Substring in C++
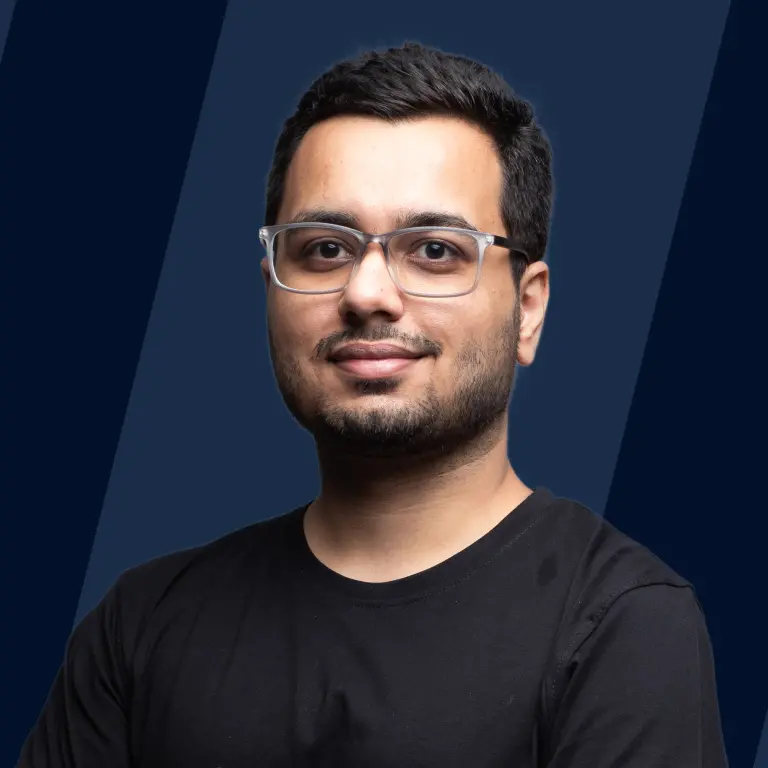
A substring in C++ is extracted using the substr() method, defined in the string.h header file. This method operates on a string, returning a new string object based on two parameters: the starting position and the length of the substring from this position. It retrieves a portion of the original string according to the specified parameters, ensuring the starting position is zero or more.
Syntax
Let's understand the syntax of the substr() method.
The substr() function takes two parameters, starting_Position, the position where we want to get the substring from the original substring, and the total length of the substring. If the second parameter is not present or the second parameter exceeds the total length of the String, the second parameter value will be the length of the original string.
Parameters
The function, Substring in C++ takes two parameters: the starting_position, and the value lies in the range of [0-len(string)]. The len(string) is the total length of the original string. The second parameter is optional.
Return Value
The substr in C++ returns a newly constructed String object.
In this way, we can handle the newly constructed string object.
Examples of Substr in c++
Output:
Time complexity: O(N) - where N is the length of the original string. Space complexity: O(N) - additional space is proportional to the size of the substrings created.
More Examples
Consider these examples:
- Attempting to use an iterator with substr, such as s.substr(s.begin(), 3), results in a compilation error due to type mismatch (iterators cannot be implicitly converted to integers).
- For s.substr(3,2), the output is it - extracts two characters starting from index 3.
- Utilizing s.substr(*s.begin() - s[0], 4) cleverly yields frui - calculating the start index as 0 from the difference *s.begin() - s[0], then taking the first four characters.
- s.substr(6,2) results in pa - a substring starting at index 6 for two characters.
- s.substr(9,0) produces an empty string - selecting zero characters starting from index 9, illustrating how specifying a length of 0 results in no output.
Important Points to Remember
When working with Substring function in c++, keep these crucial aspects in mind:
- The indexing of characters in a string starts at 0, not 1.
- When the starting position (pos) matches the string's length, the method yields an empty string.
- Specifying a pos beyond the string's length triggers an out_of_range exception, without altering the original string.
- If the length (len) specified for the substring exceeds the string's remaining length from pos, the substring returned spans from pos to the end of the string, denoted as [pos, size()).
- Omitting the len parameter results in a substring that extends from pos to the string's end, also noted as [pos, size()).
Applications of Substr in C++
- Extracting a Substring Following a Specific Character
- Retrieving a Substring Post a Designated Character
- Displaying Every Substring within a Specified String
- Calculating the Total of All Numeric Substrings within a String
- Determining the Highest Numeric Value among All Substrings of a String
- Identifying the Lowest Numeric Value among All Substrings of a String
Other Important Applications of Substr in C++
- Text Searching: Substrings are crucial for searching specific phrases in large texts, key for search engines to match queries with relevant content.
- Text Parsing: They're vital for breaking down texts into smaller parts, aiding in extracting words for processing.
- Text Manipulation: Substrings are pivotal for the find-and-replace functionality in text editing tools, allowing users to modify specific portions of text.
- Natural Language Processing (NLP): In NLP, substrings are crucial in identifying and processing words and phrases for applications like speech recognition.
- Pattern Recognition: Useful in identifying patterns or anomalies in data across various sectors like finance.
- Password Security: Employed in verifying passwords by comparing segments, enhancing security without revealing the full password.
Conclusion
- This substr() method is the method of the string.h library.
- The substr() method takes two parameters. The second parameter is optional.
- If the second parameter is negative, the parameter automatically assigns to the total length-1 value.
- The first parameter cannot be of a negative number.