JavaScript String substring() Method
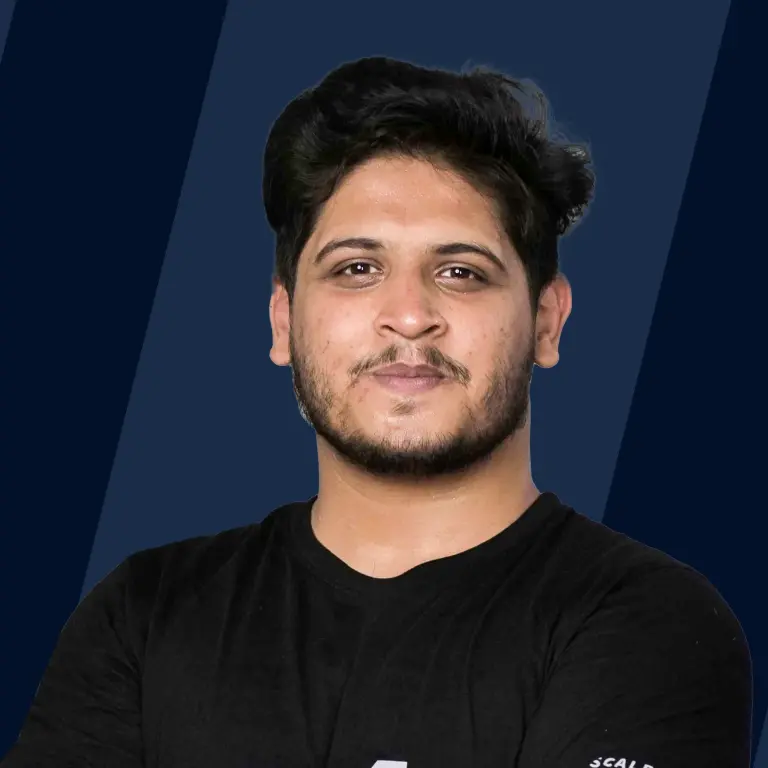
Overview
The substring() method is an inbuilt function in JavaScript that is used to extract a part of the string. Two parameters are passed to the function, i.e., the starting index and the ending index that tells the function which part of the string to grab. If the passed argument is negative or NaN, they are treated as 0, and if the arguments are greater than the string length then they are treated equal to string.length.
Syntax of String substring() in Javascript
To extract substring in javascript we can use following syntax
string_object should be the instance of String class.
Parameters of String substring() in Javascript
The substring() function accepts two function parameters that are:
- StartIndex: This value tells the position in the string where the extraction will start, or it is the index of the first character to include in the returned substring.
- EndIndex (optional): It is the index of the first character to exclude from the returned substring. If this parameter is not provided, the function returns all the characters to the end of the string which makes this parameter optional.
These two parameters help in extracting substring in javascript.
Return value of String substring() in Javascript
The substring() method returns a substring in javascript that has been extracted from the string based on the parameters (StartIndex, EndIndex) provided.
Note: The substring method does not change the value of the original string.
What is String substring() in JavaScript?
When we do a google search, we often see the search result showing a preview of a complete website with small text from the website that is related to our search keyword. To view the complete content, we have to open the website. This is where function like substring() helps to grab a small part (sub-string) from the complete string.
The string.substring() is a built-in function in JavaScript that returns a part of the given string from a starting index to an ending index. The starting and ending indices are passed to the function as function parameters. Also, because substring() is a string method of String object, it must always be invoked through a particular instance of the String class.
Note: Indexing starts from 0
The substring() extracts substring in javascript from StartIndex up to EndIndex(but not include EndIndex). Also,
- If EndIndex is omitted, the method extracts character to the end.
- If StartIndex is equal to EndIndex, the substring() returns an empty string.
- If StartIndex is greater than EndIndex, the effect is the same as if the two arguments were swapped.
- If any argument is less than 0 or NaN, then it is to be treated as 0, and if any of the arguments is greater than string.length, then it is treated as if it was string.length.
Example
Using substring()
The following example uses the substring() method to display characters from the string Hello.
Output
In this example, we are able to see different edge cases that are possible when using the substring function. Let's analyze the output:
- The first two function calls show the case when the first index is greater than the second, the output is same as if the function's parameters are swapped.
- When only the first index is passed, the function returns a string from the index to the end.
- If any of the arguments is greater than string.length, then it is treated as if it was string.length this can be observed as the function call substring(0,10) returns the complete string.
Using substring() with Length Property
Let us see an example where we use the substring() method and the length property to extract the last characters of a particular string. This method might be easier to remember and use as we don't have to remember the starting and ending indices.
Output
Here, we are using the string length property to identify the number of characters we need to extract from the string. Since, only the first index is passed to the function, the function substring() extracts characters till the end of the string.
Replacing a Substring Within a String
In the following example, we are replacing a substring within a string. The program will replace both the individual characters and the substrings. The function call at the end changes the string from "Happy New Year" to "Happy New End".
Output
In the above example, we have created a function that replaces a substring in javascript within the string. The function accepts three arguments, i.e., the old substring that we need to be replaced, the new substring that will replace the old substring and the actual string. The function works by first finding all the substrings in the string with a length equal to the string that needs to be replaced. Once the substring has been found in the string, the original string is changed to the new string by replacing the old substring with the new one using the substring method on the string.
A better method for doing the same task is as follows:
Difference Between substring() and substr()
There is a very subtle difference between the two methods, so you should avoid getting them confused. The arguments of the substring() method represent starting and ending indices. In contrast, the arguments of the substr() method represent the starting index and the number of characters to be included in the returned string.
Also, substr() is considered a legacy feature in ECMAScript and could be removed from future versions.
Output
Here, both the methods receive the same input, but the substr print characters starting from index 1 till the count of characters reaches 3 whereas substring print characters starting from index 1 till index 3(not including last index character).
Difference between substring() and slice()
The substring() and slice() methods are almost identical, and they have very small differences between them, especially in the way both methods handle negative arguments.
- If the starting index is greater than the ending index, the substring() method swaps its two-argument and returns a string, whereas the slice() method, in this case, returns an empty string.
Output
Here, slice(3,1) does not print any string because in slice method if first index in greater than the second it returns an empty string.
- If either of the two arguments is negative or NaN, the substring() method treats it as if they were 0.
Output
slice() method also treats NaN arguments as 0, but when a negative number is passed to the function, it counts backward from the end of the string to find the indexes.
Output
Here, slice method is counting the index backward from the end in order to find the indices in the string.
Conclusion
- The string.substring() is a built-in function in JavaScript that returns a part of the given string from starting index to the ending index.
- The substring() method accepts two function parameters: starting and ending index that tells the function which part of the string to grab.
- The substring() method returns a substring that has been extracted from the string based on the parameters (StartIndex, EndIndex) provided without affecting the original string.
- If the passed arguments are negative or NaN, then they are treated as 0, and if the passed arguments are greater than string.length, then they are treated as string.length.
See Also
You can read more articles on some of the advance concepts in C++ like: