C++ Ternary Operator
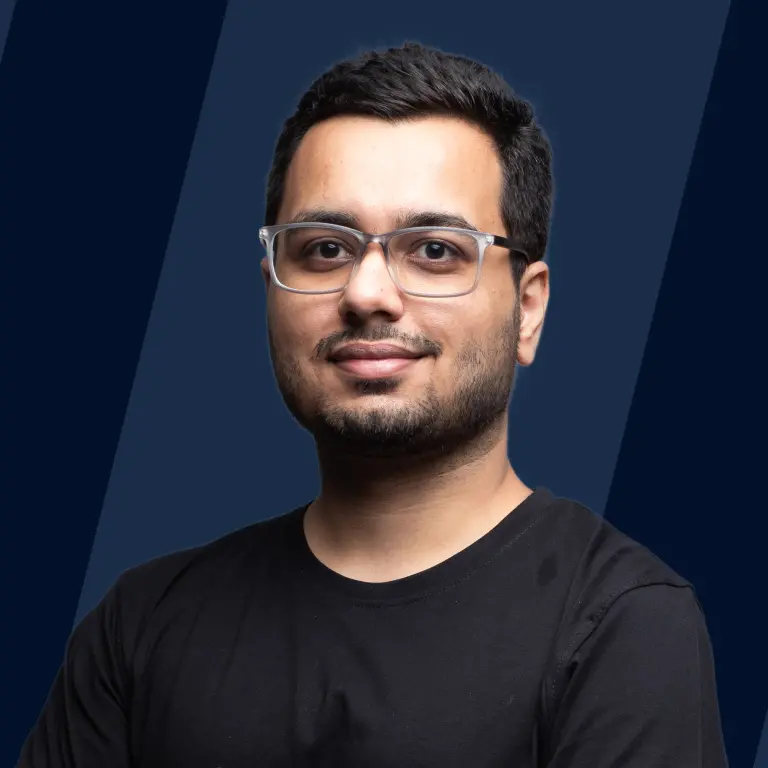
Overview
Ternary operators offer a concise alternative to conventional if-else statements. The format of the ternary operator is simple: it starts with a condition, followed by a question mark (?). If the condition is true, the expression before the colon (:) is executed; otherwise, it moves on to the expressions after the colon (:).
What is Ternary Operator in C++?
Making judgments is an essential activity in the domain of C++ programming. The ternary operator, denoted as condition ? expr1 : expr2, offers an elegant solution to simplify decision-making concisely.
Syntax
The syntax follows a straightforward pattern. The condition is evaluated first. If it's true, expr1 is executed; if false, expr2 takes the stage. This operator condenses an if-else statement into a single line, aiding in cleaner and more readable code.
For example:
In this line, if x is greater than 10, the value of x is assigned to the result; otherwise, result gets the value 10. This concise approach is useful for allocating values depending on circumstances or conducting rapid tests.
Note
Ternary operators offer concise alternatives to traditional if-else statements for simple conditional assignments. They excel in readability and compactness. Nested ternary operators can handle multiple cases within one line, though excessive nesting diminishes code clarity. Compared to lengthy if-else ladders, ternary operators enhance code aesthetics. Choose ternary operators for straightforward decisions; opt for if-else statements for complex choices where readability is paramount.
Examples
Let's delve into a couple of illustrative examples to understand the versatility of the ternary operator:
Example 1: Finding the Maximum
Suppose you must determine the maximum of two numbers, a and b. Instead of using a full if-else block, you can employ the ternary operator:
Output:
In this case, since b (12) is greater than a (8), the value of maxNumber will be 12.
Example 2: Grading Scores
Imagine you're grading student scores and want to assign a grade based on a pass/fail threshold. Here's how the ternary operator can simplify the process:
Output:
Since score (85) is greater than or equal to passingScore (60), the value of grade will be A.
The ternary operator examines the condition in brackets first in these cases. If the condition is met, the value before the colon is chosen; otherwise, the value after the colon is chosen.
By introducing the ternary operator into your C++ code, you may develop simpler, more concise, and easier-to-comprehend reasoning. Remember that while it's a terrific tool, overusing it in complicated cases may influence code readability.
When to Use a Ternary Operator in C++?
Making judgments is crucial to creating efficient and useful code in C++ programming. This is when the ternary operator comes in helpful. The ternary operator acts as a compact shorthand for an if-else expression, enabling you to make rapid decisions without losing readability.
So, when is it appropriate to use the ternary operator's power? It works perfectly when giving a variable a value depending on a condition. The operator assigns one value when the condition is true; otherwise, it assigns another. This condensed decision-making is useful for assignments, comparisons, and minor conditional assignments.
Remember that while the ternary operator simplifies code, balancing brevity and clarity is critical. Traditional if-else formulations are frequently better suited for complex circumstances or many actions. Accept the ternary operator for quick, superficial judgments, and enjoy the elegance it adds to your C++ coding endeavours.
Nested Ternary Operators in C++
Nested ternary operators serve an essential role in C++ decision-making. These operators allow you to create conditional statements in a single line of code. The format of the nested ternary operator, commonly known as a ternary expression, is as follows:
Here, condition1 is evaluated first. If true, result1 is returned; otherwise, condition2 is evaluated. If condition2 is true, result2 is returned; otherwise, result3 is returned.
When used wisely, nested ternary operators may be helpful, but they can also make code less understandable if misused. They are best suited for essential conditional assignments and can aid in condensing several lines of code into a more concise form. However, layering them too deeply might result in clarity and improved code maintainability.
Conclusion
- Ternary operators are a space-saving alternative to standard operators if-else statements.
- The ternary operator has a simple structure: it starts with a condition and ends with a question mark (?), then the expression to execute if the condition is true, a colon (:), and finally, the expression to execute if the condition is false.
- Ternary operators are expressions in and of themselves, which means they return a value. Ternary operators ensure that true and false expressions are type-compatible.
- Although ternary operators can improve code aesthetics, their overuse can cause code to become complicated.
- While ternary operators excel at handling basic circumstances, complicated expressions within the true or false branches can cause code clarity to suffer.