Tic Tac Toe Python
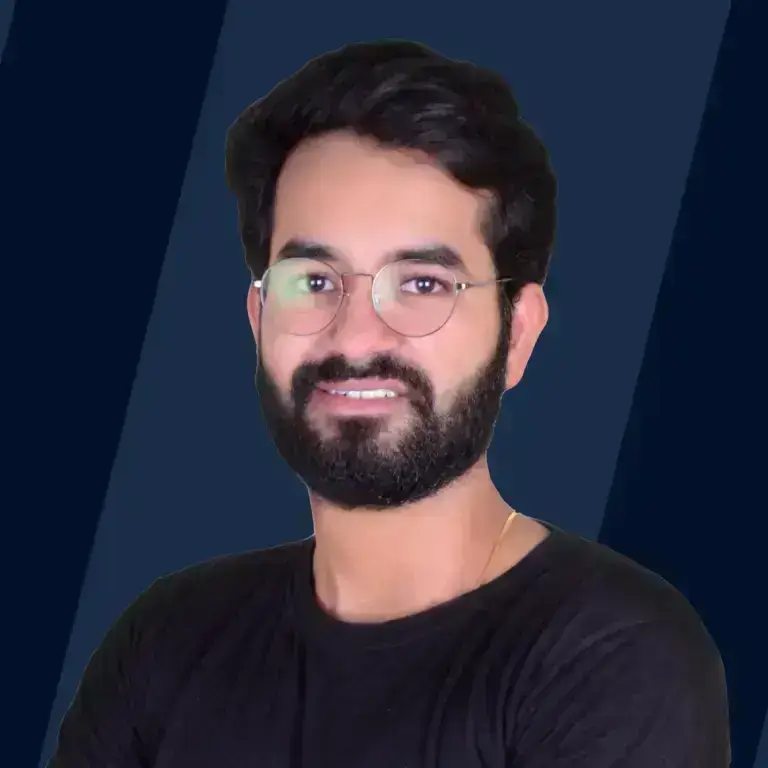
Overview
Have you ever tried implementing the most popular game - tic tac toe in Python programming language? We shall be creating an end-to-end tic tac-toe Python project and implementing the knowledge of Python that we have gained throughout the Python tutorial. Tic tac toe Python is a two-player game played on the 3X3 grid between two players. Each player chooses between X and O and the first player starts to draw the X on the space on the grid followed alternatively by the other until a player successfully marks a streak on the grid else if all spaces are filled the game is set to draw.
What We are building?
We will be implementing our Python knowledge learned so far from the scaler module link to Python module, to build, the project called the tic tac toe Python. Here we will be dividing the tic tac toe Python project into various steps to ease the understanding while parallelly building the game.
Tic tac toe Python, also known as Noughts and Crosses or Xs and Os, is a very simple two-player game where both the player get to choose any of the symbols between X and O. This game is played on a 3X3 grid board and one by one each player gets a chance to mark its respective symbol on the empty spaces of the grid. Once a player is successful in marking a strike of the same symbol either in the horizontal, vertical or diagonal way as shown in the picture below is created that player wins the game else the game goes on a draw if all the spots are filled.
Learn to Play tic tac toe Python
In the tic tac toe Python game that we shall be building, we require two players. These two players will be selecting their respective two signs which are generally used in the game that is, X and O. The two players draw the X and O on an alternative basis on the 3x3 grid having 9 empty boxes as shown below.
The game is played by drawing X and O in each box one by one for each player. The player who chose X starts the game. Once any player is successful in marking a strike of the same symbol either in the row-wise or column-wise or diagonally way as shown in the picture below is created that player wins the game else the game goes on a draw if all the spots are filled.
The players play the game as follows:
In total there are 8 ways to place the same sign to win the game. In the diagram below we can see all 8 arrangements.
When the 9 boxes get filled up without any winning arrangement then the game is said to be DRAW.
We can increase the complexity of the game by increasing the number of grids as well. We can create an automatic version of the game program as well which won't require any input from the user. This would not take away the fun involved with the game and building the game from scratch would be a lot of fun too.
While implementing the interesting tic tac tow Python project we can use NumPy, pygame, or random Python libraries. With the below module, we shall be dividing the tic tac toe Python project into multiple steps to keep a parallel track of our game implementation alongside learning the concepts behind what we are doing and how we are implementing the project. We are not going to build those heavy games but we are building the tic tac toe Python project on the CLI or the command line interface.
Pre-requisites
The major topics that must be refreshed before creating and building the tic tac toe Python project are as follows:
- Basic concepts of Python
- User define functions in Python
- Loops in Python (for loop, if else, if elif else)
- try-exception block in Python
- while loop of Python
How are we Going to Build the Tic Tac Toe Python Project?
The algorithm that we shall be following to build the tic tac toe Python project is as below:
- We shall start by designing the tic tac toe Python grid for which we shall be using the command line interface as implementing tic tac toe in Python using CLI comes in handy. We shall be using the user-defined function and print statements in Python.
- Then we shall be using the Data Structure to Store Data using the user-defined function in Python as it's very important to understand the dynamics of the game. For this, we shall be knowing about two parts that are, The Status of the Grid and The move of each player.
- After this we need to understand the game loop where we shall understand if the player has played their move without any exceptions.
- Then we all implement the information about the game from time to time to record what is the status of the game.
- It's very important to mark out the win or lose situations for which we should be creating a user-defined function in Python and will be implementing if statements in Python to check if after every move the game has been won or tied by any player.
- As this game is between two players our program needs to understand the same for we shall be using loops in Python to make this tic tac toe Python game give the player its chance alternatively.
- In this step we shall be capturing the names of the player as we want our tic tac toe Python project to showcase a proper scorecard at the end of the game to display the name of the winner alongside.
- After every move by the player we need to record the available chances the player holds to avoid any mismanagement by our tic tac toe Python project.
- As we want to showcase the Winner of the game at the end, we will be recording the points earned by each player and creating a scoreboard around it we need to create that in this step.
- We need our tic tac tor Python project to be flexible enough to allow the player to play as many matches as they want hence we shall be creating an outer loop of the game in this step.
- After each alternative chance as we want our code to handle and store the selection of the current player we shall be using the try-exception block in Python to handle any exception if encountered for the selection of the player inputs.
- The final step is to execute our entire program to help both the player play the game and record their victories too.
Final Output
The final project shall look like this where we can see the tic tac toe Python project after execution.
Output :
Requirements
The requirement for building the tic tac toe python are listed below:
- Good knowledge of Python Basics and refreshing the topics as mentioned under the subheading Pre-requisites.
- Command line Interface or any local Terminal to build the tic tac toe python.
- Text editor: We can use either VSCode Python 3 installed on your machine or we can implement the source code with any Python online compiler as well.
Building Tic Tac Toe using Python
Now after understanding the logical flow of the project and refreshing our Python knowledge based on the pre-requisite, let us start to create our tic tac toe Python project step by step.
Step 1: Designing the Tic Tac Toe Python Project:
As discussed in the algorithm before we can start playing the game as we are building our tic tac tor Python game from scratch that too using the command line interface. We need to start by designing a tic tac toe Python program 3X3 gameboard. For doing so, we shall be using the print statements from Python and designing the 9 empty boxes of the game board.
Our prime objective in creating the design of the tic tac toe Python grid function would be that whenever a player needs to mark the box of their choice, then he/she will be inputting the respective digit that is displayed on the grid. For example, if we want to select the first box of the grid on the leftmost corner then we will input 1 in the command line interface.
Code :
Output :
Explanation :
As seen above, we created a function called tictactoe_grid where we implemented the user-defined function knowledge from Python. This function takes values from the user as its parameter. We have used the print function along with the format function to act as the placeholder for the user's input value. Here the value parameter, signifies the status of each cell of the grid as a list.
Step 2: Using the Data Structure to Store Data
Now to understand the key principles of any game we need to dive deep into the dynamics of that game.
As we are building a relatively simple game, we need to know two important pieces of information that need to be stored so that they can be referred to at any point in time during the game which is as follow:
The Status of Grid: For this we will create a data structure that stores the status for each cell which can be either filled or empty.
The Move of each player: it is always good to store the past and present moves of each player which are the positions occupied by 'X' and 'O'.
Quick Note: We can access the above data from the Status of Grid which requires us to pass over the information every time we need to know the positions of each player. This is generally done by conserving time via the Time versus Space Complexity Trade-off.
Code :
Output :
Explanation :
As seen above, we created a user-defined function game_single for the tic-tac-toe Python game. This function is created for a single game of tic tac toe Python where the value signifies the parameter of the previous function. The variable position_player is used for storing the position of the box that is either filled by the cross (X) or the naught (O). We store three values that help to manage the Grid Status as a list of characters:
'X' - This signifies when a cell is occupied by the X Player. 'O' - This signifies when a cell is occupied by the O Player. ' ' - This signifies when a cell is empty or vacant.
To check the player move, we have created the variable position_player where the moves of the players are stored as a dictionary (consisting of a list of integers) where the keys are defined by 'X' and 'O' for each player.
Quick Note: The player_current variable is used to store the move of the current player as 'X' or 'O'.
Step 3: To Understand the Game-Loop
Understanding the game loop for the tic tac toe Python game allows the player to understand how to play the game until that player wins or a draw happens between the two players. Also, in the tic tac toe Python game, every single move of the player is denoted by the loop iteration.
Code :
Explanation :
To print the values for the function tictactoe_grid(), we have made use of the while loop from Python that helps us to generate the single tic tac toe Python game loop.
To handle the input from the player, we are using the Try-Exception block for 'CHANCE' input that can handle the unintended value if provided by the payer as his move during each iteration of the game. We also handled the ValueError exception to resolve if the same error gets encountered and also to maintain the continuity of the game. Finally, we also performed a few sanity checks, to verify if the value given by the player is a valid input or not or if the valid position given is already occupied or not.
Step 4: Updating the Game Information
Now that we have understood the game loop and gathered the inputs from the player, we need to update the information too. We can make our tic tac toe Python game work smoothly by updating the information by concatenating the below-given piece of code into our main game program.
Code :
Output :
Explanation :
Once we call our tictactoe_grid() function, the grid will be as shown above as we now have the value list updated. We updated the status of the grid in addition to the position of the player by updating our game information where we update the boxes that got occupied as per the players which are reflected by the value list. Also, by adding the position just filed by the move of the current player we reflect the position of the player.
Step 5: To Validate the Win or Tie Situation
Now we move towards analyzing the win or tie situation. For that, we need to validate if any player after every move it takes has won the tic tac toe Python game or if the game ended as a tie.
We will be creating two user-defined functions in Python to validate the Win or Tie situation. We name these functions win_validate() and tie_validate().
win_validate(): This function validates if the player has satisfied any of the winning combinations that are stored. If it does, then it shall return TRUE; otherwise, it shall give output as FALSE.
tie_validate(): This is a function that validates if all 'Nine' boxes on the grid are filled and the game is ending with a tie.
Code :
Explanation :
As seen above, we created user-defined function (UDF) in Python to define the win_validate() and tie_validate(). We made use of the for loops in Python and if statements to validate if the winning combination to win the game is met or not. Consecutively, while calling both the functions we shall get the respective output if the game is won by any player or it has resulted in a tie. The game_single() function shall return if the current player has won the game or not. Else, the game will return 'D' signifying a tie.
Step 6: Switching Between the Player Once the Chance of One Player is Executed
As the tic tac toe Python game is based on an alternate chance being given to each player after one player has played its chance therefore we shall be using the if-else loop in Python now to enable this switching between the players after its chance has been executed.
Code :
Explanation :
We implemented the if-else statement in Python to switch between the player once the chance of one player is executed in a way that when the current player makes its move and takes one position from the grid, then the program must switch the position to the next payer.
Step 7: Recording the Player's Names to Observe on the Scoreboard
As we are maintaining the scoreboard to see what is the score against each player's name after they have played multiple matches of the tic tac toe Python game, we will be using the main function in Python to capture and record the Player's names to observe on the scoreboard at the end of the game.
Code :
Explanation :
Above shows the special variable__name__ that has the value of "main". We use the input function in Python to provide the names of the two players. This will be captured as soon as the game starts when the entire program for the tic tac toe Python game gets executed.
Step 8: To Capture the Game Information
In the tic tac toe Python game, it's always important to store the game information so that we are capturing the data related to the current player, the selection between the X or O that the players choose, the available options in the game which is either X or O and the scoreboard information.
Code :
Explanation :
As per the above code, we selected the current player as the first player along with storing the information on the selections made by both players as a dictionary, the available options in the game as a list, and the scoreboard information as a dictionary.
Step 9: To Design the Scoreboard
We shall be designing the Scoreboard as a dictionary data structure to keep a track of multiple games that the players play. here, the players' names will act as the keys of the dictionary, and their respective wins shall be captured as the values.
Code :
Output :
Explanation :
We used the user-defined function my_scoreboard(score_board) which is taking score_board as the parameter where we define the variable that captures the names of the players in a list using the .keys() function in Python after which we index that as part of our score_board and show the respective scores which here is 0 as this is what shall be visible while the game starts.
Step 10: Outer Loop to Make our Game Flexible and Allow Multiple Games of Tic Tac Toe Python to be Played
As we are our game flexible enough to let the players play multiple games of tic tac toe Python, we shall require another loop for the same where we shall allow the current player to select the mark for each match. The selection menu will also be shown in each iteration of the game.
Code :
Output :
Explanation :
As seen above we implemented the while loop from Python to show the menu and give the current payer which is John here to make the choice for the series of the Tic tac toe Python game. John can now make use of action between the three options Cross 'X' or Naught 'O' or exit the game by pressing the respective key.
Step 11: To Handle Exception and Allot the Selection of the Symbol for the Current Player for Each Iteration of the Game
It's very important to maintain the flow of the game to Handle Exceptions and Allot the selection of the symbol for the current player for each iteration of the game, for which we shall be using the try-exception block in Python as shown in the below code. The selection made by the current player will be captured and evaluated to let them know which player has won the game.
Code :
Explanation :
In the above snippet of code, we have used the try-exception block to handle any exception for the the_choice input. We have then used the if-else statement to create the choice menu for the current player to select from.
As per the selection made by the player, the data will be stored. This is significant as it will tell us which player won after every match.
Step 12: Execution of the Tic Tac Toe Python Game and Updating the Scoreboard Simultaneously
Now we are approaching the final part of our tic tac toe Python game, where we shall be executing an independent game between the two players and capture the win for the player. And as we have our scoreboard ready we shall also be updating it when the first game end as well.
Code :
Explanation :
As seen above, we captured the details of the winner of the tic tac toe Python game for a single match. we also used the if statement in Python to validate if the match is getting a tie or if one of the players is winning the game. If the game is a tie, then again the new match will start else when the game ends in a win the score of the player gets updated and the scoreboard gets updated too.
Step 13: Swapping the Chance Given to Choose the Symbol Between the Selecting Player
It is important to swap the chance given to choose the symbol between the players after each game has ended. While playing a game, it becomes mandatory to switch the chance of choosing the mark. For doing so, we shall be using the if-else statements in Python for letting the players switch the chance of selecting the mark (X or O) once one game has ended so that both the players get an equal chance to choose between the symbol.
Code :
Explanation :
Using the if-else statement in Python we created the possibility of giving each player an equal chance to choose between the Cross or Naught after each game has ended.
Step 14: It's Gameplay Time
Now as we have understood each step in detail and the logic behind each let us compile all the above-created functions and execute our tic tac toe Python game to see it working and also to enjoy our very own created game!!
Code :
Output :
Please refer to the subsection - Final Output under the heading What we are building?
Elevate your coding skills with our Machine Coding Tic Tac Toe - LLD Case Study Course. Enroll now and master the art of low-level design!
Conclusion
- Tic tac toe Python, also known as Noughts and Crosses or Xs and Os, is a very simple two-player game where both the player choose between the symbol 'X' and 'O' to mark the position on a 3X3 grid. Once a player is successful in marking a strike of the same symbol either in the horizontal, vertical, or diagonal way that player wins the game else the game goes on a draw if all the spots are filled.
- We can access the above data from the Status of Grid which requires us to pass over the information every time we need to know the positions of each player. This is generally done by conserving time via the Time versus Space Complexity Trade-off.
- The player_current variable that we used was to store the move of the current player as 'X' or 'O' in the tic tac toe in Python.