time() in C++
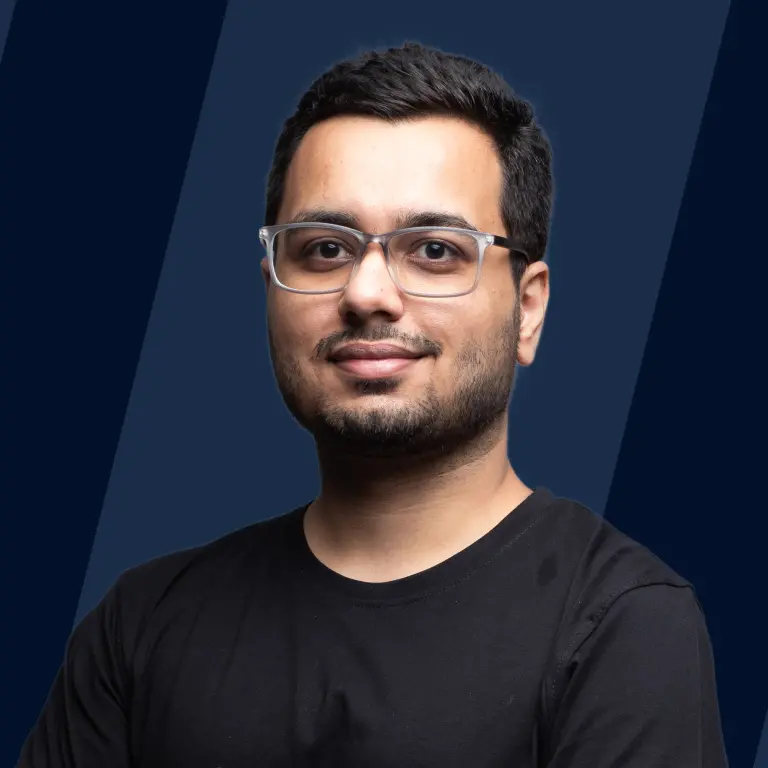
Overview
The time() function in C++ returns the current calendar time that has passed since 00:00 hours, January 1, 1970, UTC as an object of type time_t. The function accepts a single argument as a pointer to a time_t object and returns the time in seconds or -1 on failure.
What is time() in C++?
Ever wondered how we can find today's date or time using C++? C++ has a time() function that returns the current calendar time as an object of type time_t. time() function is defined in ctime header file.
The value returned from the function represents the number of seconds since 00:00 hours, January 1, 1970, UTC.
Syntax of time() in C++
The time function accepts one argument in its function call. The syntax of time() function is:
Here, the arg pointer is the function argument of type time_t.
Parameters of time() in C++
The time() function takes the following parameters as an argument:
- arg: It is a pointer to a time_t object which (if not nullptr) stores the time. Alternatively, if the value of this parameter is the NULL pointer, the parameter is not used in this case.
Return Value of time() in C++
The time() function returns either:
- On Success:: The current calendar time as a value of type time_t.
- On Failure:: -1 which is casted to type time_t.
Exceptions of time() in C++
The time() function guarantees no throw. Hence, it never throws an exception and always returns a value.
Example of time() Function
Let us see the time required by the C++ program to run a loop that required 108 iterations.
Output
Here, we calculate time once before using the sleep function and once after we sleep for 5 seconds. This way, the difference between the two times shows the compiler's time to execute the sleep function.
Example
1. Program to demonstrate time in C++
Let us see the time() function used in C++ to print the seconds passed since 00:00, January 1, 1970.
Output:
Here, we call the time() function to which we pass a NULL pointer as an argument. The function returns time in seconds that have passed since 00:00:00 GMT, Jan 1, 1970.
2. time in c++ with Reference Pointer
Let us see how we can use the argument in the time() function to store the returned time in a reference pointer.
Output:
Here, we create a variable of type time_t and pass its reference as a function argument to the time function. Therefore, the time value in seconds is stored in the present_time variable.
Conclusion
- The time() function returns the current calendar time as an object of type time_t. time() function is defined in ctime header file.
- The value returned from the function represents the number of seconds since 00:00 hours, January 1, 1970, UTC.
- The function accepts a single argument pointer (time_t* arg) to a time_t object which (if not nullptr) stores the time.
- The time() function returns either time in seconds or -1 on failure.
See Also
The header file <ctime> supports four time-related types: tm, clock_t, time_t, and size_t. Each of these types represents time and date as an integer. C++ ctime header has several other time-related functions that you can explore while working with date and time. Some of them are:
- localtime()
- asctime()
- clock()
- mktime()
- difftime()
Also, you can read more articles on some of the advanced concepts in C++ like: