C - TypeCasting
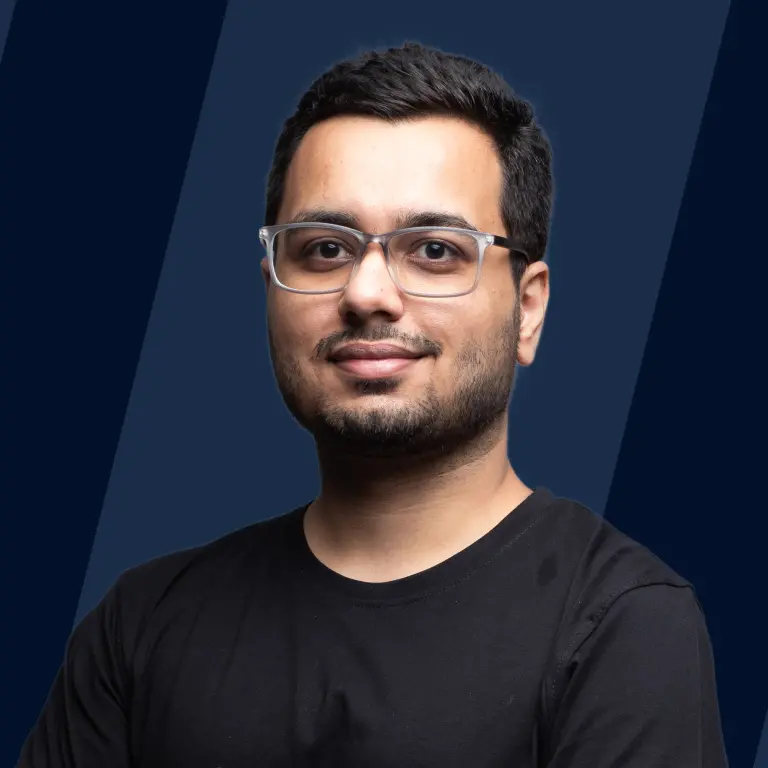
Overview
Type casting in C is a fundamental concept that allows to convert one data type into another. This process ensures that data is appropriately interpreted by the compiler, and it's a crucial tool for handling various data types efficiently. In C, type casting is achieved using keywords like int, float, char, and others, which indicate the desired data type for the value being converted.
Syntax
In C, type casting is the process of converting a value from one data type to another. The general syntax for type casting in C is as follows:
Here:
new_data_type represents the desired data type to which you want to cast the expression. expression is the value or variable that you want to cast.
For example:
Types of Type Casting in C:
1. Implicit Type Casting (Type Coercion): Implicit type casting in C, also known as type coercion, occurs automatically by the compiler when a value of one data type is assigned to a variable of another data type. This typically happens when a "smaller" data type is assigned to a "larger" data type, preserving data integrity.
For example:
2. Explicit Type Casting: Explicit type casting in C involves manually converting a value from one data type to another using the casting operator (new_data_type).
For example:
Explicit Type Casting
Let's take an example to demonstrate explicit type casting in c:
Program 1:
Output:
Explanation:
In this program, we explicitly cast the pi variable (which is a double) to an integer using (int) to remove the decimal part. The original value of pi is printed with %lf (long float) format specifier, and the rounded value is printed with %d (integer) format specifier.
Program 2:
Output:
Explanation:
Here, we explicitly cast the num variable (which is an int) to a char using (char). The original value of num is printed as an integer, and the character representation of the casted value is printed with %c.
5 Built-in Type Casting Functions in C Programming
C provides several built-in type casting functions to perform type casting in C. Here are five commonly used ones:
- atof(): Converts a string to a double.
- atoi(): Converts a string to an integer.
- itoa(): Converts an integer to a string.
- itoba(): Converts an integer to a binary string.
- atoi(): Converts a binary string to an integer. These functions are particularly useful when dealing with string-to-number or number-to-string conversions.
Advantages of Type Casting:
Data Integrity: Type casting allows you to ensure that data is properly converted when moving between different data types, preventing data loss or unexpected behavior.
Compatibility: It helps in making different data types compatible with each other, allowing operations and assignments that might otherwise be incompatible.
Flexibility: Type casting gives programmers flexibility in how they use and manipulate data, enabling them to adapt to various situations and requirements.
Precision Control: It allows you to control the precision of numeric values, such as truncating decimal places when converting from a float to an integer.
String Manipulation: Type casting functions (e.g., atoi() and itoa()) facilitate easy conversion between strings and numeric types, making string manipulation more straightforward.
Conclusion
- Type casting in C involves converting a value from one data type to another using the syntax (new_data_type) expression.
- Implicit Type Casting in C(Type Coercion) is automatically performed by the compiler when assigning values between compatible data types.
- Explicit Type Casting in C manually performed using the casting operator (new_data_type) to convert values between different data types.
- C offers built-in functions like atof(), atoi(), itoa(), itoba(), and atoi() for various type casting operations, facilitating string-to-number and number-to-string conversions.