Types of Queue in Data Structure
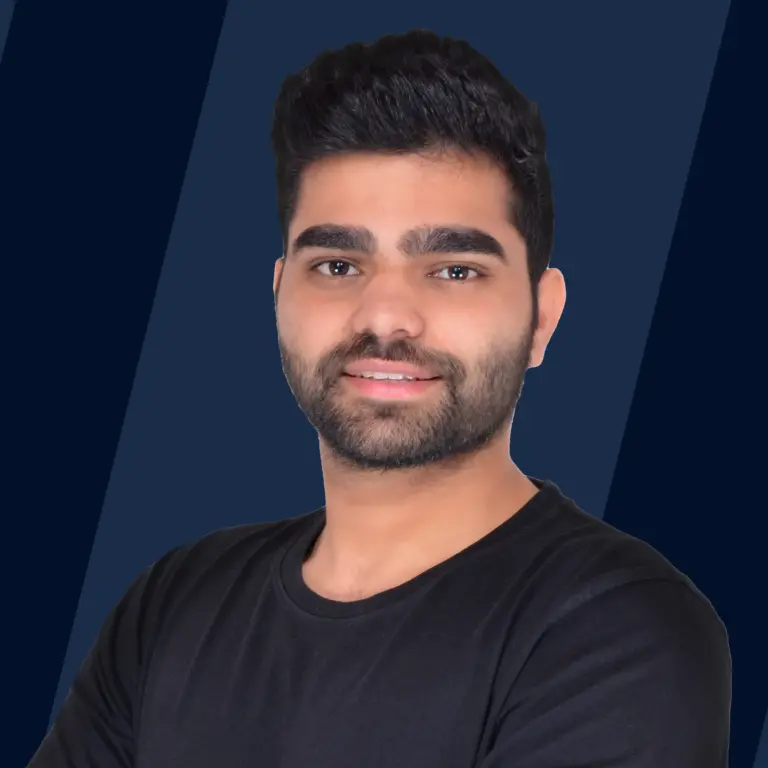
In the real world, a queue is defined as a row of elements in which one element enters from one side and exits from the other. Similarly, in data structures, the queue exhibits the same property, i.e., we can add an element from the rear end, which is also known as the tail of the queue, and remove an element from the front end, which is also known as the head of the queue. This queue characteristic is FIFO (First In, First Out). In this article, we will discuss types of queue in data structure in depth.
There are four types of queues:
- Simple Queue
- Circular Queue
- Priority Queue
- Double-ended Queue
Simple Queue
In a simple queue, we follow the First In, First Out (FIFO) rule. Insertion takes place at one end, i.e., the rear end or the tail of the queue, and deletion takes place at the other end, i.e., the front end or the head of the queue. It is employed in scenarios where data consumption should follow FIFO order, but recent data insertion may need to be removed due to factors like irrelevant data or performance issues. It is also called the Input restricted queue.
It prevents queue overflow and overloading by restricting the number of items added, and it helps maintain system stability and predictable performance.
The time complexity of a simple queue is O(1) for insertion and deletion operations.
Circular Queue
A Circular Queue, also known as a Ring Buffer, is a type of linear data structure that adheres to the FIFO (First In, First Out) principle. Unlike a traditional queue, the last position in a Circular Queue is linked back to the first position, forming a circular arrangement.
Key points about Circular Queue:
- It operates on the FIFO principle, where the rear is connected to the end, creating a circular structure.
- Commonly referred to as a ring buffer due to its circular nature.
- Insertion and deletion operations in a Circular Queue have a constant time complexity of O(1), making it efficient for managing data.
- Circular Queues find application in various domains such as memory management, traffic systems, and CPU scheduling due to their efficient operation and ability to manage data in a cyclical manner.
Note: To learn more about the circular queues, go to Circular Queue in Data Structure.
Priority Queue
A priority queue also exhibits similar characteristics to a simple queue where each element is assigned a particular priority value, where elements in the queue are assigned based on priority.
-
There are two types of priority queues:
- Ascending Order: In this priority queue, the elements are arranged in ascending Order of their priority, i.e., the element with the smallest priority comes at the start, and the element with the greatest priority comes at the end.
- Descending Order: In this priority queue, the elements are arranged in descending Order of their priority, i.e., the element with the greatest priority is at the start, and the element with the smallest priority is present at the end of the queue.
For insertion and deletion, the priority queue has a time complexity of O(logn). More information can be found at Priority Queue in Data Structure.
Double Ended Queue
A Double-ended Queue, or Deque, is a different type of queue where enqueue (insertion) and dequeue (deletion) operations are performed at both ends, i.e., the rear-end (tail) and the front-end (head). The Deque data structure supports clockwise and anticlockwise rotations in O(1) time, which can be useful in certain applications.
The Deque can further be divided into two special queues, i.e., Input-restricted Deque and Output-Restricted Deque.
The time complexity of a double-ended queue is O(1) for insertion and deletion.
Applications of Queue
Different real-world problems are solved using queues very efficiently. Here some of the major applications are listed below-
- Linear queues manage data sequentially, with additions at one end and removals from the other, commonly seen in printer or message queues.
- Circular queues, akin to linear queues but with a circular connection, optimize memory use and enhance performance, which is ideal for CPU scheduling and management.
- Priority queues prioritize tasks based on assigned levels, ensuring high-priority items are handled first, used in OS task scheduling and network packet prioritization.
- Double-ended queues, or deques, allow flexibility with additions or removals from either end, offering versatile data processing for applications like job scheduling and search algorithms.
- Concurrent queues are designed for simultaneous access by multiple threads, ensuring thread-safe data sharing, such as database transactions and web server requests, in multi-threaded environments.
- Queues find utility in scenarios where the processing doesn't demand immediate attention but follows a First In, First Out order, such as in Breadth First Search algorithms.
- They are instrumental in situations involving shared resources among multiple consumers, like CPU or disk scheduling.
- Asynchronous data transfer between processes benefits from queues, facilitating orderly management in IO buffers, pipes, or file IO.
Conclusion
- Queues adhere to the FIFO (First-In-First-Out) rule, meaning the first element added is the first to be removed.
- There are four main types of queues: Simple Queue, Circular Queue, Priority Queue, and Double-ended Queue.
- Priority queues can be categorized into Ascending and Descending based on the priority order of elements.
- Operations in a queue typically involve adding elements to the back end and deleting elements from the front end.