Vector erase() in C++
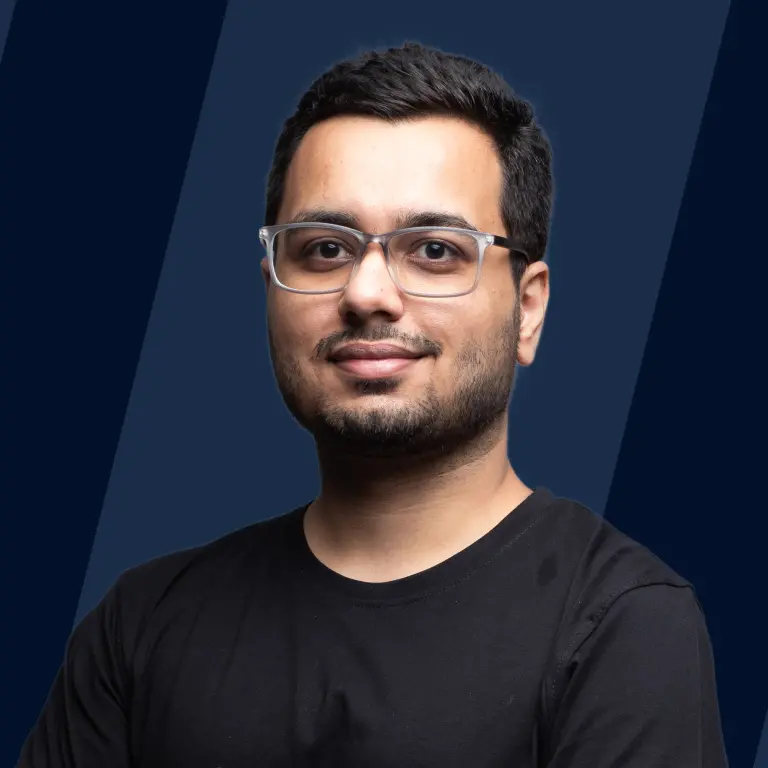
Overview
Vectors are similar to arrays but do not have a predefined size. Instead, they are dynamic in size, so we can insert and remove elements from them as we like. Hence, vectors provide all the ease of arrays while making everything dynamic. We have a predefined function called erase() to enable this dynamic property.
As the name suggests, vector erase() in C++ removes elements from the vector. It erases the elements at the position or positions given to it as parameters. Let us first examine its syntax to understand vector erase() better.
Syntax of Vector erase() in C++
Vector erase() function in C++ works in two syntaxes:
- One element is to be erased
- A range of elements are to be erased
For both of these ways, a different syntax should be followed depending on your need. Let us look at both of them one by one.
Syntax When One Element Is to Be Erased
When we want to remove a single element from somewhere in the vector, the syntax will be:
Here, vector_name is the name of the vector, and pos is the iterator that points to the element in the vector which is to be removed. This means we can remove any element from the vector, regardless of its index, given that we have its position as an iterator.
Let us look at the case when removing a range of elements.
Syntax When a Range of Elements Are to Be Erased
When we want to remove a range of consecutive or continuous elements of the vector in C++, the syntax will be:
Here, vector_name is the name of the vector, start_pos is the iterator that points to the first element of the range, which will be removed, and end_pos is also an iterator that points to the end of the range, only the elements before this position will be removed. Note that the element at start_pos will be removed, but the element at end_pos will not. Hence, the range given to this function is not inclusive.
Parameters of Vector erase() C++
The vector erase() function in C++ takes only vector iterators as parameters. Depending on the use case, if we want to remove a single element, there has to be a single parameter: an iterator pointing to the position of that element in the vector.
If a range of elements is to be removed, two parameters are required: an iterator pointing to the start of the range and another pointing to the end. The start pointer is inclusive, but the end pointer is not. Hence, elements starting from and including the start pointer up to the element before the end pointer will be removed in such a case, but the element at the end pointer will not be removed.
Return Value of C++ Vector erase()
The function vector erase() in C++ returns an iterator. This iterator points to the element which now occupies the position it erased. That means it points to the element after the element we deleted before executing the function. If we delete the vector's last element, then an iterator pointing to the vector end is returned.
For example, consider the vector
The vector initially has 6 elements. We will now erase the element '3' at index 2 using the vector erase() function.
After the function has been executed, the vector will look like
And the iterator it, which was returned from the vector erase() function, will be pointing to the element '4' at index 2, which was initiated after '3'.
Let us look at examples in C++ to understand this better.
Examples of Vector erase() in C++
In this section, we'll explore all kinds of examples to explain the different concepts of the vector erase() function in C++.
First, let us see the example of when we want to remove a single element from the vector.
One Element Is to Be Erased
Output
As you can see in the above code, the iterator was pointing to the word "hello", and after using the function vector erase(), it was removed from the vector.
Now let us look at the example when we have to erase a range of continuous elements from the vector in C++.
A Range of Elements Are to Be Erased
Output
In the above code, we erased a range of elements from the array. As you can notice, the end_pos iterator was not deleted—instead, only the elements starting from start_pos and before end_pos were removed from the vector. Hence, the vector erase() function in C++ is very helpful for deleting a range of elements from the vector without running a for loop.
Let us look at another example to see what the vector erase() function in C++ returns.
Return Value of Vector erase() C++
Output
As you can see in the above code, element 9 was erased from the vector, as the iterator pos was pointing to 9. When we checked the return value, it was found to be 10, the element after 9 in the original array. Hence, the vector erase() function in C++ returns an iterator pointing to the element after the one deleted in the initial array. If the last element is deleted, an iterator pointing to the end of the vector will be returned.
Errors and Exceptions of C++ Vector erase()
As we saw, the function vector erase() in C++ takes iterators as pointers in any case. Hence, if the iterators are out of bounds, they do not point to an element of the vector or are invalid, and the function shows undefined behavior.
Time Complexity of C++ Vector erase()
The time complexity for the vector erase C++ function is the sum of the deleted elements and the number of elements after the last erased element. Deleting each element contributes to the time complexity, and moving the elements left after deletion to occupy the empty space is another contributing factor.
Hence, if we are erasing only one element, the major time is from the number of elements after the element is erased. In the case of a range, the number of elements removed and the number of elements after the last element removed together form the total time complexity.
How Does C++ Vector erase() Work?
As you might be aware, vectors internally are dynamic arrays. This means that dynamic space is allotted in an array according to need. So when an element is inserted in the vector, a new space is allocated in the dynamic array. Similarly, when we want to erase one or more elements from the vector, it has to be removed from the dynamic array.
Hence, when we use the vector erase C++, first, the elements are removed from the dynamic array, and after that, the elements left after the deleted elements have to be relocated to take up the space. This also explains the time complexity described in the previous section for the vector erase C++ function.
Conclusion
- Vector erase C++ is used to erase elements at a particular position or positions given to it as parameters.
- Vector erase C++ function works in two syntaxes:
- One element is to be erased
- A range of elements are to be erased
- The vector erase C++ function in C++ takes only vector iterators as parameters. These iterators either point to the single element to be removed from the vector or to the start (inclusive) and end (not inclusive) elements of a range to be removed.
- The function vector erase C++ returns an iterator that points to the element which now occupies the position we erased, i.e., the element after the erased element.
- The time complexity for the vector erase C++ function is the sum of the deleted elements and the number of elements after the last erased element.
- When we use the vector erase C++ function, first, the elements are removed from the dynamic array, and after that, the elements left after the deleted elements have to be relocated to take up the empty space.