Unchecked Exception In Java
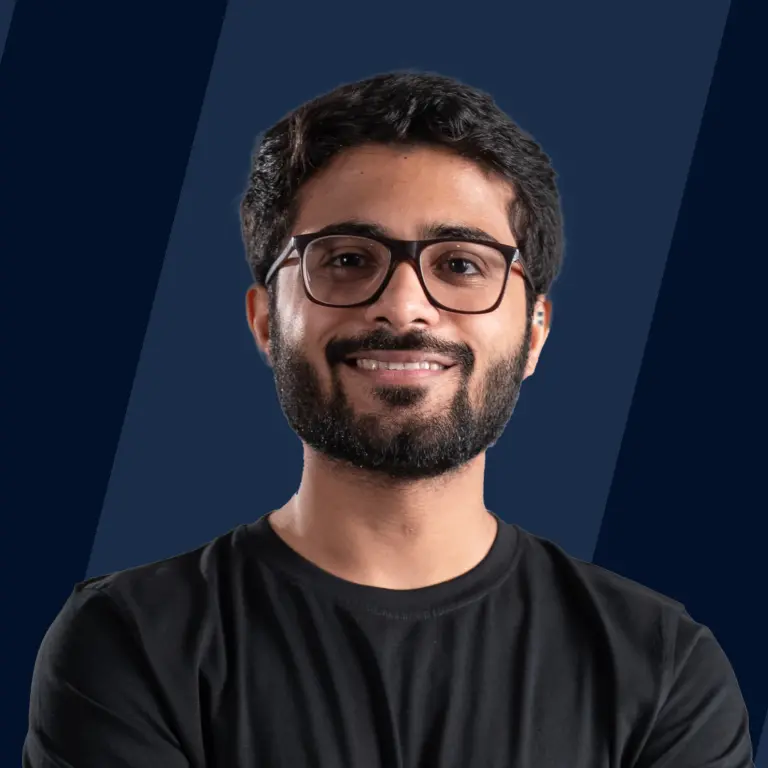
What is an Unchecked Exception in Java ?
It occurs at the time of execution and is known as a run time exception. It includes bugs, improper usage of API, and syntax or logical error in programming.
In Java, exceptions that are under Error and , Runtime exception classes are unchecked exceptions.
Types of Unchecked Exceptions
- ArithmeticException
- NullPointerException
- ArrayIndexOutOfBoundsException
- NumberFormatException
- InputMismatchException
- IllegalStateException
Arithmetic exception :
It is a type of unchecked error in code that is thrown whenever there is a wrong mathematical or arithmetic calculation in the code, especially during run time. For instance, when the denominator in a fraction is zero, the arithmetic exception is thrown.
NullPointerException :
It is a run time exception. It is thrown when a null value is assigned to a reference object and the program tries to use that null object.
ArrayIndexOutOfBoundsException :
It occurs when we access an array with an invalid index. This means that either the index value is less than zero or greater than that of the array’s length.
NumberFormatException :
It is a type of unchecked exception that occurs when we are trying to convert a string to an int or other numeric value. This exception is thrown in cases when it is not possible to convert a string to other numeric types.
InputMismatchException :
It occurs when an input provided by the user is incorrect. The type of incorrect input can be out of range or incorrect data type.
IllegalStateException :
It is a run time exception that occurs when a method of a code is triggered or invoked at the wrong time. This exception is used to give a signal that the method is invoked at the wrong time.
Examples
Example of Arithmetic Exception :
Output :
In this, when the number 1 is divided by 0, an exception is thrown at a run time.
Example of NullPointerException
Output :
In this, the null pointer exception is thrown at a run time when the reference of the object is null.
Example of ArrayIndexOutOfBoundsException
Output :
In this, the for loop starts from 0 and ends at the length of the array which is 4 in this case. Because the index pointer 'i' is equal to the length of an array which is 5, it iterates 5 times in an array and prints the exception because several elements in an array are less than the length.
Example of NumberFormatException
Output :
In this, instead of a string, a null value is passed an exception occurs.
Example of InputMismatchException
Output :
In this example, instead of passing an int value for the age, the string value is passed. This caused an exception.
Example of IllegalStateException
Output :
When to Use Unchecked Exception in Java ?
If a developer does not want the error to be solved (considering it does not crash the system) then unchecked exceptions should be used. It is used for caching the checked exception when it is unable to handle the error.
Unchecked Exceptions — The Controversy
Java language does not require a specific method to catch or specify unchecked exceptions. Hence programmer might write a code that throws only unchecked exceptions to make all other exception subclasses inherit the run time exception.
Both methods allow programmers to write code without even bothering about compiler errors or any other exceptions in the code.
The conclusion is that if the user expects to recover from the exception in the code then checked exception can be used else for any other cases, unchecked exceptions are a way to go.
Learn More
Conclusion
- Unchecked exception occurs at the time of execution also known as run time exceptions. It includes bugs, improper usage of API, and syntax or logical error in programming.
- Types of Unchecked Exception :
- ArithmeticException
- NullPointerException
- ArrayIndexOutOfBoundsException
- NumberFormatException
- InputMismatchException
- IllegalStateException
- If a developer does not want the error to recover then unchecked exceptions should be used. It is used for caching the checked exception when it is unable to handle the error.