java.util Package in Java
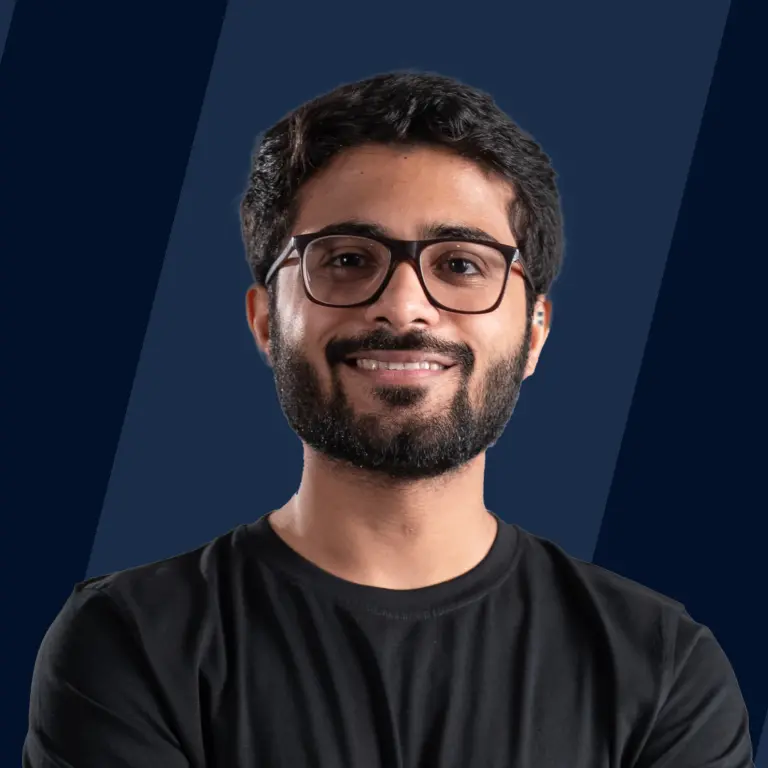
The java.util package in Java is a built-in package that contains various utility classes and interfaces. It provides basic functionality for commonly occurring use cases. It contains Java's collections framework, date and time utilities, string-tokenizer, event-model utilities, etc.
Introduction to java.util Package in Java
To support the needs of a software developer, Java provides various built-in and pre-written functionalities in the form of packages. These built-in packages contain various kinds of classes and interfaces that can be used to develop better and maintainable code. Some of the built-in Java packages are shown in the figure given below:
One of the most important Java packages is the java.util package. The java.util package contains several pre-written classes that can act as a base to solve commonly occurring problems in software development. It provides basic and essential source code snippets to the programmers that can lead to clean and maintainable code. It contains various classes and methods that perform common tasks such as string tokenization, date and time formatting, Java Collections implementation, etc.
What does import java.util do in Java?
To load the basic utility classes and interfaces provided by the java.util package, we need to use Java's import keyword. The import keyword is used to access and load the package and its respective classes into the Java program. The syntax to import the package or its classes is given below:
Here, the first import statement is used to load a certain class from the specified package, whereas the second statement is used to import the whole package into the Java program. For the java.util package:
The above statement is used to load all the functionalities provided by the java.util package.
java.util Package Import Statement Example
Let's look at some examples to understand the use of import statements while loading the java.util package:
Example 1: To Load the Whole Util Package
As discussed, this statement will load all the pre-written classes and interfaces provided by the java.util package.
Example 2: To Load a Specific Class from the java.util Package
The above statement is importing the Scanner class available in the java.util package. This class is widely used to take input from the user in a Java program.
What is the Use of java.util Package in Java?
The java.util package in Java consists of several components that provide basic necessities to the programmer. These components include:
Let's explore these components and their usage in Java:
- Data Structure Classes: The java.util package contains several pre-written data structures like Dictionary, Stack, LinkedList, etc. that can be used directly in the program using the import statements.
- Date and Time Facility: The java.util package provides several date and time-related classes that can be used to create and manipulate date and time values in a program.
- Enumeration: It provides a special interface known as enumeration(enum for short) that can be used to iterate through a set of values.
- Exceptions: It provides classes to handle commonly occurring exceptions in a Java program.
- Miscellaneous Utility Classes: The java.util package contains essential utilities such as the string tokenizer and random-number generator.
What is Collections Framework in Java?
Collections Framework in Java is a special part of the java.util package that can be used to represent and manipulate collections. It provides easy storage and organization of a group of objects (or collection). It includes pre-written classes, interfaces, and algorithms under a unified architecture.
java.util Package Interfaces
Interface | Description |
---|---|
Collections | Root interface for the Collections API |
Comparator | Provides sorting logic |
Deque | Implements Deque data structure |
Enumeration | Produces enumeration objects |
Iterator | Provides iteration logic |
List | Implements an ordered collection (Sequence) |
Map | Implements Map data structure (key-value pairs) |
Queue | Implements Queue data structure |
Set | Produces a collection that contains no duplicate elements |
SortedSet | Produces a set that remembers the total ordering |
java.util Package Classes
Class | Description |
---|---|
Collections | Provides methods to represent and manage collections |
Formatter | An interpreter for format strings |
Scanner | Text scanner used to take user inputs |
Arrays | Provides methods for array manipulation |
LinkedList | Implements Doubly-linked list data structure |
HashMap | Implements Map data structure using Hash tables |
TreeMap | Implements Red-Black tree data structure |
Stack | Implements last-in-first-out (LIFO) data structure |
PriorityQueue | Implements unbounded priority queue data structure |
Date | Represents a specific instance of time |
Calendar | Provides methods to manipulate calendar fields |
Random | Used to generate a stream of pseudorandom numbers. |
StringTokenizer | Used to break a string into tokens |
Timer, TimerTask | Used by threads to schedule tasks |
UUID | Represents an immutable universally unique identifier |
java.util Package Enum
Enum Class | Description |
---|---|
Formatter.BigDecimalLayoutForm | Used for BigDecimal formatting |
Locale.Category | Used for locale categories |
Locale.FilteringMode | Provides constants for filtering mode selection |
Locale.IsoCountryCode | Used to specify the type defined in ISO 3166 |
java.util Package Exceptions
Exception Class | Description |
---|---|
ConcurrentModificationException | When an object is modified concurrently without permission |
EmptyStackException | Indicates that the Stack is empty |
InputMismatchException | When the user does not provide valid input |
MissingResourceException | Indicates absence of a resource |
NoSuchElementException | Indicates absence of requested element |
PatternSyntaxException | Indicates syntax error in a regular-expression pattern |
IllegalFormatException | Indicates that a format string contains illegal syntax |
Conclusion
- Util package in Java is a built-in package that contains several pre-written utility classes and interfaces.
- The import java.util.*; statement can be used to load the contents of the java.util package in a Java program.
- It consists of components such as data structures, exceptions, enumerations, utility classes, etc.
- It allows us to write clean and maintainable code.
- It contains the Collections Framework which is used to represent and manipulate collections.