Build a TODO App with JavaScript
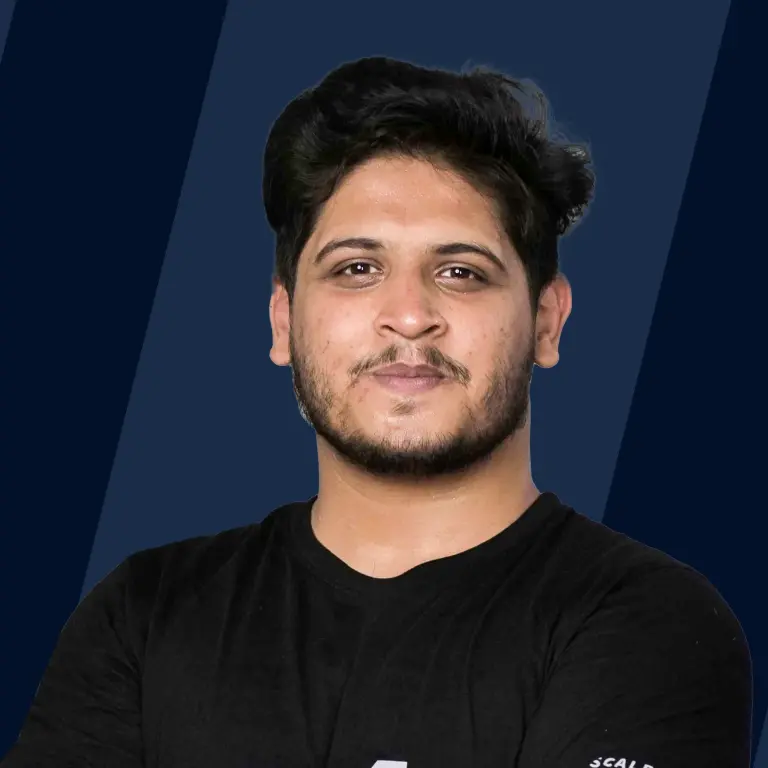
Overview
In this article, we will be building a TODO app using Javascript. The TODO app will be capable of doing the CRUD operations, Create, Read, Update and Delete. Let us dive into the article to get an understanding of CRUD operations and apply it in real to make a TODO list.
What are We Building?
We are going to build a TODO list application using Javascript. In the app, we will be able to perform four operations. We can create a new task, read, update and delete an existing task.
Pre-requisites
To build the TODO app shown in this article, you must have a basic knowledge of Javascript and its libraries. The topics that you should be aware of are Javascript variables, arrays, objects, and functions. If you do not have experience in building Javascript applications, do not worry. We will take you through the basics so that you understand every bit of the concept clearly.
How are We Going to Build the Todo App?
Here are a few steps we will execute before adding details to the project.
- We create three files to write the HTML, CSS, and Javascript code.
- Name the three files as index.html, style.css, and main.js.
- The next step is to link the style.css and main.js files to the index.html file.
HTML
Let us write the body of the code to make a TODO app using Javascript.
In the body element, we will create a div with the id myDIV and a class name header. Inside the div, we have three elements. We have a heading written as My To Do List Using Javascript. Below it, we have a textbox taking the tasks as input with a suitable id and placeholder.
We can also write some pre-defined tasks in the to-do list by creating an unordered list in HTML.
Now, we are all set for the body part. Let us customize our TODO list app and make it look attractive and user-friendly by adding some CSS.
CSS
Let us add beautifying elements to our application to make it look better. We will try to make it simple by adding some colors.
First, we will style the list items in the unordered list by adding properties like a cursor, padding, background color, font size, and a transition time of 0.2s to make the transition smooth. We can add a different color to the odd list items to make it even more attractive. Here is how you can do it.
You can make the app more user-friendly by changing the color of the list items when they are marked as checked or hovered.
Now, let us style the close button that helps us remove the unnecessary tasks in the list.
You can then style the header div by adding some padding, background, and text color.
The next step is to style the input box by adding padding and font size and specifying a width of 75%.
We have added a grey background to the add button, which changes color on hover.
After applying all these styles, we have made our TODO app more attractive and user-friendly.
JavaScript
Now let us use some Javascript to make our app work in the backend. First, we will create a close button and append it to all list items. It gets done by creating an array of variables denoting each list item. Then a span of text containing the close button is appended to each list item.
To remove the list item by clicking the close button, we hide it from the display area using the onclick function in Javascript. It gets implemented as :
Let us add a few more functionalities to our app by adding a checkbox to mark the completed tasks. We can strike through the completed tasks or remove them as per our choice.
Let us now learn how to add a new task using Javascript. We will also check if the user has entered something in the text box or not using the if-else loop. The added text gets inserted into the list with all the defined functionalities.
Let us see how it gets implemented using the Javascript code.
Final Output
Let us combine all the things we have learned before and write them in a single piece of code. We have implemented all the CRUD operations in the code. We have added a button that appends the text in the input box to the list. The list is readable to the user. We can also update it by checking it and marking it as completed. We have kept a close button to remove the task from the list.
Output
Conclusion
- In this article, we have learned to build a TODO app with Javascript.
- We have performed CRUD operations on the tasks in the list.
- The app allows you to create, read, edit/update tasks, and delete tasks.
- We used CSS to make our app look more attractive and user-friendly.
- We use Javascript in our code to make our application more responsive and working.
- The combination of HTML, CSS, and Javascript helps us to build a to-do app that performs all the operations.