Java Variables
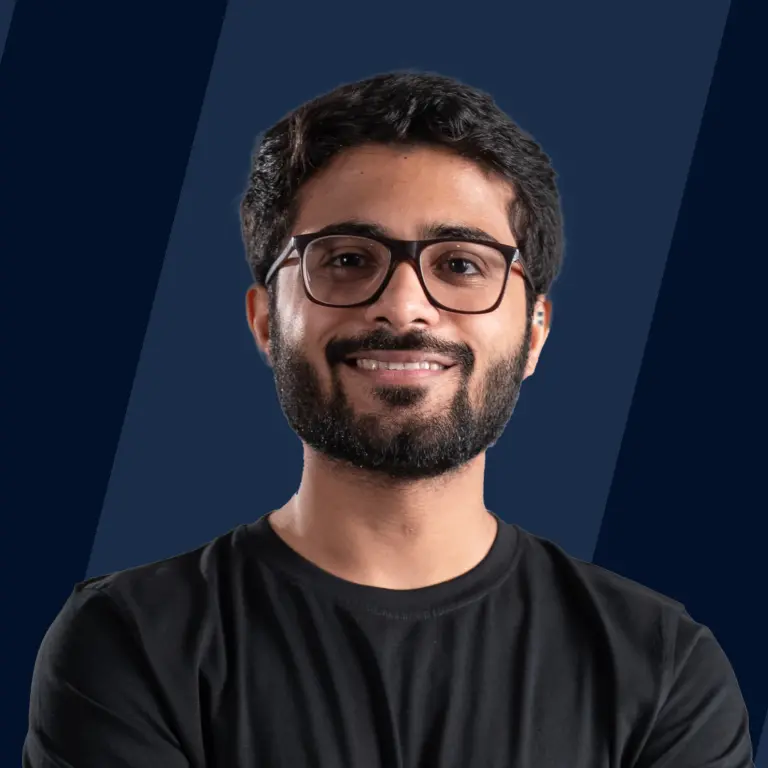
Overview
In Java, variables can be thought of as a container that has a name and a type. The name helps us identify each variable and the type tells us the amount of memory required by it and additional information required to be stored for the variable. These variables can be of various types such as local, instance, and static.
Declaring (Creating) Variables
Each variable in Java has a data type. These types of variables in Java are used to determine the size and layout of that variable. It can also be used to verify the range of the values it can store and the set of operations that can be performed with the variable.
To declare a variable in Java, we need its data type. Data type defines the type of data to be stored in a variable, it can be int, string, float, boolean, etc. Let us see a common structure to declare and initialize a variable along with an integer declaration.
Example
We can declare a variable without a value as well. In this case, the variable must be initialized before using it in the program else the program may crash.
Points to Remember While Using Variables:
- All the variables must be initialized before using them anywhere in the program. The best way is to initialize variables while declaring them at least with some default value.
- Variables are only accessible within their block of code or scope. Avoid accessing variables outside of the scope.
- The type of the variable and its initialization value must be compatible. The string value cannot be assigned to an integer variable.
- Naming of the variables must be thoughtful and should denote their purpose. Do not use names such as a, b, n, etc.
- For the constants that are not to be modified throughout the program use the final keyword.
Types of Variables in Java
Types of variables in Java are divided into three categories, local, instance, and static.
Let us take an example and understand each.
Local Variable
Local variables in types of variables in Java, as the name suggests are locally declared within some scope. These are usually declared inside methods, constructors, blocks of codes, loops, etc.
These can only be accessed and are visible within the local scope they are declared in. Local variables are created when its block of code is entered and these are destroyed once we exit the block.
We cannot use access modifiers (Private, Default, Public, etc.) for the local variables. Local variables don't have any default values and thus, must be assigned some value before using it.
In the above example, localVariable can only be accessed within the exampleMethod(). If this method is called, then only the variable is created and is destroyed as soon as the method completes its execution.
Instance Variable
Instance variables in types of variables in Java, are variables declared inside a class but outside a method, constructor, or block of any code.
The space, for instance, the variable is declared when the object of the class is created using the new keyword. This space is deallocated or the instance variable is destroyed when the object is destroyed.
Instance variables unlike local variables can be used in many methods, constructors, or among classes. We can also assign access modifiers to make these accessible within packages or subclasses. These are usually private but if required can be made to be accessed outside the class as well.
Instance variables can have default values. These are initialized in the class or in a constructor when the object of the class is created. The default value saves the program from crashing if the user accesses the value without defining it.
Instance variables can be normally accessed within the class as local variables with their names. The exception case is a static method where the proper name and designation of the object are required as ObjectReference.variableName for the instance variable.
In the above example, the int variable is an instance variable with its default value 20.
This variable is accessed in the main method, as it is static it requires a proper definition and name of the instance variable.
Static Variable
Class variables in types of variables in Java are declared with the static keyword are static. These are the same for all the objects irrespective of the number of objects. These are declared within the class outside the methods, constructors, or any blocks of code.
The main use case of static variables is to store constant values. It is sometimes used when the single object is modified by multiple methods of the same class and concurrency is required.
These are created when the program starts and are destroyed with the program being destroyed. These can be declared public to make them available to other classes in the package. Normally, these are visible to all the members of the class.
We can simply initialize them with the constant value. These can also be assigned in special static initializer blocks.
Static variables can be used to store the constants or counters. In certain scenarios, a shared state among various objects is required where static variables are useful. Static variables can also be used where data is shared among various threads. These are also used as global variables in Java.
Static variables can be accessed by their class name as ClassName.variableName.
In the example above staticVariable is as follows:
And is called as follows:
Note: Class name is not required when calling in the same class.
Java Variable Examples
Let us look at some of the examples to better understand the behavior and types of variables in Java.
Add Two Numbers
Numbers can be integers, floats, doubles, etc. We can add two numbers using the + operator. Let us first look at the addition of the same type of variables.
Output:
When similar types of variables are added, the numbers are added as expected.
Widening
Widening refers to the process of converting a variable of a smaller data type into a larger data type. The smaller data type is the one that occupies less space and has minimal features. The float data type is larger than int. This type of conversion is performed automatically by the Java Compiler and no information is lost. It is also known as Explicit Type Conversion.
Widening is supported in Java but is not always the best programming practice.
Output:
In the above example int variable is converted into double, i.e. 42 is changed to 42.0.
Narrowing
Many a time we wish to convert a larger number into a smaller number for certain operations. For example, we don't require much precision and would want to convert the floating point number into an integer.
The narrowing may result in a loss of data and the compiler cannot perform on its own. It must be done explicitly by the programmer. It is also known as implicit type conversion
Output:
In the example above, the precision of area is really good due to the value of PI but in practical use cases such high precision is not required and thus, we can convert it into int.
Overflow
Imagine trying to fit 2 liters of water in a 1 liter water bottle - The water flows out of the bottle. Similarly, when we try to fit a larger variable into a smaller space, it overflows and it is not a desired state. Such coding practices must be avoided.
It commonly occurs due to some mathematical operation that results in a value that exceeds the maximum representable value.
Output
maxInt holds the maximum value that can be represented by an int returned by Integer.MAX_INT. As this is the maximum value an integer can hold, further adding even 1 to it causes overflow and therefore, it shows undesired behavior. In this case, adding 1 to the maximum int value wraps around the minimum int value due to overflow.
Adding Lower Type
The addition of two or more lower-type variables may result in overflow and thus, we can always use a larger variable to store these. Let us look at a very basic example of it.
Output:
In the examples above, bytes and shorts are converted to int.
Java Variable Type Conversion & Type Casting
Type conversion and Type casting both are mechanisms of conversion of one data type into another.
Type Conversion | Type Casting |
---|---|
Compiler automatically performs type conversion whenever safe and necessary. The entire control of conversion is in the hands of the compiler, programmer is hardly aware of the conversion. | It is performed by the programmer explicitly. The compiler on its own cannot understand the process of typecasting. |
It is the process of conversion of a smaller data type into a larger one in Java. It is also called Widening. | It is the process of conversion of larger data type into smaller one. Narrowing is also known as typecasting. |
No amount is data is compromised in type conversion as well and no errors are generated. | Data loss is possible, errors may be generated, or may result in unexpected results and thus, must only be performed whenever necessary. |
Note that for type conversion data types must be compatible, for example, string with alphabets cannot be converted to double or float. | It can be performed between compatible and incompatible data types. |
For example, adding an integer and a float implicitly converts the integer to a float first to operate. | Example - Casting a string ("hello") to an integer ((int) "hello") will likely cause a compiler error or unpredictable behavior. |
Differences between the Instance Variables and the Static Variables
Feature | Instance Variables | Static Variables |
---|---|---|
Declaration | Declared inside a class but outside any method or block, with the public, private, or protected access modifiers. | Prefixed with the static keyword and declared inside a class but outside any method or block, with access modifiers. |
Memory Allocation | Each instance of the class has its copy of instance variables. | Shared among all instances of the class; only one copy exists for the entire class. |
Initialization | Instance variables are initialized when an object is created. | Static variables are initialized when the class is loaded into memory. |
Usage in Methods | Instance variables can be used in both instance methods and static methods. | Static variables cannot be directly accessed in instance methods; they are usually accessed using the class name. |
Scope | The scope of an instance variable is limited to the object it belongs to. | The scope of a static variable is at the class level; it is accessible by all instances of the class. |
Conclusion
- There are three types of variables in Java. Local variables are only accessible inside their block of code.
- Instance and static variables are declared inside the class and outside methods, constructors, and blocks of codes.
- Instance variables are different for different objects and can have some default values.
- Static variables are the same for all the objects of the class.
- The compiler performs type conversion to make a smaller class variable compatible with the larger class. The programmer performs type casting to reduce the larger data type variable into smaller ones.