What is User Defined Exception in Java?
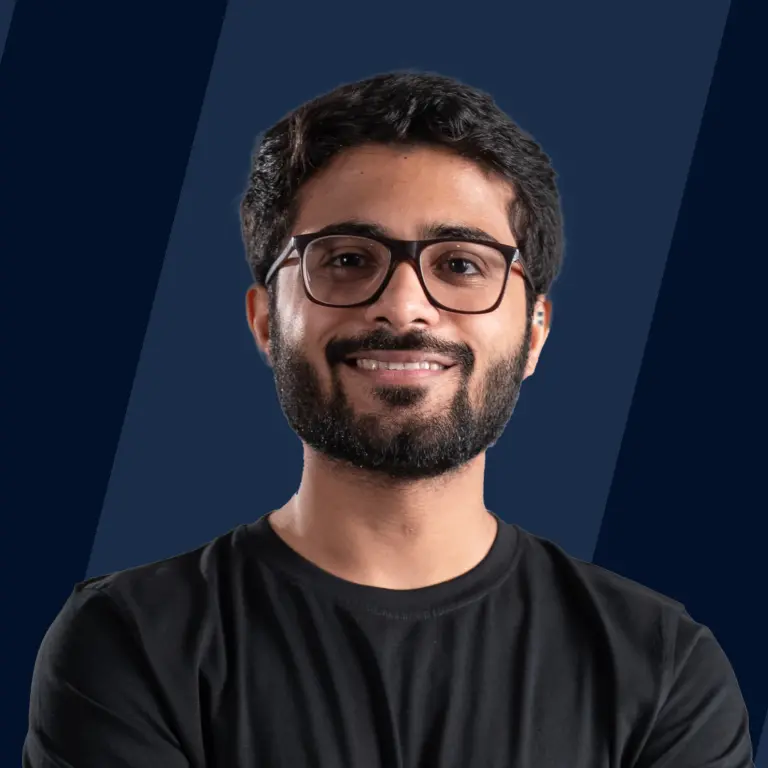
An exception is a case where the normal flow of the program execution is disrupted.
Java provides a few built-in exceptions, which are again segregated as compile-time and runtime exceptions. We are in a sunny day scenario until our use case is satisfied with one of the built-in exceptions, but what if there's no such exception that fits our use case, or do we want to customize the exception?
User-defined exceptions are also referred to as custom exceptions. The exceptions created per our use case and thrown using the throw keyword are user-defined exceptions, and such exceptions are derived classes of the Exception class from the java.lang package.
Why Use Custom Exceptions?
Although java provides the Exception class, which covers almost all cases of exceptions that could be raised during program execution, custom exceptions will bring the spotlight onto exception handling.
Custom exceptions enable the flexibility of adding messages and methods that are not part of the Exception class. They can be used to store case-specific messages like status codes and error codes or override an existing method to present the exception as per the use case.
Custom exceptions are helpful while writing exceptions for business logic. It helps the application developers to better understand the exception, which is business-specific.
Now that we are aware of custom exceptions in java and their use cases, let's have a look at how to create them.
How to Create User-Defined Exceptions in Java?
To create a custom exception in java, we have to create a class by extending it with an Exception class from the java.lang package.
It's always a good practice to add comments and follow naming conventions to easily identify and recognize the benefit of our exception class.
Below is the template to be followed for creating a user-defined exception in java.
Let's add our custom message to the exception class. This can be done in two ways :
1. Pass the Exception Message to Super Class Constructor and Retrieve It Using the getMesssage() Method
Here's the code to demonstrate that in java
In the above code, we've made a call to the parent class constructor using the super keyword. Since the custom class is extended by the Exception class, it is considered to be the parent class, and the Exception class has a parameter constructor which expects a string. It will construct a new exception with the specified message that can be retrieved later using the getMessage() method.
Learn more about this and super keyword in Java from this informative article over here.
2. Override the toString() Method and Customize It with Our Exception Message
Here's the code to demonstrate that in Java
In the above code, we've overridden the toString() method. Usually, when we print an object of a java class, it prints the hashcode of that object (default implementation of the toString() method). Overriding the toString() method provides the flexibility to customize the print statement when we print an object. Now that we've overridden the method with our custom message, the user only needs to print the exception object to know the exception message. Easy, right?
Now that we've seen how to create a user-defined exception class in Java, let's see how to use our custom exception and have a walkthrough over a few more examples.
Examples
1. Simple User-Defined Exception in Java
In order to use our custom exception, we've to create a new object of the class and throw it using the throw keyword.
Here's the code to demonstrate that in Java
Note that the throw keyword has to be inside a try block, and the corresponding exception is caught in a catch block.
Call to the super constructor has to be the first statement inside a constructor.
2.User-Defined Exception for Validating Login Credentials
Consider a use case where we want to validate the login credentials entered by the user and throw an exception with a specific error message or a specific status code to make the user better understand the exception. We can define a custom exception for this case. Let's have a look over it.
In the above Java program, we've defined a custom exception, InvalidCredentialsException and added our specific error message. In the main method, we've updated the id and password variables with user input. We've put a conditional check to validate the credentials inside a try block, which throws an InvalidCredentialsException if the credentials are not valid. Then we've written a catch block to print our custom exception message.
3.User-Defined Exception for Value Less than Threshold Value
Consider a use case where a value has to be entered by a user or returned by another process, and the value has to be greater than a threshold value; else, we've raised an exception. This exception matches the example case where a user is trying to withdraw some amount, and the amount cannot be negative or zero. Let's have a look over the custom exception for this case in Java.
In the above java program, we've defined a custom exception, AmountLessThanRequiredException, and added our specific error message. In the main method, we've updated the withdrawAmount variable with user input. We've put a condition to check if the given withdrawal amount is less than or equal to zero inside a try block, which throws an AmountLessThanRequiredException if credentials are not valid. Then we've written a catch block to print our custom exception message.
4. User-defined Exception for Validity of an Entity
Consider a use case where a user is trying to access or log in with expired entity details, for example, a user trying to log in to an application where the provided credentials are no more active. In such a case, a user-defined exception with a custom message helps the user better understand the exception.
Here's the code to do that in Java.
In the above java program, we've defined a custom exception, IdDoesnotExistException, and added our specific error message. In the main method, we've updated the id variable with user input. Let's imagine that there's a function named getIdList() which returns a set of all active IDs. We've put an if condition to check if the given id is active inside a try block, which throws an IdDoesnotExistException if the id is not active or invalid. Then we've written a catch block to print our custom exception message.
5.User-defined Exception for Validating the Age of a User
Consider a use case where the user has to input an integer, and the value must be greater than or fit in a range, else we've to raise an exception. This exception matches the example case where the user tries to register for voter ID, which has an age restriction of 18. Let's create a custom exception for this case with a custom message describing the exception.
Let's have a look over the code!
In the above java program, we've defined a custom exception, AgeDoesNotFitException, and added our specific error message. In the main() method, we've updated the age variable with user input. We've put a conditional check to compare the given age with the required age limit inside a try block, which throws an AgeDoesNotFitException if credentials are not valid. Then we've written a catch block to print our custom exception message.
Learn More
Now that we’ve explored the definition of user-defined examples and walked through a few examples and use cases, you might have built up some interest in the concept of exception handling in Java (of course, it is an interesting topic). Fasten your enthusiasm by reading this interesting blog on exception handling over here.
Explore Scaler Topics Java Tutorial and enhance your Java skills with Reading Tracks and Challenges.
Conclusion
Let's recall
- An exception breaks the normal execution flow.
- User-defined exceptions provide the flexibility to customize the exception as per our use case.
- A custom exception class must extend the Exception class from the java.lang package.
- Error message of a custom exception can be configured by passing a message to the super class constructor or by overriding the toString() method.
- To use our custom exception, we've to create a new object of the custom exception class and throw it using the throw keyword.