Unary Operator in JavaScript
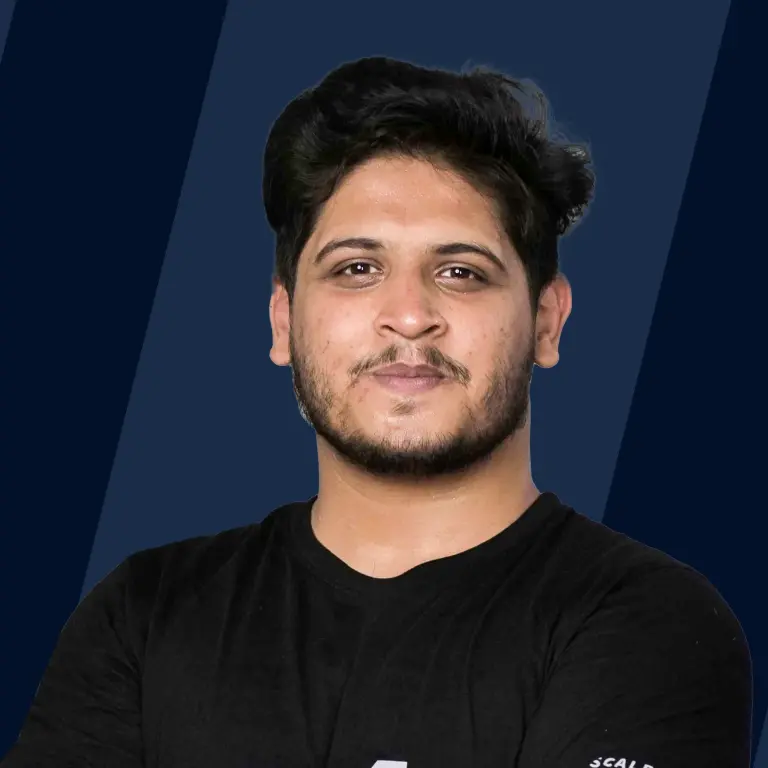
To perform a mathematical operation like addition, subtraction, multiplication, or division in javascript, we need at least two operands, but the unary operator in javascript works with only one operand and performs various unary operations like increment, decrement, converting a value into a number, etc.
As an example, we applied the increment operator on a variable to increment its value by one :
Output :
Above, we applied the increment operator on variable myVal and logged myVal on the console. As a result, we print 11 on the console, an increment of one in the original value of myVal.
What are the Types of Unary Operators in JavaScript ?
There are the following types of unary operators in javascript :
Operator | Explanation |
---|---|
Increment(++) | Increment of the operand by one. |
Decrement(--) | Decrement of the operand by one. |
Unary plus(+) | Try to convert the operand into a number value. |
Unary negation(-) | Try to convert the operand into a number value and negate the value. |
Logical Not(!) | Convert the operand into a boolean value then negate it. |
Bitwise NOT(~) | Invert all the bits of the operand and return a number. |
Void | Used for returning the undefined after evaluating an expression. |
delete | Remove the property of an object. |
typeof | Return the data type of the operand. |
Examples for Unary Operator in JavaScript
Increment Operator(++)
Increment operator in javascript increment the operand by one. It can be used as a prefix(++ operand) or as a postfix(operand ++).
As an example, we applied the increment operator on a variable to increment its value by one :
Example :
Output :
Above, we applied the increment operator on variable myVal and logged myVal on the console. As result, we print 11 on the console which is an increment in the original value of myVal.
Using the increment operator as a postfix or as a prefix works the same and increments the operand by one. But, the difference is, using the increment operator as a postfix returns the value after the increment, and using the increment operator as a prefix returns the value before the increment.
Using the increment operator on an operand, increment the operand by one. But, using it as a postfix on an operand returns the value after the increment, and using it as a prefix on an operand returns the value before the increment.
Decrement operator(--)
The decrement operator in javascript decreases the operand by one. It can be used as a prefix(++ operand) or as a postfix(operand ++).
As an example, we applied the decrement operator on a variable to decrease its value by one :
Example :
Output :
Above, we applied the decrement operator on the variable myVal and logged myVal on the console. As result, we print 9 on the console which is a decrement in the original value of myVal.
Unary Plus Operator(+)
Unary plus operator in javascript converts the operand to a number value. We have to use it as a prefix(+ operand) and if the operand can't be converted into a number then it will return NaN.
As an example, we applied the unary plus operator on a variable to convert its value into a number value :
Example :
Output :
Above, variable myVal is having a string value of "10". We applied the unary plus operator on variable myVal and logged it on the console. As a result, we printed 10 on the console, it's a conversion of variable myVal from string value "10" to a number value 10.
Some more examples are given below :
Unary plus operation | result |
---|---|
+"20" | 20 |
+20 | 20 |
"myname" | NaN |
+true | 1 |
+false | 0 |
+null | 0 |
Unary Negation Operator(-)
Unary negation operator in javascript converts the operand to a number value and negates it. We have to use it as a prefix(- operand) and if the operand can't be converted into a number then it will return NaN.
As an example, we applied the unary negation operator on a variable to convert its value into a number and negate it :
Example :
Output :
Above, the variable myVal is having a string value of "10". We applied the unary negation operator on the myVal variable and logged it on the console. As a result, we print -10 on the console, it's a conversion and negation of the myVal variable from string value "10" to a negative number value -10.
Some more examples are given below :
Unary plus operation | result |
---|---|
-"20" | -20 |
-20 | -20 |
-"myname" | NaN |
-true | -1 |
-false | -0 |
-null | -0 |
Logical Not (!)
Logical Not operator in javascript converts the operand to a boolean and negates it. It is used as a prefix(! operand), and can also be used as a double negation operator by writing it two times(!! operand).
As an example, we applied the logical not operator on a variable to convert its value into a boolean and negate it :
Example :
Output :
Above, we applied the logical Not operator on the myVal variable and logged it on the console. As result, we printed the false on the console because the boolean of 10 is true and after negation, it becomes false.
Some more examples are given below :
Logical Not operation | result |
---|---|
!"20" | false |
!20 | false |
!"myname" | false |
! 0 | true |
!false | true |
!null | true |
Bitwise NOT (~)
Bitwise NOT operator in javascript inverts the bits of operand. Applying it to a number results in : -(number + 1), one will get added to the number then the final value will be multiplied with -. We have to use the bitwise Not operator as a prefix(~ operand).
As an example, we applied the bitwise NOT operator on a variable to inverse its value like this :
Example :
Output :
Above, we applied the bitwise Not operator on a variable myVal and logged it on the console. As a result, we printed -5 on the console because a bit of 4 of getting inverted.
Some more examples are given below :
Bitwise NOT operation | result |
---|---|
~ -4 | 3 |
~ -4.5 | 3 |
~ true | -2 |
~ false | -1 |
~ null | -1 |
Void
The void operator in javascript is used to return the undefined after evaluating an expression. It can be used as a prefix void expression or void (expression), both are valid but using pair of opening and closing brackets () is preferred.
As an example, we applied the void operator over a function. As a result, it will return undefined after evaluating the expression.
Example :
Output :
Above, we applied the void operator on function myFun and called myFun in the console. As a result, we printed undefined on the console because void returned undefined.
Void is frequently used to add expression as a hypertext link, here is an example of using void on a link :
The expression above creates a hypertext link, on clicking, it will submit the form.
delete
The delete operator in javascript is used to remove a property from an object. It is used as a prefix delete expression where the expression should always be the reference of an object property.
As an example, we applied the delete operator on an object to delete an object propertie like this :
Example :
Above, we use the delete operator on an object myObj to remove a property from it and then logged the object myObje on the console. As result, we printed { age: 20 } on the console, because the name property gets removed after using the delete operator.
typeof
The typeof operator in javascript is used to know the operand data type. It returns the data type of the operand in string format. The typeof operator is used as a prefix(typeof operand).
As an example, we applied the void operator on a variable to know about its data type like this :
Example :
Output :
Above, we applied the typeof operator on the myName variable and log it on the console. As a result, we printed string on the console because the data type of the myName variable is a string.
Some more examples :
typeof operation | result |
---|---|
typeof 4 | number |
typeof 4.5 | number |
typeof "name" | string |
typeof true | boolean |
typeof null | object |
Learn More
The ternary operator in javascript is a clear, concise, and short conditional operator to take conditional decisions without using if and else conditions, to learn more check out ternary operator in javascript.
Conclusion
- Unary operator in javascript is used to perform various unary operations like increment, decrement, converting a value into a number, etc.
- Unary operator works with only one operand.
- A unary operator comes either before or after an operand.