Sum of Two Arrays in C++
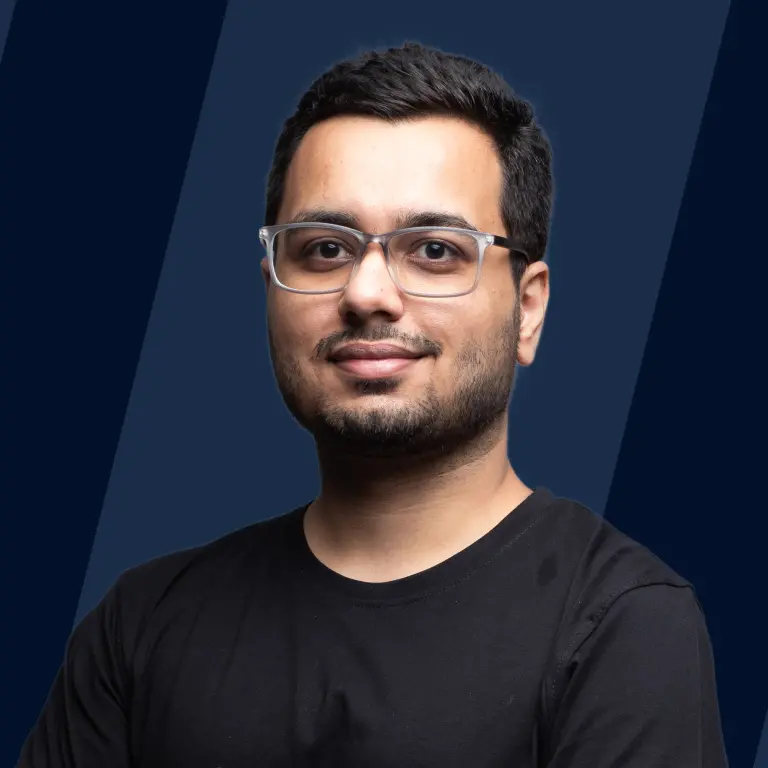
Arrays in programming are containers that hold several values under a single identifier. They make data management easier by letting you organize similar items efficiently. In C++, defining an array requires providing its type and size. For example, int numbers[5] generates an array named numbers that may store five integers. Elements are accessed via indices, which begin with 0. Consider this basic scenario.
This C++ code generates an array and prints its items in a loop. Arrays are fundamental to programming, providing an organised way to manage a wide range of data.
C++ Program to Add Two Arrays
Are you ready to learn more about C++ programming and improve your skills? Today, we'll look at a basic yet vital task: adding two arrays. This procedure is essential in a wide range of applications, including data handling and mathematical computations.
Let us start with the basics. In C++, an array is a collection of elements of the same data type that may be accessed using an index or a key. To add two arrays, sum the corresponding items from each array and store the result in a new array.
Using the For Loop
Our first solution uses the common 'for' loop. This loop pattern is ideal for jobs that require a specific amount of iterations. Here is a piece of code:
In this programme, we set up two arrays, array1 and array2, each with integer values. The for loop goes over each element of the arrays, adding related items and saving the result in a new array called result.
Implementing a While Loop
Now, let's look at the identical array addition operation with the 'while' loop, which is more flexible and can adjust to changing situations. Here is a piece of code:
In this version, the while loop produces the same array addition outcome using a different syntax. The loop adapts to dynamic settings by continuing as long as the condition i < size is true.
Mastering various loop structures adds a degree of expertise to your C++ programming skills. By studying the array addition task using both for and while loops, we gained important insights into its applicability and adaptability. As you continue your coding journey, keep in mind that the decision between these loops is determined by the precise needs of your assignment, with each bringing unique qualities to the table.
Conclusion
- Implementing the sum of two arrays in C++ releases the potential of efficiency since the language's array handling features help to simplify and optimise code performance.
- When it comes to adding two arrays, C++'s flexibility shows through. Its versatility allows it to handle a range of array sizes and kinds, making it an excellent solution for tackling a variety of issues.
- C++ provides developers with great control over memory management and array manipulation. This degree of control assures that the sum of two arrays is not only correct but also completed with the greatest precision.
- C++'s organised syntax helps you write code that is understandable and maintainable. Using straightforward array indexing and arithmetic operations, developers may readily understand, edit, and troubleshoot their code for the sum of two arrays.
- C++ easily integrates with various features like functions, loops, and conditional statements. This integration not only enhances the sum of two arrays but also allows developers to easily learn and implement more complex algorithms.