What are user defined data types in C?
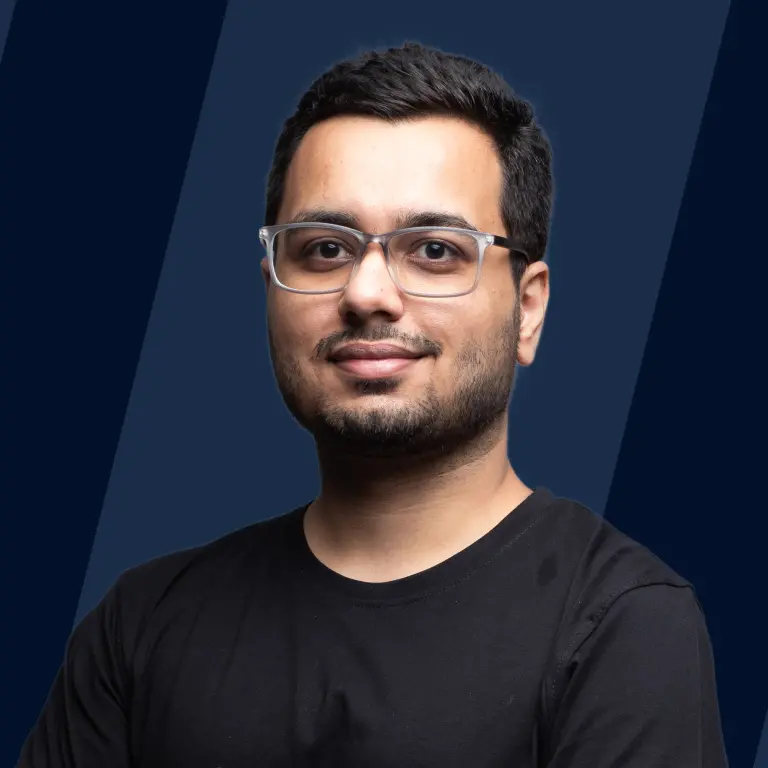
The data types that are defined by the user depending upon the use case of the programmer are termed user-defined data types. C offers a set of constructs that allow users to define their data types. These user-defined data types are constructed using a combination of fundamental data types and derived data types. Fundamental data types are basic built-in types of C programming language. These are integer data type (int), floating data type (float), and character data type (char). Derived data types are derived from fundamental data types, like functions, arrays, and pointers in the C programming language. For example, an array is a derived data type as it contains elements of a similar data type and acts like a new data type in the C programming language. User-defined data types are created by the user using a combination of fundamental and derived data types in the C programming language. To create a user-defined data type, the C programming language has the following constructs:
- Structures (struct)
- Union
- Type definitions (Typedef)
- Enumerations (enum)
- Empty data type (void)
Why do we Need User-Defined Data Types in C?
- User-defined data types in C are highly customizable, depending upon the use case of the programmer.
- User-defined data types in C increase the readability of the program as data types can be given meaningful names.
- User-defined data types increase the extensibility of the program to introduce new data types and manage data in the application code.
- If we do not use user defined data types in C, then it is very difficult to maintain multiple variables that represent information belonging to the same entity in the program logic.
Let us take an example to understand why we need user-defined data types. Suppose we have to implement a C program where we want to maintain the following information:
- Records of student like name, age, school name and class, and date of birth.
To do this, if we do not use any user defined data types in C, then we will have to use multi-dimensional arrays to represent the information in the following way:
We can represent the above data using user defined data types in C. Below we will discuss the use of structures in C to represent the data and typedef to give a meaningful name to the structure.
You can see the difference in implementation in the two examples given above. In general, when a software program is written, the developer needs to make sure that the code is readable, clean, and maintainable. Using user-defined data types, these things can be implemented.
Types of User-Defined Data Types in C
Structures
Structures are used to package multiple data types that represent the properties of a single entity. Multiple or same data types can be represented under the same name using a structure in C. Structures are used to group multiple variables of different data types. It gathers together, different atomic information that comprises a given entity. For example, an entity Book has many attributes like title, number of pages, author, publisher, date of publishing, etc. All this information can be represented using a clean data structure using structures in C. Members inside the structures are referred to as data members or fields. Members of the structures can be accessed using the dot (.) operator.
Declaration
To define a structure we use the keyword struct in the C programming language.
struct <name> {
<data type 1> <variable name 1>,
<data type 2> <variable name 2>,
...
};
Example
Output
For a detailed discussion on structures in C, read the following link: https://www.scaler.com/topics/c/structures-c/
Union
A Union is a user-defined data type in C. It is a collection of different data types in the C programming language that allows users to store different data types at the same memory location in the RAM. Unions are like structures in the C programming language with an additional property that all members of a union share the same memory location in the RAM. We can define a Union with many data members, but only one member can contain a value at any given time. The memory of the Union data type is equal to the size of the data member of the Union with the highest number of bytes in size while the memory required in the case of structures in C is the sum of total bytes required by all the data members of the structure.
Declaration
A Union is declared using the keyword union and members are accessed using the dot (.) operator.
Example
Output
In the above example, if we compare the size of the struct and union with the same data members then we can see that the size of the struct book is the sum of the size of all its data members. In contrast, the size of the union Book is the size of the data member with the maximum size in bytes. Moreover, changes in the data member pages is reflected in the data member prices. This means both the variables share the same memory location and changes in one will be reflected upon the other.
Type Definitions (typedef)
Type definitions allow users to define an identifier that would represent a data type using existing or predefined data types. It is used to create an alias or an identifier for an existing data type by assigning a meaningful name to the data type. The identifier is defined using the keyword typedef. The main advantage of using typedef is that it allows the user to create meaningful data type names. This increases the readability of the program.
Declaration
typedef type identifier
OR
typedef existing_data_type_name new_user_defined_data_type_name
Example
In this first example, we declare a struct with two fields and define its type as Book. In the second example, we define the type of int as number so we can use the data type number to initialize a variable with an integer value. In the third example, we define the type of float as decimal so we can use the keyword decimal to declare a float variable.
Enumerations (enum)
Enumerations is another user-defined data type in C. Enumerations in C are a way to define constants so that they can be used in the program. These constants are usually defined globally and are used throughout the code. The constant values are generally integers and are given meaningful names to increase the readability of the program and highlight the need for the constants being used. Related constants can be defined in the set using Enumerations in C. This set of named values is called elements or members. The enumeration in C is used to assign a constant integer value to variable names. The constant values can be assigned by the user. Each value is associated with a unique name. This unique named constant is global and cannot be reused in some other enumeration. Users can assign the value or if these values are not assigned then the compiler will assign the values by default starting from 0. Note that two enums cannot have the same variable names as constants as enums are global in the C programming language.
Declaration
Enumerations are declared using the keyword enum.
Example
Output
Empty Data Type (void)
The keyword void is an empty data type that is used in functions and pointers. It does not represent any value. In the case of functions, it specifies that the function does not return any value and in the case of pointers, it specifies that the pointer is universal. C11 standard defines the keyword void as:
The void type comprises an empty set of values; it is an incomplete object type that cannot be completed.
- In the case of functions, it represents the return type of the function. It means the function does not return any value to the calling function.
- In the case of pointers, it represents that the pointer does not specify what it points to. It can point to any data type.
Declaration
Example
Output
- In this example, we can see the use of void in the case of function and pointers. In the case of functions, when we do not return any value, we need to specify that the function has a void return type.
- In the case of pointers, the same void* pointer can be used to point to an int variable or a float variable.
Conclusion
- User-defined data types in C can be used to represent the data in a logical way. It increases readability and makes the logic of the program, clear and simple.
- Structures in the C programming language are used to create a collection of multiple data types that represent properties of the same entity.
- Union is useful when we want to reuse memory locations. They are similar to the structure but with the additional property that the memory is shared between all the data members in the union.
- Type definitions are used to enhance the readability of the program by giving meaningful names to the existing data types.
- Enumerations are useful for assigning names to integral constants. They increase the readability of the program.
- Void data types are used with functions and pointers. In the case of functions, it specifies that the function does not return any value. In the case of pointers, it specifies that the pointer does not specify what it points to.