Vector Pair in C++
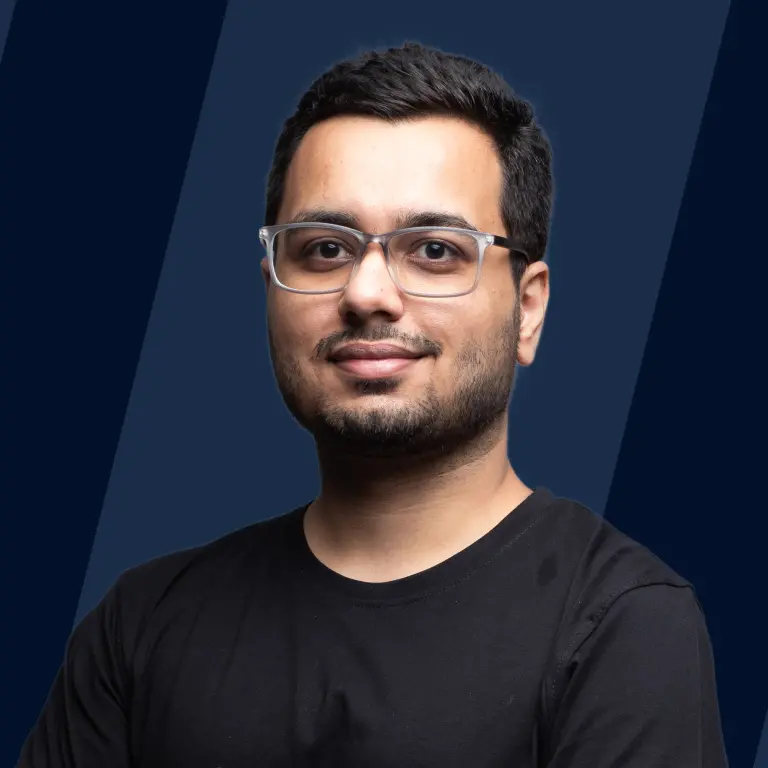
Overview
A vector in C++ is a dynamic array that can store a collection of elements of the same data type. It's a versatile data structure, often likened to a list or array in other programming languages. Vectors provide dynamic resizing, meaning they can grow or shrink as needed, making them handy for managing data. They offer efficient random access and insertion/removal at the end. Vectors are part of the C++ Standard Template Library (STL), providing a range of helpful functions and methods to manipulate data.
What is Vector Pair in C++?
Vector Pair is a powerful feature in C++ that allows programmers to work with pairs of elements stored within a vector container. It is particularly useful when you must group and perform various operations on two related pieces of data, like coordinates, keys, or values. In this article, we will delve into the syntax and usage of Vector Pair in C++, shedding light on its essential attributes.
Syntax
The syntax for declaring and using Vector Pair in C++ is straightforward. You must include the <vector> header to utilize the vector container and <utility> for pairs. Here's a basic outline:
Explanation:
- We include the necessary headers for vectors and pairs.
- Declare a vector vecPair that will store pairs of an integer and a double.
- Use push_back to add pairs to the vector. We create pairs using std::make_pair.
- Access individual elements of the pairs using the .first and .second member functions.
Vector Pair offers flexibility in choosing the types for the pair elements. You can use custom classes, structures, or standard data types per your requirements.
Memory management is crucial when dealing with vector pairs, particularly with large data sets. Vectors dynamically allocate memory, so it's essential to be mindful of memory usage to prevent inefficiencies and potential crashes. When working with large data sets, consider using reserve() to preallocate memory, reducing frequent reallocations and improving performance.
Modern C++ features like range-based for loops and lambda expressions enhance code readability. A range-based for loop simplifies iteration through elements, while lambdas facilitate custom operations on vector pairs.
Standard library algorithms like std::find, std::sort, and std::for_each provide efficient solutions for common tasks. For instance, std::find quickly locates elements, std::sort sorts pairs based on specified criteria, and std::for_each simplifies element-wise operations.
Example:
Applications:
Vector Pair is extensively employed in scenarios where you must maintain associations between two pieces of data. Some common applications include:
- Storing and managing key-value pairs in data structures.
- Implementing algorithms like Dijkstra's shortest path algorithm where nodes and distances are paired.
- Maintaining data in a sorted order based on one of the pair elements.
Understanding the syntax and usage of Vector Pair in C++ is essential for any programmer. This feature simplifies the management of paired data elements and opens up possibilities for various applications. By following the provided syntax and examples, you can harness the power of Vector Pair to enhance your C++ programming skills and efficiently handle related data pairs.
How to Use a Vector Pair in C++?
Vectors are versatile containers in C++ that can hold various data types. While vectors are powerful, combining them with pairs can unlock even greater functionality. In this guide, we will explore how to use vector pairs in C++ for various purposes, including accessing and modifying data, sorting and searching elements, and employing efficient iteration techniques.
Access and Modification
Accessing and modifying elements within a vector pair is straightforward. First, let's create a vector of pairs:
In this example, we create a vector of pairs, allowing us to store both an integer and a string for each student. We then access and modify the second element of the first pair to change Alice's name to Alicia.
Sorting and Searching
Sorting and searching within a vector pair can be accomplished using standard C++ functions or custom comparators. Here's how to sort a vector pair based on the first element (integer in our case):
Iteration Techniques
Iterating through a vector pair is similar to iterating through a regular vector. You can use range-based for loop or iterator to traverse the elements:
In this guide, we've explored how to use vector pairs in C++ for various essential tasks, such as accessing, modifying, sorting, searching, and iterating. By harnessing the power of vector pairs, you can efficiently manage and manipulate paired data in your C++ programs.
Examples
Let us now see several examples of vector pairs, explore their applications, and demonstrate how they can be leveraged in real-world scenarios.
Basic Usage of Vector Pair
A vector pair, also known as a std::vector<std::pair<T1, T2>>, is a container that stores pairs of elements. These elements can be of different types (T1 and T2). Let's start with a basic example:
In this example, we create a vector pair to store student IDs (int) and their corresponding names (string). We use push_back to add elements and then iterate through the vector to print the pairs.
Sorting Vector Pair
Vector pairs can be sorted based on each pair's first or second element. Here's an example of sorting based on the first element (student ID):
This code sorts the vector pair based on student IDs in ascending order.
Searching in Vector Pair
You can perform searches in vector pairs using algorithms like std::find:
Explanation:
This code demonstrates searching for a specific student ID in the vector pair.
Vector pairs in C++ are incredibly useful for storing and manipulating pairs of related data. They are versatile containers that can be sorted, searched, and customized to suit various applications. Whether you're managing student records, employee details, or any other related data, vector pairs offer a straightforward and efficient solution in C++ programming.
Conclusion
- Vector Pair in C++ provides a flexible and dynamic way to manage pairs of data elements. This dynamic nature allows programmers to add or remove pairs as needed, making it a versatile data structure.
- When searching for specific pairs within a Vector Pair, C++ offers efficient algorithms to find elements quickly. This is particularly useful when working with large datasets, as it helps optimize performance.
- Unlike other data structures, Vector Pair in C++ maintains the order of elements as they are added. This property simplifies data processing tasks that rely on maintaining a specific order, such as sorting or filtering.
- Vector Pair is valuable in various scenarios, such as creating dictionaries, storing key-value pairs, or managing related data points in a structured manner. Its adaptability makes it a popular choice among C++ developers.
- C++'s Vector Pair is straightforward to implement and work with, even for programmers who are relatively new to the language. Its simplicity and clarity make it an excellent choice for projects where ease of use is a priority.