JavaScript Program to Add Digits of a Number
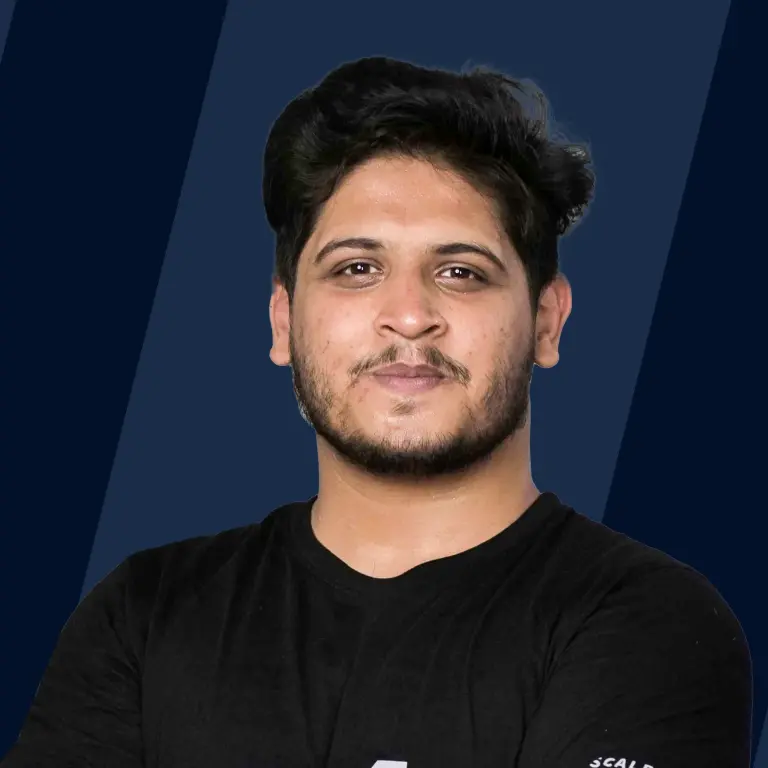
Overview
Adding the digits of a number is one of the simplest programs that help in understanding the working of loops. If we are given a number, and we need to find the sum of its digits, the simplest approach is to split the number into digits and add each of them in integer form.
Introduction
A positive integer with two or more digits has been provided as input. The objective is to return the sum of all of the number's digits. Let's take a look take an example to understand this clearly:
Example:
Number : 2568
The number 2568 has 4 digits: 2, 5, 6 and 8. When we add these digits: 2 + 5 + 6 + 8 = 21 We obtain 21, which is the desired output.
Add Digits of a Number in JavaScript
Now that we know what our objective is let's use JavaScript to implement this approach in code:
Code:
Output:
- In the code above, firstly, we initialise the variable n with the number.
- We also declare a variable remainder and initialize a variable sumOfDigits as 0.
- Now, we will keep dividing the number by 10 and storing the remainder in the remainder variable while constantly adding the remainder to the sumOfDigits variable.
- At the same time, we also divide the number by 10 so that the digits which are already added are removed, and we keep following steps 3 t0 4 until the number exists, using the while loop.
However, there is another method to find the sum of digits in JavaScript. It is by using the split and reduce methods. Convert the number to a string, split it to generate an array with all digits, then reduce each portion and return the sum.
Output:
Here, we are using the toString() method to convert the number to a string to perform some operations. We are using the split() method to split the digits and using map() to make them the number, and at last, we are using reduce() method to calculate the sum of digits, and this sum is stored in the sum variable.
Example to Add Digits of a Number, Where Input is to be obtained from the User
In this example, we will get the number from the user as an input and then return the sum of its digits.
Conclusion
- Adding the digits of a number means splitting the number into digits and adding each of them to obtain the result.
- To find the sum of digits in JavaScript, there are two approaches, the first is to divide the number and add the remainder to obtain the sum, and the other is by using split and reduce methods in JavaScript.