What is Unary Operator Overloading in C++?
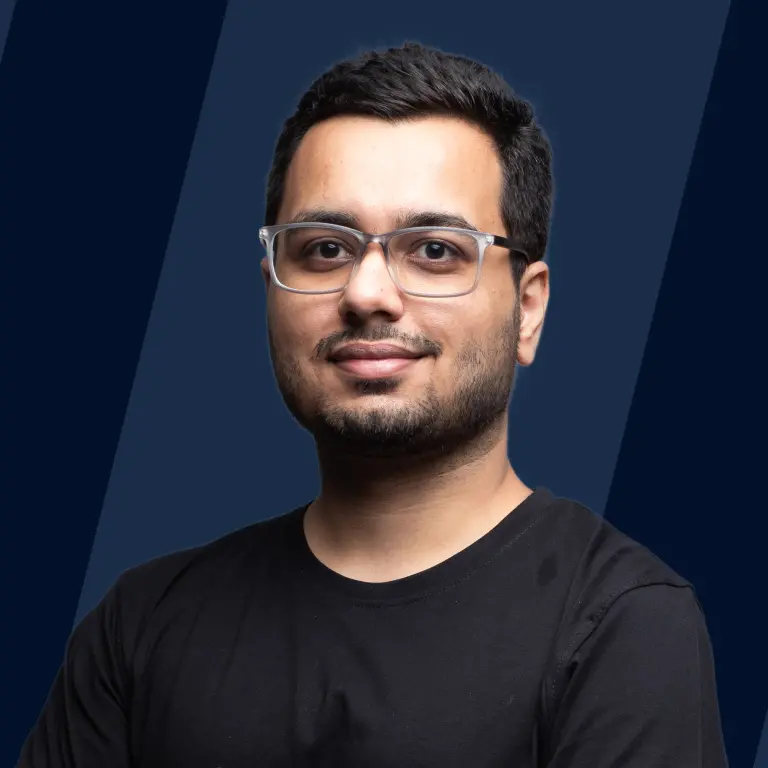
In operator overloading, we overload an operator to perform a similar operation with the class objects; it is polymorphism. We can not use the simple unary operators with the class objects as the operators will not understand the behavior of the member variables, and it will throw a compilation error. We do operator overloading to give operators a user-defined meaning, which is utilized for user-defined data type (object of a class) operations.
We can perform unary operator overloading in C++ by adding a new operator function definition in the class itself or by using the global friend function created for the operator function. The syntax for unary operator overloading in C++ is given below:
Syntax:
What is a Unary Operator in C++?
To conduct mathematical and logical operations on numerical quantities, C++ includes a wide variety of operators. The unary operators are one such extensively used operator. Unary Operators work on the operations carried out on just one operand. Unary operators do not utilize two operands to calculate the result as binary operators do.
A single operand/variable is used with the unary operator to determine the new value of that variable. Unary operators are used with the operand in either the prefix or postfix position. Unary operators come in various forms and have right-to-left associativity and equal precedence.
These are some examples of, unary operators:
- Increment operator (++),
- Decrement operator (--),
- Unary minus operator (-),
- Logical not operator (!),
- Address of (&), etc.
Let's understand unary operator overloading in C++ by using an example.
Example
A. Overloading Unary Operators for User-Defined Classes:
Defining a Complex user-defined class in a C++ Program:
C++ Program:
There are two ways for unary operation overloading in C++, i.e.,
- By adding the operator function as a class member function.
- By using the global friend function created for the operator function.
Let's see both methods below using the Complex class defined above.
B. Overload Unary Minus (-) Operator using class Member function
C++ Program:
Output:
Explanation:
- In the above C++ program, we have overloaded a minus (-) unary operator to work with the Complex class objects.
- We can't simply use the minus (-) operator with the Complex class object. It will give a compilation error until we have defined the minus (-) operator w.r.t to the Complex class objects.
- We have provided a minus (-) operator overloaded definition in the Complex class. It is invoked when we have used the unary minus(-) operator in the main() function with the c2 object.
C. Overload Unary Minus (-) Operator using Global Friend Function
C++ Program
Output:
Explanation:
- In the above C++ program, we have made a friend function in the Complex with a global overloaded minus (-) unary operator function.
- In C++, a friend function is a special function that does not belong to a class but can access its private and protected data.
- We have provided a global minus (-) operator overloaded definition outside the Complex class as a global friend function that can access the real and img variables. It is invoked when we have used the unary minus(-) operator in the main() function with the c2 object.
Learn More
- Learn more about operator overloading here, Operator Overloading in C++.
- Checkout our Free C++ Course to master C++ and get certified.
Conclusion
- Unary Operators in C++ work on the operations carried out on just one operand/variable.
- Following are some of the examples for unary operators, Increment operator (++), Decrement operator (- -), Unary minus operator (-), Logical not operator (!), etc.
- Unary operator overloading in C++ is polymorphism in which we overload an operator to perform a similar operation with the class objects.
- We do operator overloading to give operators a user-defined meaning, which is utilized for user-defined data type (object of a class) operations.
- We can perform unary operator overloading by adding a new operator function definition in the class itself or by using the global friend function created for the operator function.