toLowerCase() in Java
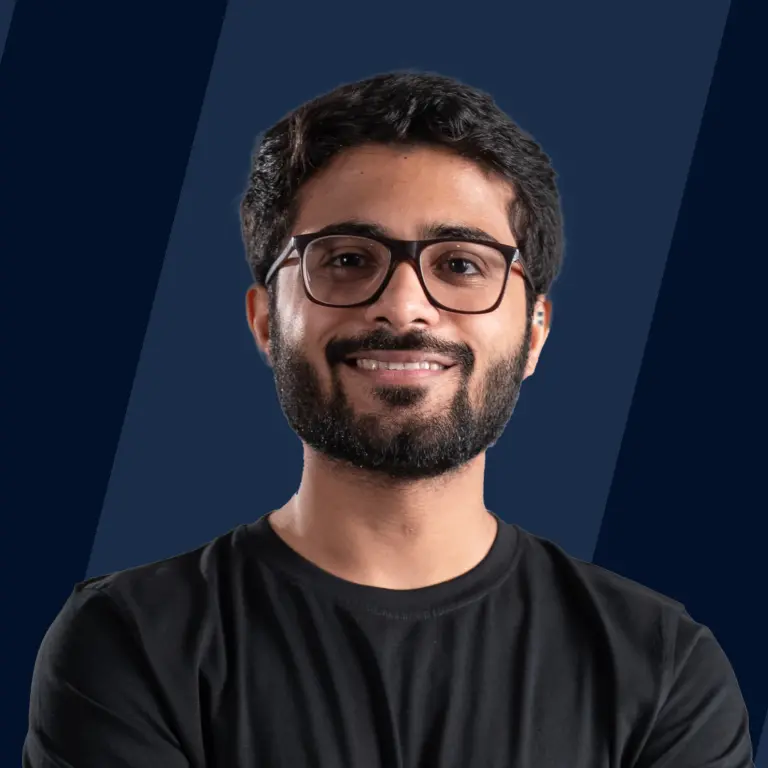
Overview
The toLowerCase() method in java is a String class method. It is used to convert all letters of a string into lowercase letters. This method is a String class method that is present in the java.util package. Here is the hierarchy of the toLowerCase() method in java.
There are two types of toLowerCase() methods in java :
- toLowerCase() :
Converts all characters into lowercase alphabets. - toLowerCase(Locale loc) :
Converts all the characters into lowercase according to the given Locale. The locale specifies the language we want the output in or any other rules.
Syntax of toLowerCase() in Java
Parameters of toLowerCase() in Java
The toLowerCase() method in java does not take any parameters. Here we don't pass any parameter to the toLowerCase() method in java. We call the method using the string object.
Return Value of toLowerCase() in Java
Return Type : String
The toLowerCase() method in java returns a String converted into lowercase. While returning the string to lowercase, all uppercase letters are swapped with lowercase and the previous lowercase characters are kept untouched.
Exceptions of toLowerCase() in Java
The toLowerCase() method in java does not throw any exceptions.
Examples
Let's understand how to convert a string into lowercase using the toLowerCase() method in java.
Example - 1 : Returns String in Lowercase Letter
Output :
In the above example, we have declared a string s1 with a sentence with both uppercase and lowercase letters. This string s1 is converted into lowercase letters using s1.toLowerCase() and stored in String s2. Finally, we have printed s2 which holds the converted lowercase string.
Example - 2 : Returns String in Lowercase Letter
Output :
In this example, we have created a string object s1 that holds the string to be converted. And converted the string into lowercase letters using s1.toLowerCase(); directly in the print statement.
Internal Working of toLowerCase() in Java
The class String includes methods for examining individual characters of the sequence, comparing strings, searching strings, extracting substrings, and creating a copy of a string with all characters translated to uppercase or lowercase. Case mapping is based on the Unicode Standard version specified by the Character class.
The internal implementation of the toLowerCase() method of the String class in Java is as follows :
The above code takes a Locale object and returns a String in lowercase form. It first checks if the Locale object is null and throws a NullPointerException if it is. The method then iterates over each character in the String and checks if it needs to be changed to lowercase according to the rules of the specified Locale object.
If there are any characters that need to be changed, the method creates a new character array with the lowercase characters and returns a new String. If no characters need to be changed, the method simply returns the original String. The method takes into account special cases where certain characters need to be mapped to multiple lowercase characters, such as the Turkish dotless i or the Greek capital letter sigma. It also handles characters outside the Basic Multilingual Plane by growing the result array if needed.
toLowerCase() with Locale Parameter
The toLowerCase() with Locale Parameter converts all the characters of the string into lowercase characters using the given Locale such as languages, country code, etc.
Locale : A Locale object represents a specific geographical, political, or cultural region. An operation that requires a Locale to perform its task is called locale-sensitive and uses the Locale to tailor information for the user.
Syntax :
Parameters :
The toLowerCase() with Locale method in java takes Locale as a parameter. This Locale sets rules for the output string.
Returns :
The toLowerCase() with Locale method in java returns String converted into lowercase as per the given Locale.
Example :
Now let's see an example to convert string letters into lowercase using the toLowerCase() method with Locale. Here, we will try to print output in a different language like 'Turkish' using the Locale parameter.
Output :
This article covers toLowerCase() method in Java. Two types of the toLowerCase() method are covered in this article, without parameters, and with locale parameters.
In this example, we have used the toLowerCase method in Java along with Locales. Here we have created locales for two languages Turkish and English using Locale.forLanguageTag("tr"); and converted it into lower case using s1.toLowerCase(tur);. The final output is displayed in the output section.
Conclusion
- The toLowerCase() is a method of String class located in the java.util package in Java.
- It converts all letters of String into lowercase and returns it in the form of String.
- The toLowerCase() method neither takes any argument nor throws any exception.
- We can convert a string into the required language using the toLowerCase(Locale L) method. It takes Locale as a parameter and returns a string with lowercase letters as per rules defined by the locale.