Synchronization in Java
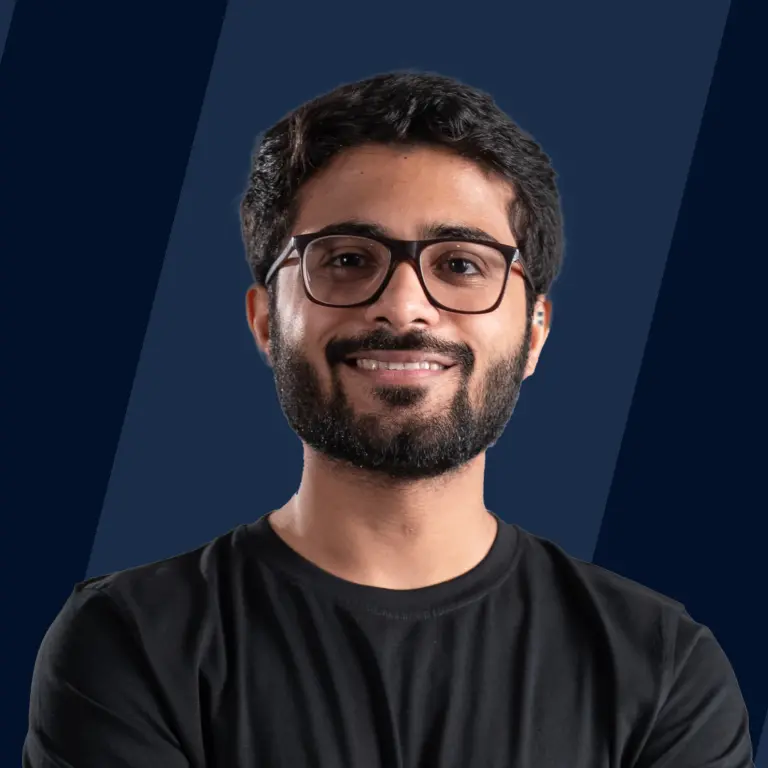
Thread synchronization in Java is a way of programming several threads to carry out independent tasks easily. It is capable of controlling access to multiple threads to a particular shared resource.
Why Use Synchronization?
The main reasons for using thread synchronization in Java are as follows:
- To prevent interference between threads.
- To prevent the problem of consistency.
Types of Synchronization
There are two types of thread synchronization in Java:
- Process synchronization:
Process synchronization ensures that multiple processes in a system coordinate their actions to avoid conflicts and maintain consistency.
- Thread synchronization:
Thread synchronization involves coordinating the execution of threads to ensure proper sharing of resources and prevent data inconsistency in a multithreaded environment.
Thread Synchronization
Thread synchronization refers to the concept where only one thread is executed at a time while other threads are in the waiting state. This process is called thread synchronization. It is used because it avoids interference of thread and the problem of inconsistency. There are two types of thread synchronization in Java:
- Mutual exclusive- It will keep the threads from interfering with each other while sharing any resources.
- Inter-thread communication- It is a mechanism in Java in which a thread running in the critical section is paused and another thread is allowed to enter or lock the same critical section that is executed.
Mutual Exclusive
It is used for preventing threads from interfering with each other and to keep distance between the threads. Mutual exclusive is achieved using the following:
- Synchronized Method
- Synchronized Block
- Static Synchronization
Concept of Lock in Java
The concept of a lock in Java revolves around synchronization, where it's built around an internal entity called the lock or monitor. In Java, every object has a lock associated with it. As per convention, a thread requiring consistent access to an object's fields must acquire the object's lock before accessing them. Subsequently, the lock is released when the thread is finished with the fields. This ensures that only one thread can access the object's synchronized code block at any given time, preventing data corruption or inconsistencies due to concurrent access.
Understanding the Problem without Synchronization
Understanding the problem without synchronization involves recognizing situations where multiple threads accessing shared data simultaneously can lead to data inconsistency or corruption. Without synchronization mechanisms like locks, this can result in race conditions and program errors.
Example:
Output:
Explanation
In this example, class thread1 extends the class thread and has a method run that is void. The class thread2 extends the thread and has a method run that is void. Both are printing tables of different numbers. In the main class, the objects of threads 1 and 2 are created and the method start is invoked.
Example of Java Synchronization
It is a method that can be declared synchronized using the keyword “synchronized” before the method name. By writing this, it will make the code in a method thread-safe so that no resources are shared when the method is executed.
Syntax:
Output:
Explanation:
In the provided code, there's a Table class with a synchronized method printTable, which prints multiples of a given number. Two threads, MyThread1 and MyThread2, are created, each instantiated with the same Table object. Both threads invoke the printTable method with different input parameters. Since the method is synchronized, only one thread can execute it at a time, ensuring that the output is more orderly and synchronized. This prevents interleaving of output lines between different threads, leading to a more predictable and synchronized behavior.
Example of Synchronized Method by Using an Anonymous Class
Using anonymous classes for thread creation, resulting in shorter and more concise code.
Output:
Explanation:
In this program, the Table class has a synchronized method printTable(int n). The main method creates a single instance of the Table class named obj. Two threads (t1 and t2) are created using anonymous classes that override the run method. Each thread invokes the printTable method on the shared Table object obj, with different arguments (2 for t1 and 10 for t2). Due to synchronization, only one thread can execute the printTable method at a time on the shared object, ensuring that the output is synchronized.
Conclusion
- Thread synchronization in Java is used to avoid the error called memory consistency which is caused due to inconsistency view of shared memory.
- In Java, there are two types of thread synchronization:
- Process Synchronization
- Thread Synchronization
- Mutual exclusive
- Inter-thread communication
- If a method is declared synchronized then the thread holds that object of the thread until the other thread is done executing.
- It is used for controlling the access of multiple threads and to access shared resources.
- It avoids the deadlock situations in the thread.