JavaScript String trim() Method
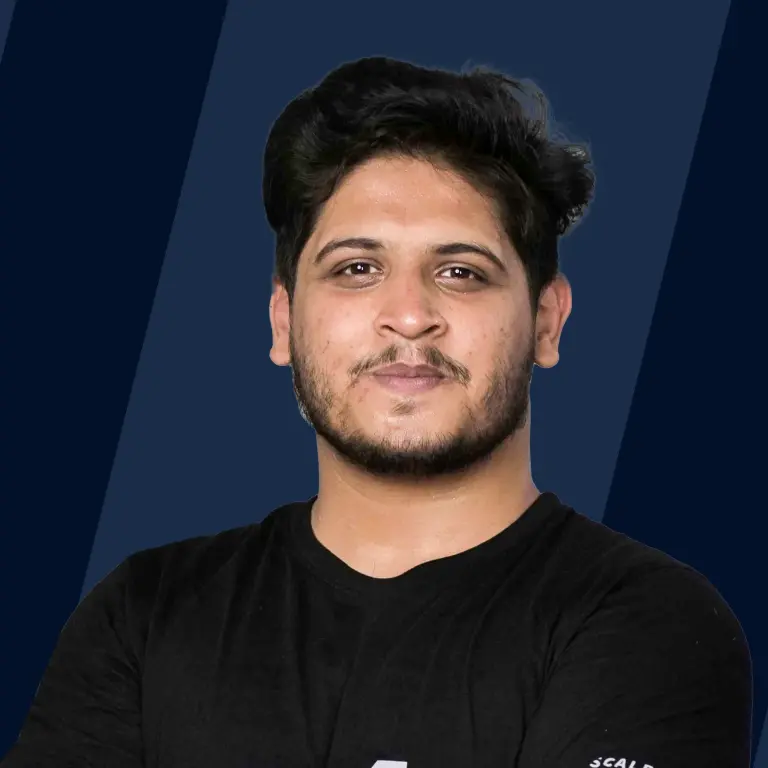
Overview
Trim in JavaScript is a built-in string function that is used to remove the white space characters from the start and the end of a particular string. It doesn't change the original string but returns a new string without leading and trailing white spaces. trim in JavaScript doesn't take in any parameter or functional arguments. If we want to remove the white-space characters only from the start or the end of a particular string, then we can use string.trimLeft() or string.trimRight() methods respectively.
Syntax of String trim() in JavaScript
Syntax:
Here, str is an object of the String class that denotes the value of the string to be trimmed.
Parameters of String trim() in JavaScript
trim() method in JavaScript doesn't have any functional parameters or arguments inside it. This method is invoked by the object of the String class.
Return Value of String trim() in JavaScript
There is a point to be noted that the trim() method in JavaScript doesn't change the original string but returns a new string after removing the white space characters from the original string from both ends. The white space characters can include all the spaces, tabs, no-break spaces, etc. If there is no white space character neither at the start nor at the end of the original string, then the trim in JavaScript will not return any exception but return a copy of the original string.
Here, the result is the value of the new string returned after trimming the original string str from both ends.
Short Example Code
In this JavaScript example code, we have initialized string str with a value having white space characters at both ends. Then, we are using trim in JavaScript that will remove white space characters from the original string str from both ends and returns a new string named result. We have then displayed the result in the console output window.
Output
As we can observe, white space characters are being removed from both ends after using the trim() method in JavaScript.
Value of str before trim: Learning trim in JavaScript
Value of str after trim: Learning trim in JavaScript
What is String trim() in JavaScript?
trim() in JavaScript is a built-in string function that is used to remove the white space characters from the start and the end of the given string. It doesn’t change the original string but rather, returns a new string without leading and trailing white spaces. The white space characters can include all the spaces, tabs, no-break spaces, etc. We know that trim() in JavaScript is a string method and therefore, it must be invoked by the instance or the object of the String class. Basically, we can create that instance or object of the String class upon which the trim() method will be implemented.
Have you ever wondered what if we want to trim a string from one end only i.e. either from the start or from the end of the given string?
Well in JavaScript, there are some additional methods similar to the trim() method which trim the string only from one end, either left or right. These methods are: trimLeft()and trimRight() and again, they don’t change the original string but return a new string after removing the white spaces.
Now, we will study these additional methods in detail along with their syntax and example codes.
trimLeft() or trimStart() in JavaScript
As the name suggests, the trimLeft() method in JavaScript is used to remove the leading white space characters from the given string i.e. white space characters from the start or the left of the given string. trimStart() is an alias name for trimLeft() method in JavaScript and both have the same functionalities. This method doesn’t affect the trailing white space characters of the given string.
Syntax:
Here, str is an object of the String class that denotes the value of the string to be trimmed from the left. The returned value (new string) will be stored in the ans variable.
Example 1
In this JavaScript example code, we have initialized string str with trailing as well as leading white space characters. Using the trimLeft() method, the leading white space characters will be removed from the string str but the trailing white space characters will remain as it is in the given string.
Output
As we can observe, we have displayed the result in the console window using document.write() method and it will still contain the trailing white space characters.
Example 2
In this JavaScript example code, we have initialized a string str with trailing as well as leading white space characters. This time we are using the trimStart() method by which the leading white space characters will be removed from the string str but the trailing white space characters will remain as it is in the given string. It has the same functionality as that of the trimLeft() method in JavaScript.
Output
As we can observe, we have displayed the result in the console window using the console.log() method and it will still contain the trailing white space characters.
trimRight() or trimEnd() in JavaScript
As the name suggests, the trimRight() method in JavaScript is used to remove the trailing white space characters from a particular string i.e. white space characters from the last or the right end of the given string. trimEnd() is an alias name for trimRight() method** in JavaScript and both have the same functionalities. This method doesn’t affect the leading white space characters of the given string.
Syntax:
Here, str is an object of the String class that denotes the value of the string to be trimmed from the right using the trimRight() method. The returned value (new string) will be stored in the ans variable.
Example 1
In this JavaScript example code, we have initialized a string str with trailing as well as leading white space characters. Using the trimRight() method, the trailing white space characters will be removed from the string str but the leading white space characters will remain as it is in the given string.
Output
As we can observe, we have displayed the result in the console window using the console.log() method and it will still contain the leading white space characters at the start of the string.
Example 2
In this JavaScript example code, we have initialized string str with trailing as well as leading white space characters. This time we are using the trimEnd() method which has the same functionality as that of the trimRight() method and with this, the trailing white space characters will be removed from the string str but the leading white space characters will remain as it is in the given string.
Output
As we can observe, we have displayed the result in the console window using the console.log() method and it will still contain the leading white space characters at the start of the string.
Some More Examples for trim() in JavaScript
To get clarity and for quick revision of the topic, let us discuss some more examples where we will implement trim() in JavaScript.
Example 1
In this JavaScript example code, firstly we have initialized the two strings: str1 with white space characters only at the end and str2 with white space characters at both ends. Then we implemented trim() and trimLeft() methods on str1 and str2 to get different results in the console output window.
Output
str1.trim() and str2.trim() will remove the white space characters from both the ends of strings str1 and str2 respectively while str2.trimLeft() will only remove the leading white space characters from the string str2.
Example 2
In this JavaScript example code, we are actually looking at a real practical life scenario where data scientists or data analysts work on some dataset for data processing and there is an inconsistency in the entries due to trailing and leading white space characters. This often results in errors and miscalculations. Here, we have two string arrays initialized already with some data entries inside them. Now, we are implementing trim() in JavaScript using the for-in loop to ensure that entries are without leading and trailing white space characters to maintain accuracy and consistency.
Output
As we can observe that there is an inconsistency in the dataset before trimming the white spaces from the entries. After trim() in JavaScript is implemented, all the entries are consistent now without any leading or trailing white space characters.
Example 3
In this JavaScript example code, we have implemented all the trim() methods: trim(), trimStart(), trimRight() using if-else statements in a function. Firstly, we have initialized a string str1 which contains the white space characters only at the beginning of the string. We have then invoked solve function to display the desired result. It consists of if-else conditions to determine the presence of white space characters in the string. If white space characters are present at both ends, then the function will implement the str1.trim() method. If white space characters are present only at the beginning of the string, then the function will implement the str1.trimStart() method else str1.trimRight() method will be implemented. Here in our case, it will implement and print the returned string of the str1.trimStart() method.
Output
As we can observe that the given string str1 has the white space characters only in the beginning and hence, according to the third if-else condition, it will display - "Leading white space characters only!!" along with the string after trimming.
Conclusion
- trim() in JavaScript is a built-in string function that is used to remove the white space characters from the start and the end of the given string.
- The syntax of trim() method in JavaScript: string.trim(); It doesn't take in any parameter and just returns a new string after removing the white space characters from both ends.
- If we want to remove the white space characters only from the start of the given string, then we can use string.trimLeft() method. trimStart() is an alias for trimLeft() method which has same the functionality.
- If we want to remove the white space characters only from the end of the given string, then we can use string.trimRight() method. trimEnd() is an alias for trimRight() method which has same the functionality.