What are the Types of Inheritance in C++?
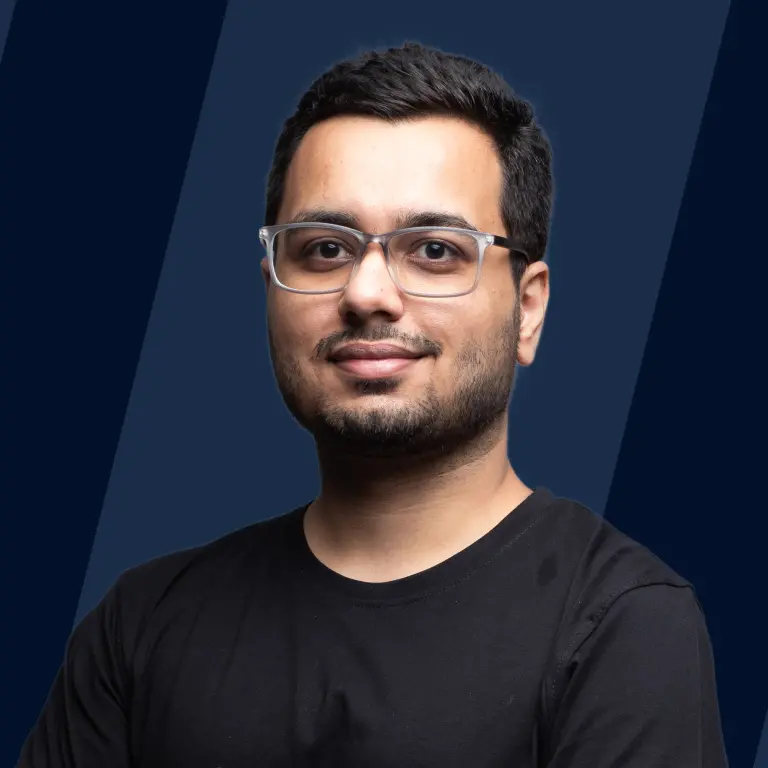
Inheritance is one of four pillars and the most significant feature of object-oriented programming in C++. As the name suggests, Inheritance is the ability of a class to inherit all the properties and characteristics of another class.
Introduction
You can understand the concept of inheritance by considering a real-life example: A child inherits properties and characteristics from their parents, like color, height, weight, and so on. Inheritance helps us reuse the code since the child class can reuse the parent members by inheriting them.
In addition, inheritance reduces code redundancy, as the child class inherits all the properties of the parent class, so there is no room for duplicate data to achieve the same task.
Depending on the way the child class is derived from the parent class or how many parent classes a child class inherits, we have the following types of inheritance in C++, namely:
- Single Inheritance
- Multiple Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
Single Inheritance in C++:
Single inheritance is one of the simplest inheritance among other types of inheritance in C++, in which the child class is derived only from the single parent class.
As you can see from the image above, class B is derived from a single-parent class A.
Let's see the syntax to perform single inheritance in C++:
Here, class A is the parent class, class B is the child class, and class B is inherited from class A.
Let's see the example of single inheritance:
The output of the above example is:
In the above example, the child class Dog inherits the parent class Animal in a public visibility mode. So, all the public and protected member functions and data members of the Animal class are directly accessible in the child class Dog.
Multiple Inheritance in C++
Multiple inheritances are another type of inheritance in c++ in which the child class inherits its properties from more than one base class. This means the child class is inherited from multiple parent classes, so the child class can derive the combined features of all these classes. The child class accesses all base class data members according to the visibility mode used.
As you can see from the image above, class C is derived from both the base classes A and B.
Let's see the syntax to perform multiple inheritances in cpp:
Here, class A and class B are the parent classes, and class C is the child class inherited from both parent classes A and B.
Let's see the example of multiple inheritances:
The output of the above example is:
In the above example, the child class Dog inherits the parent classes Animal and Speak in a public visibility mode. So, all the public and protected member functions and data members of the base classes are directly accessible in the child class. Also, when you create an object for a derived class, the constructors for the two base class, Animal and Speak, are called.
Multilevel Inheritance in C++:
Multilevel inheritance is one type of inheritance in C++ in which a derived class inherits all its properties from a class that itself inherits from another class, i.e., the parent class for one is a child class for the other.
As you can see from the image above, class C is derived from class B, which in turn is derived from class A.
Let's see the syntax to perform multilevel inheritance in cpp:
Here, class A is the parent class, class B is the child class, which is inherited from the parent class A, and class B is the parent class for class C, which is being inherited from class B.
Let's see the example of multilevel inheritance:
The output of the above example is:
Here, in the example, the object of the derived class Pug can directly access the members of the class Animal and Dog.
Hierarchical Inheritance in C++
In hierarchical inheritance, multiple child classes can inherit the property from a single-parent class.
As you can see from the image above, the child classes B, C, and D are derived from the single base class A.
Let's see the syntax to perform hierarchical inheritance in cpp:
Here, class A is the parent class, and classes B, C, and D are the child classes.
Let's see the example of hierarchical inheritance:
The output of the above example is:
Here, in the example, all the derived classes can access the base class Animal public members. When it creates objects of these three derived classes, it calls the base class's constructor for all three objects.
Hybrid Inheritance in C++
As the name hybrid suggests, it combines two or more types of inheritances. Due to the mixture of more types of inheritance, it is one of the most complexes.
As you can see from the above image, hybrid inheritance is the combination of hierarchical and multiple inheritances.
Let's see the syntax to perform hierarchical inheritance in cpp:
Here, class A is the parent class, classes B, and C are the child classes, and classes B and C are the parent class for class D, which inherits its properties from classes B and C.
Let's see the example of hybrid inheritance:
The output of the above example is:
Here, in the example, the constructors of all its superclasses are called when the object of the derived class Cute is created.
What are Child and Parent Classes?
You may have heard the terms like child class or derived class and parent class, or the base class, a lot of times in inheritance; let's understand these terms in detail:
-
Child Classes:
A child class is a class that inherits the properties from another class, also known as the derived class. The child class can access the data members and the member functions of the parent class according to the visibility mode specified during the declaration of the child class.
-
Parent Classes:
The parent class is the class from which the child is being derived and inherited; it is also known as the base class. Depending on the type of inheritance being applied, a single-parent class can derive multiple child classes, and multiple-parent classes can inherit a single-child class.
Here, class B is inherited from class A, then class B will behave as the child class, and class A will act like a parent or base class.
What is Inheritance?
Inheritance is one of the main pillars of Object-oriented programming in C++. It allows us to inherit the existing class (base class) properties to a new child class. This helps us reuse the same code again and extend or modify the attributes and behaviours defined in the base class.
Some advantages of inheritance are reusability, enhanced reliability, reduced code redundancy, and less development and maintenance costs as the existing code is used.
Learn more about inheritance
Why and When to Use Inheritance?
Inheritance is used because of the benefits it provides; the most important benefits of inheritance are discussed below:
-
Code Reusability:
One major advantage is code reusability; we can reuse the same code by inheriting the features and properties of one class into another.
-
Reduce Code Redundancy:
As we are not writing the same code again, code redundancy is reduced, leading to fewer development and maintenance costs.
-
Transitive Nature of Inheritance:
The transitive nature of inheritance means that if class B is derived from class A, class B will inherit all the properties of class A. Now, all the child classes of class B will also be able to inherit properties of class A because of the transitive nature of inheritance.
This will help in testing and debugging, as you can remove the bugs from the base class, and it will automatically remove bugs from all the child classes.
Inheritance is used when we want to inherit the properties of the base class in some other class; instead of writing the same piece of code again, we use inheritance to reuse the code. Also, while using inheritance, we should take care of things like both the class (child and parent class) should be in the same logical domain, the child classes should be of the proper subtype of the parent classes, and the parent class implementation is necessary for the child classes.
Visibility Modes
An important feature in inheritance is to know how the derived class will inherit the base class and which members of the base class will be acquired and accessible by the derived class. For this, we have three types of visibility modes (also known as access modifiers ) for all types of Inheritance in C++:
-
Public visibility mode:
In public visibility mode, when we inherit a child class from the parent class, then the public, private, and protected members of the base class remain public, private, and protected members, respectively, in the derived class. The public visibility mode retains the accessibility of all the parent class members.
The public members of the parent are accessible by the child's class and all other classes. The protected members of the base class are accessible only inside the derived class and its inherited classes. However, the private members are not accessible to the child class.
The syntax of using visibility modes is as follows:
The visibility_mode can be public, private, or protected.
Let's see an example to understand the concept of public visibility mode:
The protected variable x2 will be inherited from the Parent class and accessible in the Child class. Similarly, the public variable x3 will be inherited from the Parent class and accessible in the Child class, but the private variable x1 will not be accessible in the Child class.
-
Private Visibility Mode:
In the private visibility mode, when we inherit a child class from the parent class, all the base class members (public, private and protected members) will become private in the derived class.
The access of these members outside the derived class is restricted, and the member functions of the derived class can only access them.
Let's see an example to understand the concept of private visibility mode:
As the visibility mode is private, so, all the members of the base class have become private in the derived class. The error is thrown when the object of the derived class tries to access these members outside the derived class.
-
Protected Visibility Mode:
In the protected visibility mode, when we inherit a child class from the parent class, all the base class members will become the derived class's protected members.
Because of this, these members are now only accessible by the derived class and its member functions. These members can also be inherited and accessible to the inherited subclasses of this derived class.
Let's see an example to understand the concept of protected visibility mode:
As the visibility mode is protected, the protected and the public members of the Parent class become the protected members of the Child class.
The protected variable x2 will be inherited from the Parent class and accessible in the Child class. Similarly, the public variable x3 will be inherited from the parent class as the protected member and will be accessible in the Child class, but the private variable x1 will not be accessible in the Child class.
Given table will summarize the above three modes of inheritance in C++:
NOTE: If no visibility mode is specified, then, by default, the private mode is considered.
Diamond Problem
The diamond problem is a problem or an ambiguity that arises because of multiple inheritances where there are a lot of chances of having multiple properties or methods with the same name in different sub-classes, which may lead to ambiguity.
The diamond problem occurs when two classes B and C inherit from class A, and then class D inherits from both B and C, so if there is a method defined in class A that class B and C have overridden, then class D faces the ambiguity of which version of the method does class D inherit: that of B, or that of C?
This problem is known as the diamond problem because of the shape of the class inheritance diagram in this situation, as you can see from the image above.
The following example below will help to illustrate the ambiguous situation caused because of multiple inheritances:
In the above example, the error will be thrown, stating that var1 is ambiguous and the ambiguity is being caused. This happens because there are two copies of the data member var1 of the base class A, one for class B and one for class C. When the object obj of the child class D tries to access this member variable, it is not specified which copy of the variable var1 is to be used. Hence the ambiguity caused by an error is thrown.
There are two ways to deal with the diamond problem and to remove the ambiguity:
- Using the Scope Resolution Operator:
Let's consider the same example above and see how the scope resolution operator can help deal with the diamond problem.
The output of the above program will be:
The ambiguity error will be removed as the scope resolution operator specifies which copy of var1 will be used.
Here, the A’s version of var1 is accessed.
- Using Virtual Keyword:
With the help of the scope resolution operator, we were able to remove the error of ambiguity, but still, there are two copies of the parent class. If you require only one copy of the base class, there is another solution using the virtual keyword.
The virtual keyword allows only one copy of the parent class to be created, and then we can call the object of the child class in a way we normally do, and using that object, we can access the data members of the parent class in the usual way.
The example below will show the use of the virtual keyword to remove ambiguity in the diamond problem.
The output of the above program will be:
In the above example, classes B and C inherit the base class A as virtual, so it will only create a single copy of the base class A. So, now the derived class D object has only one copy of the base class data members. Hence only one copy of the variable var1 will be created, which makes it error-free and unambiguous.
Ready to make C++ your coding superpower? Enroll in our C++ course and gain the skills to create efficient, high-quality programs.
Conclusion
- Inheritance is one of the main features of object-oriented programming in CPP. It allows us to inherit another class's properties.
- There are five main types of inheritance in C++: single inheritance, multiple inheritance, multilevel inheritance, hybrid inheritance, and hierarchical inheritance.
- Visibility modes are used to know how the derived class will inherit the base class
- In public visibility mode, all base class members remain the same name as the derived class.
- In private visibility mode, all the base class members will become private in the derived class.
- In protected visibility mode, all the base class members will become the derived class's protected members.
- The diamond problem is an ambiguity arising from multiple inheritances.
- We can use the virtual keyword to overcome the ambiguity and conflict in the diamond problem. This will help us to differentiate the functions with the same name that came to the last derived class in the diamond problem .with the same name that came to last derived class in diamond problem.