tolower() in C
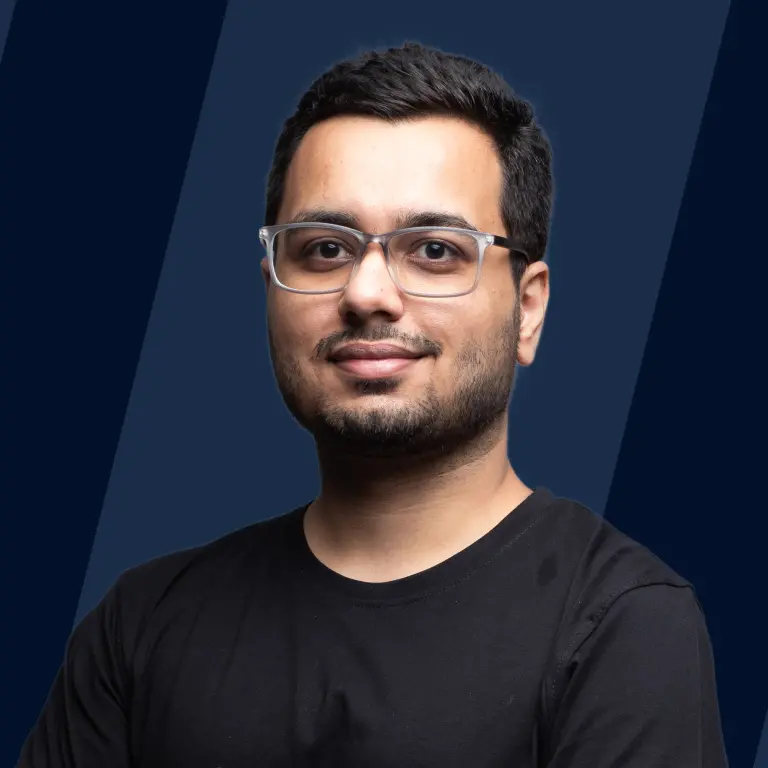
Overview
tolower() is a C library function that returns a given character argument to lowercase. If an uppercase alphabet is passed as an argument then the tolower() function returns a lowercase alphabet. For a lowercase alphabet or special symbol argument, the same value is returned. Also, we can pass only a single character at a time as an argument of the tolower() function. The tolower() function is defined in the ctype.h header file.
Syntax of tolower() in C
Syntax of tolower() in C is given below:
Parameters of tolower() in C
tolower in C takes only a single parameter of integer (int) data type. If we pass a character as an argument, the compiler will implicitly or internally caste the character to integer or will take its ASCII value as an argument. We can pass float and double data type variables as parameters to the tolower() function in C, but the compiler will internally caste them to int data type i.e, truncate the decimal part.
Return Value for tolower() in C
The value which is returned by tolower() in the C function is of integer(int) data type, which means this function returns the ASCII value of the final character. As we need this function to return char, return value int can be implicitly casted into char.
Exception for tolower() in C
This function doesn’t throw an exception but there are some cases where its behavior is undefined, like:
- For long long integers which are not in the range of integer data type, tolower() in C function returns the garbage value because its parameter is of integer data type.
- If the user tries to pass any object of user-defined data types like structure, enum, or union, this function will throw an error.
- We can pass only a single character as a parameter of tolower() function at a time, if we try to pass the character array or string as an argument then this function will give a runtime error.
Example of tolower() in C
Let's see a simple implementation of tolower function in C
Output:
In the above code, we simply created two characters and passed them as arguments in tolower() in the C function. Uppercase character ('A') got converted into lowercase while lowercase character ('a') remained the same. Let's see this change through the image for better understanding:
In the above image, we can see there is some change in ASCII value, we will look into that in detail in the next section.
What is tolower in C?
Let’s look into ASCII values first before moving forward: ASCII is a set of a total of 128 7-bit characters. Every character is given an ASCII number like digits from 0 to 9 have ASCII values between 48 to 57, uppercase letters in the range 65 to 90, and lowercase letters in the range 97 to 122. The difference between the ASCII value of each lowercase letter and the uppercase letter is 32.
If we pass a value in the range 65 to 90 that is the uppercase letter as an argument in function tolower in C, it will return the sum of the current value and 32 means its lowercase ASCII value, in another case, it will return the same value.
For float and double data type values it truncates the decimal part and if the integral part is in range 65 to 90 it will return the sum of an integral part and 32 otherwise only the integral part.
Let's understand the working of the tolower() function in C in detail through the image:
In the above image, we can see the changes in ASCII value or integral value by 32 when the passed parameter is in the range 65 to 90.
In the above image, we can see there are no changes in ASCII value or integral value when the passed parameter is not in the range 65 to 90.
Code to Understand ASCII and Return Value
Let's see some examples to get a better understanding of tolower() in C:
-
Uppercase alphabets
In this example, we will pass the uppercase alphabet as an argument and will look over its ASCII values:
Output:
In the above code, we simply passed the uppercase character as argument and in output got its lowercase character.
-
Lowercase alphabets
In this example, we will pass lowercase alphabates as argument and will look over it's ASCII values:
Output:
In the above code, we simply passed lowercase character as argument and in output got same lowercase character.
-
Special Characters/ Numbers
In this example, we will pass special characters, two different types of numbers, and a double value.
Output:
In the above code:
- For two special characters, the same character is returned.
- For number 70, the function returns a value 70 incremented by 32 For number 105, the same value is returned.
- When a double 70.456670 is passed as an argument, its decimal part was truncated and the function returned its integer part 70 incremented by 32.
Please note 70 lies between uppercase ASCII range(65-90), while 105 lies between lowercase ASCII range 97-122.
Conclusion
- tolower in C is used to convert uppercase letter to lowercase letter.
- It takes an integer data type as a parameter and its return type is also of integer data type.
- If we pass any character with an ASCII value between 65 to 90 or any number in this range, it will add 32 in it to convert it into a lowercase character.
- For characters with ASCII values other than 65 to 90 or numbers not in this range, the same value is returned.