What is Upcasting And Downcasting in Java?
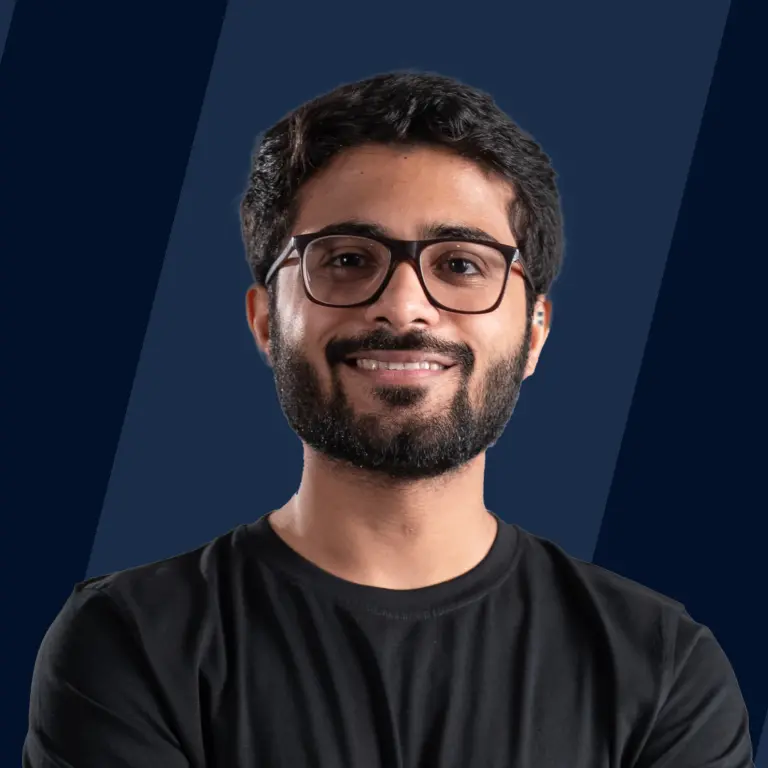
What is Upcasting and Downcasting in Java?
Upcasting (or widening) is the typecasting from a subclass to a superclass (Child to Parent class). In this way, we can access the properties (methods and variables) of the superclass, i.e., the Parent as well. Downcasting (or narrowing) is typecasting from a superclass to a subclass so that we can access the methods of the subclass, i.e., the child class.
Upcasting and downcasting in Java are the two forms of typecasting where conversion of data type takes place. Typecasting is one of the important concepts in Java where the conversion of data types can be used for various purposes. Here, we're going to see how the conversion of an object takes place (because we have usually seen the typecasting in the case of datatypes only).
Basically, we'll be typecasting an object to a data type and there would be a parent and child object for it. The purpose of typecasting is to enable a function in a program to process the variables correctly.
Let's understand the meaning and differences related to upcasting and downcasting in Java.
Upcasting in Java
Upcasting, in simpler words, is the typecasting of a child object to a parent object.
Notice in this image that the reference variable is of the type Parent whereas the object is created of the type Child. This is known as upcasting. The reason is that the child object created here can show the properties of its parent, therefore it's correct to put the reference of its parent.
Let's take up an example of sports. It's a personal choice to be interested in one sport or the other, be it cricket, football, hockey, etc. So, it's correct to say that Cricket is-a sport or Football is-a sport (IS-A relationship).
Sport, in common, is a parent object and each of its different forms is its child object and they have an IS-A relationship.
Now, let's see how we are going to understand it in terms of upcasting. Sport is a parent object whereas Cricket and Football are the child object. Each of these child objects inherits the properties from their parent, i.e. Sport. And a child object can definitely exhibit the properties of its parent, which is why a child object can be implicitly typecasted as a parent object.
Look at the code below which show how this implicit typecasting is possible.
Output:
Syntax of Upcasting
Downcasting in Java
Downcasting refers to typecasting a parent object to a child object. However, this cannot be an implicit typecasting.
Why?
Because it's correct to say that Cricket is a Sport or Football is a Sport. But, we cannot say that, in particular, Sport is a Cricket or Sport is a Football. It doesn't have a single child but multiple children.
When you try to create an object of the type Parent and assign it the reference variable of type Child, you will get a runtime error. But wait, if downcasting is not allowed in Java, then we should receive an error during the compile-time only. It means that downcasting is possible but has a certain limitation. Let's discuss this limitation in detail.
While downcasting, it is important to verify beforehand that the object created is of the type Child irrespective of the reference variable. This tells the compiler the runtime type of the object.
An example will make it more clear.
We created an object of the type Cricket with the reference of the type Sport. The explicit casting informs the compiler about the runtime type of object. Since the object creation type was Cricket initially, downcasting is possible in this case.
Let's take up another scenario where the downcasting isn't possible.
This throws ClassCastException because the runtime object of the sport is Sport. It should've been Cricket, like in the above case. Therefore, this superclass to subclass casting isn't possible.
Output:
If you want to access the methods of a subclass, then downcasting will be required. On the contrary, there are also probable chances of getting a ClassCastException. So, it's better to first provide Parent class reference to the Child class object. Then perform explicit casting of superclass reference to subclass type. Seems confusing? Let's look at this example code.
Output:
Syntax of Downcasting
Difference between Upcasting and Downcasting in Java
Upcasting | Downcasting |
---|---|
Upcasting refers to typecasting a child object to a parent object. | Downcasting provides casting a parent object to a child object. |
Implicit, as well as explicit upcasting, is allowed. | Implicit downcasting is not allowed in Java. |
Methods and variables of the Parent class can be accessed. | Methods and variables of both the Parent and Child classes can be accessed through downcasting. |
Parent p = new Child(); | Parent p1 = new Child(); Child c1 = (Child)p1; |
Conclusion
- Upcasting and downcasting in Java are the two forms of typecasting. The purpose of typecasting is to enable a function in a program to process the variables correctly.
- Upcasting refers to typecasting a child object to a parent object.
- Downcasting provides casting a parent object to a child object.
- Both implicit, as well as explicit upcasting, is allowed.
- Implicit downcasting is not allowed in Java
- Syntax for upcasting: Parent p = new Child();
- Syntax for downcasting: Parent p1 = new Child(); Child c1 = (Child)p1;