Transient Keyword in Java
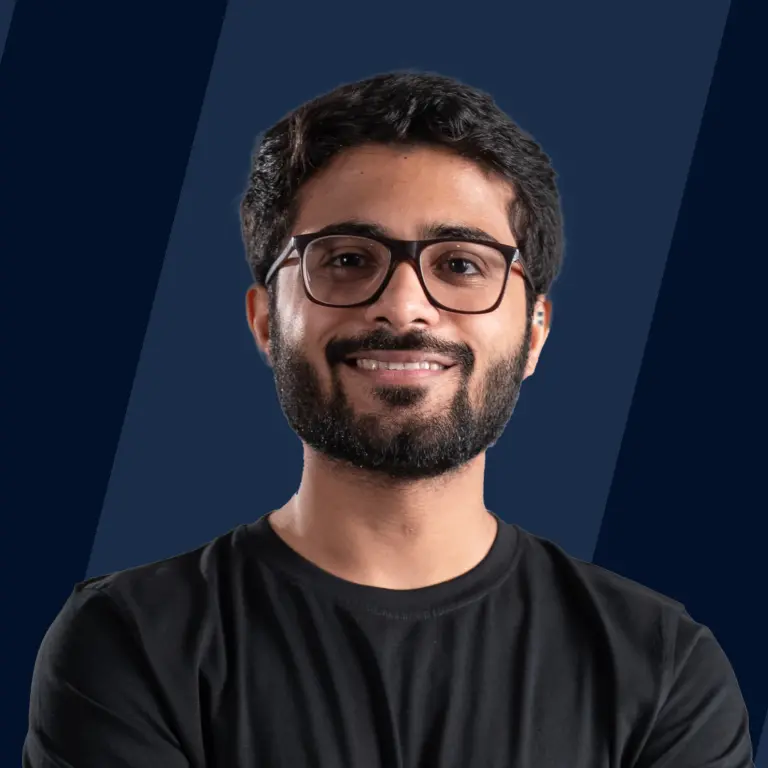
Overview
Serialization is the process of converting an object's state into a format that can be stored or transmitted, typically as a file or stream, in order to be reconstructed later. In Java, serialization is achieved using the Serializable interface and related classes.
The transient keyword is a variable modifier in Java used in the context of serialization. When applied to a variable, it instructs the Java Virtual Machine (JVM) to exclude that variable from the serialization process. Transient variables are not saved in the serialized form of the object.
Introduction to Transient Keyword in Java
Transient keyword is a variable modifier used in the context of Serialization in Java.
Serialization is a process of converting an object state into some file which can be saved in a hard disk drive rather than the main memory.
Let's take an example of an object where we have some confidential data in a variable.
During serialization, we do not want the confidential data to be saved on the hard disk. In such cases, the Transient keyword in Java is used along with the variable to prevent the serialization of that particular variable.
This informs the JVM that these transient marked variables need to be excluded while serializing the object.
How to Use Transient Keyword in Java?
The Transient Keyword in Java can be used by just mentioning the word transient while defining the variable. E.g.
Let’s see how we can use Java transient keyword with a program:
Below is a code snippet in which we have a Student class, with some member variables. Along with this we have two member functions, serialize() and deserialize() which serializes and deserializes the Student object respectively.
In this example the variable fullName is marked as transient because we can set the fullName as firstName + secondName once we deserialize the object, hence, no point of saving duplicate data in file.
Code Snippet 1:
Result:
On running this code, a new file named student.ser is generated in the project directory, which represents the serialized object.
We have used the Java transient keyword with the fullName variable. Therefore, when we deserialize the object and inspect the result, you can see that the fullName field is null.
Note: It is not mandatory to create a file with the extension .ser; you can use any extension for serialized files.
Usage of Transient Keyword in Java
Transient Keyword in Java are used with variables which need to be kept secured. Since serialization creates a persistent file, if the object contains passwords or security keys, everything will be saved in the disk, which is not a good sign of a secured system.
If we look at the serialized file(try to open the file as a txt file), we can clearly see the password in the .ser file.
File Content (content of file generated from code snippet 1 ):
The password variable is critical data and hence serialization needs to be avoided, hence we should use a transient keyword with that variable to skip the serialization of the variable.
Since we have used transient keyword with fullName and password, JVM will ignore these variables while saving the object as a serialized file.
How to Use Transient with Final Keyword?
Using transient with the final keyword does not affect serialization because variables declared as final are serialized directly by their values. Since a final variable can only be assigned once and cannot be reassigned, there is no need to exclude it from serialization.
If a field is both transient and final, it means that the field will not be serialized, but its value will remain the same even after deserialization. So, if a field is declared as final, its value will remain the same regardless of whether the object is serialized or not.
Difference Between Transient and Volatile Keyword in Java
The transient and volatile keywords have different purposes and are used in different contexts.
As we discussed earlier, the transient keyword is used in the context of serialization to determine whether a variable should be included in the serialization process or not. It allows us to exclude certain variables from serialization.
On the other hand, the volatile keyword is used in the context of modifying a variable in a multithreaded environment. It ensures that the variable is accessed and modified correctly by different threads, providing thread safety.
In summary, transient is used in serialization to control the inclusion of variables, while volatile is used to make variables thread-safe.
Explore Scaler Topics Java Tutorial and enhance your Java skills with Reading Tracks and Challenges.
Conclusion
- The transient keyword in Java serves as a variable modifier in the context of serialization, allowing developers to exclude specific variables from being saved during the serialization process.
- Serialization is the process of converting an object's state into a format that can be stored or transmitted, typically as a file or stream, and the Serializable interface is used for achieving serialization in Java.
- Transient variables marked with the transient keyword are not saved in the serialized form of the object, making it useful for excluding sensitive or redundant data from serialized files.
- The volatile keyword, on the other hand, ensures thread safety by providing memory visibility and preventing certain optimizations in multithreaded environments.
- It is important to understand the distinction between transient and volatile keywords, as they serve different purposes: transient for serialization exclusion and volatile for thread safety.