toupper() in C++
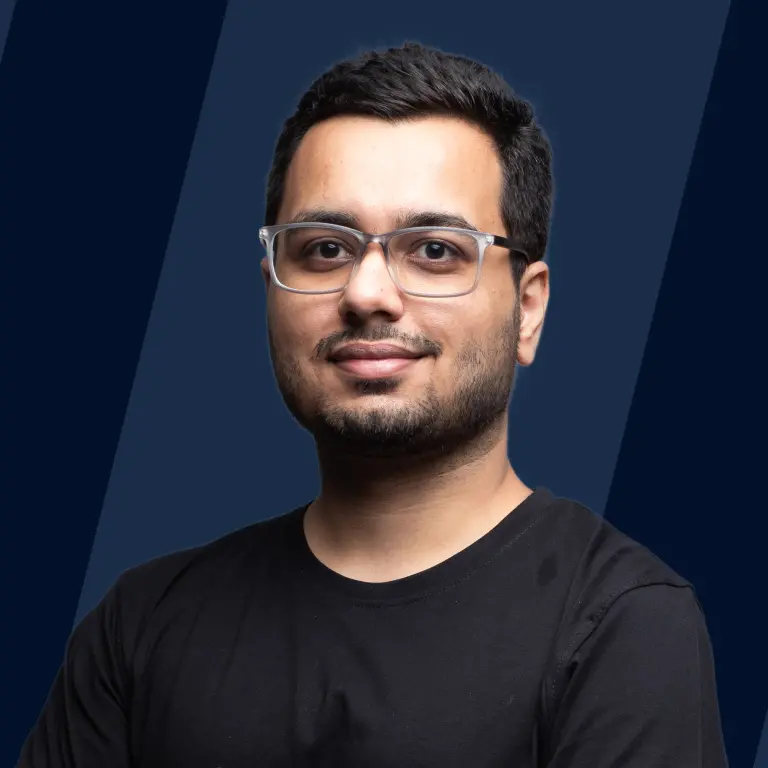
Overview
The toupper() function in C++ is included in the ctype.h header file and converts the lower character passed as the parameter into uppercase. If an uppercase character or special characters other than alphabets are passed as parameters to the function, then the function returns the same value.
Syntax of C++ toupper()
The syntax for toupper c++ function is as follows
Parameters of toupper() in C++
The toupper function in cpp takes one parameter. The character that has to be converted into uppercase is passed as the parameter to the toupper c++ function.
From the syntax, we can observe that the parameter passed is of int datatype, but we are dealing with the characters.
This is because when passing a character, the compiler implicitly or internally converts it into the ASCII value of the character, represented in the form of int.
Return Value of toupper() C++
The toupper c++ function converts or typecasts the lowercase character passed to an uppercase character. The function returns the ASCII value of the Uppercase character of the parameter. Hence it has an int return type.
The computer can understand only the language of binary. When a char data type is declared in C or C++, it is stored as an integer value according to ASCII.
Examples
Let us look at a simple implementation of toupper c++ function in a program.
C++ toupper() without Type Conversion
Code
Output
Explanation
In the above example, we have passed the characters a, b, y, and z as parameters to the toupper c++ function. The toupper function implicitly converts the character to an integer type and converts it to its uppercase, and returns the ASCII value of the uppercase character.
toupper() in C++ with Type Conversion
Code
Output
Explanation
This example is similar to the previous example. In this example, we have used the type conversion to convert the ASCII value to the character datatype.
toupper() in C++ with String
Code
Output
Explanation
In the above example, we have a string with special, uppercase, and lowercase characters. Using a while loop, we iterate through each character in the string and pass it as a parameter to the toupper function in C++.
The lowercase characters are converted into uppercase characters as their ASCII ranges from 97 to 122. The uppercase characters and special characters remain the same as their ASCII value is not n the range of 97 to 122.
Undefined Behaviour of toupper() in C++
The toupper function has no exceptions, but in some cases, the function's behavior is not defined.
- If the parameter passed to the toupper function is not a primitive data type.
- If the parameter passed after typecasting isn't in the integer range.
How does C++ toupper() work?
All the characters have a unique ASCII value associated with them. The lowercase characters will have ASCII values running from 97 to 122. The upper case letters range from 65 to 90.
When a character is passed as a parameter to the toupper c++ function, the character passed as a parameter is converted into its respective ASCII code by the toupper function. If the double and float value after typecasting to integer or any integer value lies in the range of 97 to 122, then 32 is subtracted from the value. If the value of the integer is outside the range of 97 to 122, then the parameter passed is returned without any changes.
Conclusion
- The toupper function in c++ is included in the ctype.h header file.
- The toupper() in c++ converts the lowercase character passed as its parameter into an integer type.
- If the passed parameter is not in the range of 97-122, then the toupper c++ function will return the parameter passed without any changes.
- If the parameter passed to the toupper function is not a primitive data type or after typecasting doesn’t lie in the integer range, then the toupper function will show undefined behavior.