores the count of students in the database. Since we are using a string in the code hence we are including a string library and also an input-output library for taking input and displaying output on the console.
2. Defining add student method. This method takes user input for the various fields present in the student structure and adds the student to the database.
Output :
Explanation: In the above code, we first ask the user to enter the roll number of the student he/she wants to insert. If the student is already present in the database, we do not add it. Otherwise, we take other details of the student, like the first name, last name, CGPa, and all the course IDs, and at last, we increase the total student count by 1.
3. Defining find student by roll number method. This method takes the roll number as input from the user and searches for the student with that roll number in the database; if the roll number is found, it prints the student's details; otherwise, it prints no such student with the given roll number.
Output:
Explanation : In the above code first, we ask the user to enter the roll number of the student he/she wants to search. If the student with the given roll number is found then it prints the details of that student otherwise it prints no such student.
4. Defining find student by first name method. This method takes the first name as input from the user and searches for the student with that first name in the database, if the first name is found then it prints the details of that student otherwise it prints no such student with the given first name.
Output:
Explanation : In the above code first, we ask the user to enter the first name of the student he/she wants to search for. If the student with the given first name is found then it prints the details of that student otherwise it prints no such student.
5. Defining find student by course id method. This method takes course id as input from the user and searches those students that are enrolled in that particular course in the database.
Output:
Explanation: In the above code first, we ask the user to enter the id of the student he/she wants to search for. If the student with the given id is found then it prints the details of that student otherwise it prints no such student.
6. Defining find total number of students in the database method.
This method counts the total number of students present in the database. As we were maintaining a variable number_of_students for maintaining the total number of students so this method simply prints the value of that variable.
Output:
The total number Students are 1
You can have maximum of 100 students in the database
7. Defining delete student by roll number method. This method takes the roll number as input from the user and removes it from the database.
Output:
Explanation: In the above code first, we ask the user to enter the roll_number of the student he/she wants to delete. If the student is found then we delete it by copying the values of the students present on the right side of the array and reduce the count of students by 1.
8. Defining update student details method. This method takes the roll number of the student whose details you want to update as input from the user and when the roll number is found in the database then it asks the user which details he/she wants to update.
Output:
Explanation: In the above code first, we ask the user to enter the roll_number of the student he/she wants to update. If the student is found then we ask the user which field i.e first name, last name, cgpa, or courses he/she wants to update and update it accordingly. If the student is not found then we print no such student.
9. Defining main method. This is our driver code which provides choices to the user about the tasks he/she wants to perform.
Output:
Explanation: The above code displays various choices that a user can make on the student database. First, the program takes the input of a variable choice and then decides which method it should call according to the choice of the user switch case.
What’s Next
More features that we can implement:
- To search for a student, whether it exists in the database or not, we can use hashmaps to store every student's roll number. When checking the roll number, we can use the hashmap to find the student in less time.
- We can add the total attendance count to the structure, which can be used to store the attendance count of every student.
- We can add a marks list for every student in the database and create a method for retrieving students according to the marks obtained in ascending or descending order.
Conclusion
- Structures in C++ are user-defined data types that store different data types in a single place.
- A menu-driven program displays every feature of the project and provides the user with choices for the tasks he/she wants to perform.
- Console application, which is nothing but an application that takes input and displays output at a command-line console.
- The switch statement in C++ evaluates the expression provided to the switch, compares it with the cases present in the switch's block scope, and then executes the code corresponding to the matched case.