Calculating The Exponential Value in Python
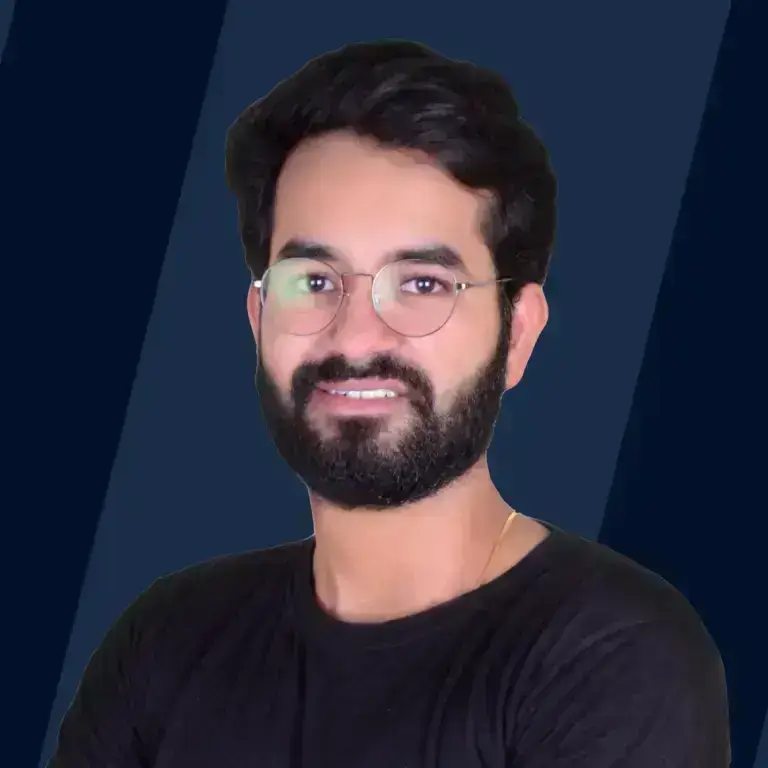
Overview
In this article, we will learn about calculating the exponential in python using different ways, but first, let’s understand its mathematical concept.
You may have seen exponential value in mathematics in the form of , having a simple meaning of multiplying A for x times.
Here, A is a base value, and x is an exponent value.
For eg.
In this example, 5 is a base value, and 3 is an exponent value, so we multiplied 5 for three times.
How to calculate The Exponential Value of a number in Python?
There are several methods to calculate the exponential in python. Here are three common approaches:
Exponential in Python Using Exponentiation operator (**)
In Python, we have an exponentiation operator, which is one of the ways to calculate the exponential value of the given base and exponent values.
We use the (**) double asterisk/exponentiation operator between the base and exponent values.
In the above example, we took base 2 and exponent as 16. Here, 2 gets multiplied 16 times.
It is the simplest method for calculating the exponential value in Python.
We can use floating-point values as well while calculating the exponential value in python.
Using exponentiations operator
When we use any operand as a floating-point number, we get the result in the floating-point number as shown in the following code snippet.
So these are some methods for calculating exponential values in Python. There are various pros and cons for the different methods explained above, so use them as per your requirements.
Exponential in Python Using pow() function
We have a huge variety of built-in functions in Python, and pow() is one of them, which helps us calculate the exponential value.
In the pow() function, we can pass the base and exponent values. These values can be of different data types, including integers, float, and complex.
pow(base, exponent) is equivalent to the base ** exponent.
But in this pow() function, three parameters are also allowed. The first two arguments are base and exponent, but we can give the third argument, which will calculate the modulus of the calculated exponential value.
The time complexity of calculating the exponential value by squaring is O(Log(exponent)).
Although Python doesn’t use the method of squaring but still shows complexity due to exponential increase with big values.
Let’s consider some of the examples with 3 parameters.
The first three examples have three arguments in the above examples, and the 4th only with two arguments.
It is advisable to use pow(5,3,2) instead of pow(5,3)%2 because the efficiency is more here to calculate the modulo of the exponential value.
We can perform these operations for negative numbers as well:
When using a negative number in the pow() function, we should take care of some things while using a negative number.
As the pow() function first converts its argument into float and then calculates the power, we see some return type differences.
Base | Exponent | Return Value |
---|---|---|
Non-Negative | Non-Negative | Integer |
Non-Negative | Negative | Float |
Negative | Non-Negative | Integer |
Negative | Negative | Float |
The pow() function can give the different errors in different situations, for, eg.
Exponential in Python Using math.pow() function
Apart from the built-in pow() function, we have the math.pow() function comes from the Python math module containing some useful mathematical functions.
This math.pow() function can also calculate the exponential value in Python.
The math.pow() shows the time complexity of O(1) due to the floating-point exponentiation, which is better than the built-in pow() function, But due to this, it sacrifices the precision of the result.
The math.pow() can take only two arguments, unlike the three arguments in the built-in pow() function.
The math.pow() function always returns a float value, whereas in the pow() function, we get int values most of the time.
These different techniques have different time complexities alongside their pros and cons.
Method | Time Complexity | Space Complexity |
---|---|---|
Operator (**) | O(Log(exponent)) | O(1) |
pow() function | O(Log(exponent)) | O(1) |
math.pow() function | O(1) | O(1) |
Conclusion
In this article, we saw the exponential values and how to calculate them using different techniques in Python.
- exponential value in python is the multiplication of base value exponent times.
- The exponentiation operator, which ** denotes, is one of the simplest ways to find the exponential value.
- The pow() is one of the inbuilt functions, which takes 2 to 3 arguments. It helps us to find the exponential value when two arguments are passed, and if we pass the third argument, then the modulus of the exponential value gets calculated.
- The math.pow() function comes from the math module, and it is the fastest way to calculate the exponential value with time complexity O(1).