What is Virtual Destructor in C++?
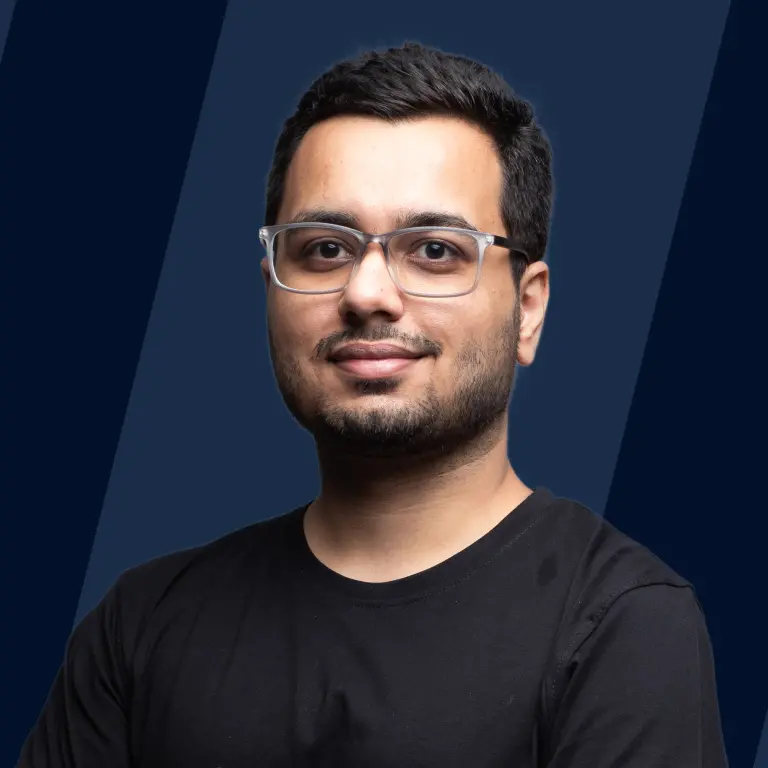
Before we learn about Virtual Destructor in C++, let's learn a bit about Destructor in C++.
A destructor in C++ is a class member function that is implicitly invoked when the scope of a class object ends. Same as a constructor, which is also invoked implicitly when an object of a class is created. A destructor is used to free up the resources occupied by an object. The destructor function has the same name as that of a class, but a tilde (~) sign is used before writing the function's name.
Now, Let's learn about Virtual Destructor in C++.
Virtual Destructor in C++ is used to release or free the memory used by the child class (derived class) object when the child class object is removed from the memory using the parent class's pointer object.
Virtual destructors maintain the hierarchy of calling destructors from child class to parent class as the virtual keyword used in the destructor follows the concept of late binding or the run-time binding.
The destructor of the parent class uses a virtual keyword before its name and makes itself a virtual destructor to ensure that the destructor of both the parent class and child class should be called at the run time. The derived class's destructor is called first, and then the parent class or base class releases the memory occupied by both destructors.
Why We Use Virtual Destructor in C++?
As we know, a destructor is implicitly invoked when an object of a class goes out of scope or the object's scope ends to free up the memory occupied by that object.
Due to early binding, when the object pointer of the parent class is deleted, which was pointing to the object of the derived class then, only the destructor of the parent class is invoked; it does not invoke the destructor of the child class, which leads to the problem of memory leak in our program.
So, When we use a Virtual destructor, i.e., a virtual keyword preceded by a tilde(~) sign and destructor name, inside the parent class, it ensures that first the child class's destructor should be invoked. And then, the destructor of the parent class is called so that it releases the memory occupied by both destructors.
Note: There is no concept of virtual constructors in C++.
Example
Let's look at some examples to understand the working of virtual destructor in C++.
Example 1: Delete Child Object Using Base Class Pointer Without a Virtual Destructor
Code:
Output:
Example Explanation:
In the above program, we have used one parent class (Base) and a derived class (Child), inside which both constructors and destructors are defined in both classes.
Deleting an object of a derived class using a pointer of the parent class shows an undefined behavior because it does not have a virtual destructor. So, when we delete the object of the Child class to release the space occupied, it invokes the base class's destructor, but the Child class's destructor is not invoked. As seen in the output, the destructor of the Child class is not invoked because the base class pointer can only remove the base class's destructor, which causes the problem of memory leak in the program.
Let's see another example that overcomes the memory leak problem using a virtual destructor.
Example 2: Delete Child Object Using a Base Class Pointer with a Virtual Destructor
Code:
Output:
Example Explanation:
In the above program, a virtual destructor is used in the base class to call the child class's destructor before the base class's destructor is invoked. This releases the memory occupied and also resolves the problem of memory leaks.
How Does Virtual Destructor Work?
Virtual destructor works in a way that ensures the correct order of invoking destructors when the object of the derived class goes out of scope or is deleted. If the order of invoking is incorrect, it will lead to a problem known as a memory leak.
Virtual destructor in C++ is mainly responsible for resolving the problem of memory leaks.
When we use a virtual destructor inside the base class, it will call the destructor of the child class, ensuring that the child class's object should be deleted so there might be no memory leakage.
Note: The derived class's destructor is invoked indirectly using the vptr(Virtual Pointer Table).
Verifying Compiler Augmented Code in Case of the Virtual Destructor
The Augmented code of the compiler can not be seen, but we can use a trick to prove that when we use a virtual destructor, the compiler augments the call of the base class' destructor in a derived class.
Let's see an example to verify the above statement:
Example
Output:
-
When we use a pure virtual function(), the compiler will throw an error at the time of linking.
-
As seen in the above output, the compiler tried to augment the call of the base class destructor into the derived class destructor. But due to the base class's destructor being unavailable, the linker exited with an error.
Pure Virtual Destructors in C++
When we initialize a virtual destructor with 0 and don't implement it in the class, it becomes a Pure Virtual Destructor in C++. Once a destructor is declared as pure virtual, it must be implemented. This is against the pure virtual behavior in C++.
Legally we can use a pure virtual destructor in C++. It is important to note that if there is a pure virtual destructor inside the class, then a pure virtual destructor must have a functional body.
A pure virtual destructor makes a class Abstract; therefore, creating objects of that class is impossible.
Why Does a Pure Virtual Destructor Require a Function Body?
A pure virtual destructor must have a function body, as we know that the destructor of the child class will be invoked first before invoking the base class destructor. Therefore, if a function body is not provided, nothing will be called during the object's destruction, and an error will be thrown.
Example
Let's see an example to understand the requirement of a function body for pure virtual function:
Code:
Output:
In the above code, if we don't implement the base class pure virtual constructor outside the class, then the code will through an error when the derived class destructor calls the base class destructor.
Learn more
- To explore more about Constructor and Destructor, refer to Constructor and Destructor in C++
- To explore more about Copy Constructor, refer to Copy Constructor in C++
- To explore more about Inheritance in C++ refer to Inheritance in C++
- To explore more about Abstract Class in C++ refer to Abstract Class in C++
Conclusion
- Destructor in C++ is a member function of the class that is implicitly invoked when the scope of an object of the class ends.
- Virtual Destructor in C++ is a member function that frees up the memory allocated by the object of a child class or derived class when it is removed from the memory using the parent class pointer object.
- Virtual destructor in C++ is mainly responsible for resolving the memory leak problem.
- When we made a virtual destructor pure virtual destructor, it became mandatory to implement that destructor.