Unary Operator in C
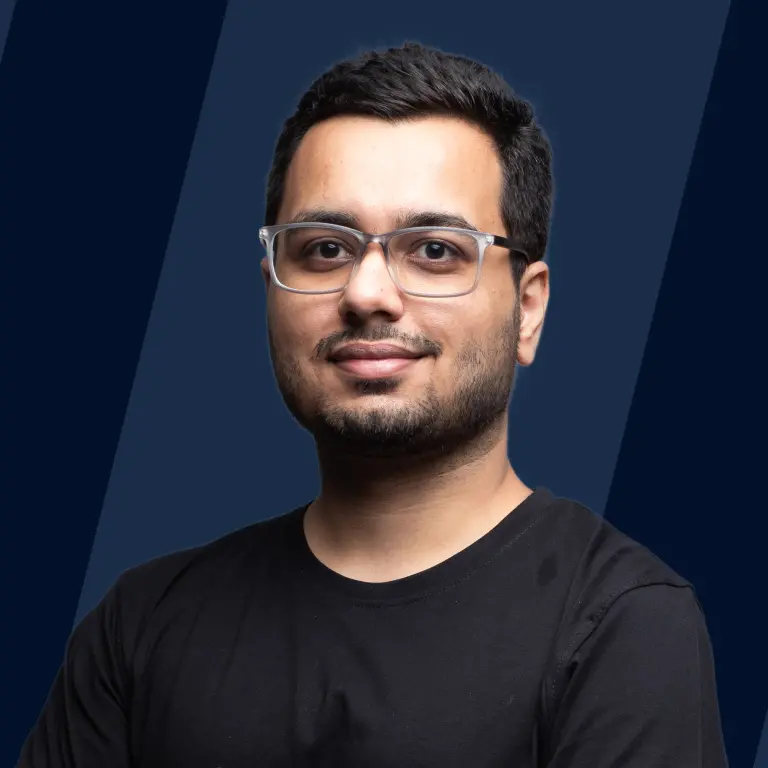
In the C programming language, a unary operator is a single operator that operates on a single operand to produce a new value. The unary operator in C has right to left associativity. All unary operators have equal precedence in C. Unary operators can perform operations such as negation, increment, decrement, and others.
For example, the negation operator (-) negates the value of its operand, while the increment operator (++) adds 1 to its operand. Unary operators can be used to modify variables, perform arithmetic operations, or control the flow of execution.
Types of Unary Operators in C
The types of Unary Operators are as follows:
- Unary plus (+) Operator
- Unary minus (-) Operator
- Increment (++) Operator
- Decrement (--) Operator
- Address of (&) Operator in C
- sizeof() Operator
- Dereferencing (*) Operator
- Logical NOT (!) Operator
- Bitwise NOT (~) Operator
1. Unary plus (+) Operator
Unary plus generally returns the value of the operands.
Syntax:
Example:
Output:
2. Unary Minus (-) Operator
Unary Minus converts positive to negative and negative to positive values.
Syntax:
Example:
Output:
3. Increment (++) Operator
The increment operator is a subtype of the unary operator that uses variables as operands and applies to only one operand. The increment (++) operator increases a variable's value by one. Before or after the increment operator, we cannot use the rvalue (right value).
Pre Increment
The increment (++) operator is applied before the operand in the Pre increment operator. Pre means the first increment, then assign it to another variable.
Syntax:
Example:
Output:
Post Increment
The increment (++) operator is applied after the operand in the Post increment operator. Post means to assign it to another variable and then increment.
Syntax:
Example:
Output:
4. Decrement (--) Operator
The decrement operator is a subtype of the unary operator that uses variables as operands and applies to only one operand. To decrement the value of a variable by one, use the decrement (--) operator. Before or after the decrement operator, we cannot use rvalue (right value).
Pre-Decrement
The decrement (--) operator is applied before the operand in the Pre decrement operator. Pre means first decrement, then assign it to another variable.
Syntax:
Example:
Output:
Post Decrement
The decrement (--) operator is applied after the operand in the Post decrement operator. Post means to assign it to another variable and then decrement.
Syntax:
Example:
Output:
5. Address of (&) Operator in C
Addressof operator (&) is a type of unary operator. Addressof (&) operator returns the address of the variable. Only a basic variable or an array element can be utilized with the & operator.
Syntax:
Example:
Output:
6. sizeof() Operator
The sizeof() operator is a type of miscellaneous unary operator, and the sizeof() operator returns the size of its variable or operand in bytes format.
When the size of an array or structure is unknown to the programmer, the sizeof() operator is typically used to evaluate its length.
Syntax:
Example:
Output:
7. Dereferencing (*) Operator
The Dereferencing operator is a type of unary operator. The character asterisk (*) denotes this operator, which is used to represent the value of any pointer variable.
Syntax:
Example:
Output:
8. Logical NOT (!) operator
Logical NOT (!) is a type of unary operator. The logical not (!) operator is used to complement the condition under consideration. Logical NOT (!) returns TRUE when a condition is FALSE and returns FALSE when a condition is TRUE.
Syntax:
Example:
Output:
9. Bitwise NOT (~) Operator
Bitwise NOT (~) is a type of unary operator. Its job is to complement each bit one by one. In the bitwise not (~) operator, the result of NOT is 0 when the bit is 1 and 1 when the bit is 0.
Syntax:
Example:
Output:
Difference between the Unary Plus and the Unary Minus
Unary Plus | Unary Minus |
---|---|
This operator does not affect the value of the operand. | It converts positive to negative and negative to positive values. |
Unary plus generally returns the value of the operands. | This operator turns the value into a negative value. |
Difference between Prefix and Postfix Increment/Decrement
Difference between prefix increment and postfix increment
Prefix Increment | Postfix Increment |
---|---|
Prefix Increment operators applied before the variable. | Postfix Increment operators applied after the variable. |
First increment the value and then use it in the equation after completion of the equation. | First, use the value in the equation and then increment the value. |
Ex: x = ++y | Ex: x = y++ |
Difference between prefix decrement and postfix decrement
Prefix Decrement | Postfix Decrement |
---|---|
Prefix Decrement operators applied before the variable. | Postfix Decrement operators applied after the variable. |
First decrement the value and then use it in the equation after completion of the equation. | First, use the value in the equation and then decrement the value. |
Ex: x = --y | Ex: x = y-- |
Learn More
To learn more about operators in C, check the below link.
Conclusion
- In C, we use unary operators to perform operations on unary operands.
- To manipulate bits of a number, use the bitwise NOT operator of the unary operator in c.
- The unary plus and minus operators are not the same as the arithmetic operators.