What is a Void Pointer in C++?
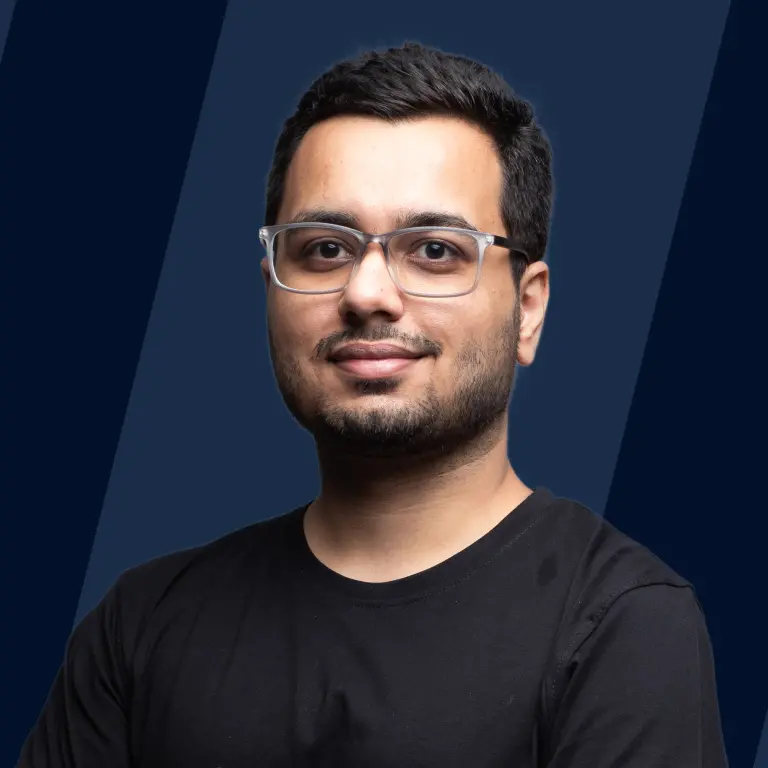
A void pointer in C++ is a special pointer that can point to objects of any data type. In other words, a void pointer is a general purpose pointer that can store the address of any data type and it can be typecasted to any type. A void pointer is not associated with any particular data type.
The size of a void pointer is different in different systems. In 16-bit systems, the size of a void pointer is 2 bytes. In a 32-bit system, the size of a void pointer is 4 bytes. And, in a 64-bit system, the size of a void pointer is 8 bytes.
Syntax
In C++, we use the void keyword to declare a void pointer.
Example
In the above example, we used a void pointer to store the address of an integer variable and then used the same pointer to store the address of a character variable.
Advantages of using Void Pointer
Following are the advantages of a void pointer :
- The calloc() and malloc() functions return void* type (void pointers). This allows us to use these methods to allocate memory of any data type of our choice.
- In the C programming language, void pointers are used to implement generic functions. For example, the qsort() function in the standard C library .
How to Use Void Pointer in C++?
Example 1 – Void Pointer for int, float, and String
In C++, a void pointer is a type of pointer that can point to any data type, be it an integer, float, or even a string. Below is an example that demonstrates how to use a void pointer with different data types:
Output:
In this example, a void pointer is used to point to an integer, float, and string. The printData function takes a void pointer and an enum class DataType as parameters. Inside the function, the void pointer is explicitly converted to the appropriate data type using static_cast, and the data is printed. The main function demonstrates how to call the printData function with different data types.
Example : 2 – Trying to Convert the Void Pointer to Constant
C++ does not allow us to convert void pointers to constants. Let us see what happens when we try to do so.
Output :
In the above example, we were able to initialize the void pointer to both int and char pointers. However, when we tried to initialize the void pointer to a constant, we got an error.
Example : 3 – Pointer to Void
We can store the address of a variable in a void pointer.
Output :
In the above example, we declared a void pointer variable and a float variable. Then, we stored the address of the float variable in the void pointer variable.
Example : 4 – Memory allocation with malloc method
The malloc() method returns a void pointer if the memory allocation is successful. We can then type cast this void pointer into different data types.
Output :
In the above example, we created an integer pointer ptr. We used the malloc() method to allocate memory to ptr that was equivalent to the size of 10 integers. Since malloc() returns a void* type, we typecasted the void pointer to an integer pointer. Finally, we used this pointer ptr to assign (and then print) values to the allocated memory.
Example - 5 : Printing the Content of Void Pointer
We can use static_cast to print the data present in a void pointer. static_cast converts the pointer of the void* data type to the data type of that variable whose address is stored by the void pointer.
Output :
In the above example, we used static_cast to convert the void pointer into an int pointer, so that we could print the contents of the variable var.
C-Style Casting
To print the values stored in a void pointer, we can use the C-style casting.
For Example :
Here ptr is a void pointer that contains the address of an int variable.
Although C-style casting can be used with void pointers, using static_cast (explained in Example 5 above) is the preferred method for casting. This is so because static_cast is checked by the compiler so that the cast could never fail at the runtime. On the other hand, the C-style casts are not checked by the compiler, and hence, have a chance of failing at the runtime.
Void pointer miscellany
-
We can set void pointers to null values.
The pointer ptr is a void pointer and we have set it to be a null pointer.
-
We can not perform pointer arithmetic on a void pointer. This is so because, to increase or decrease the pointer's value, pointer arithmetic needs the pointer to know the size of the object it points to.
-
We should avoid deleting a void pointer if a void pointer points to dynamically allocated memory . This can lead to undefined behavior of the program.
-
Void pointers can not be dereferenced. This is so because the void pointer has no associated data type with it. So, there is no way for the compiler to know what type of data is pointed by the void pointer.
-
We can not create void references because a void reference would be of the type void&, and we would not know what type of value the pointer referenced.
Difference Between a Void Pointer in C and C++
The C programming language allows us to assign a void pointer to any other pointer type without any typecasting. On the other hand, in the C++ programming language, we must typecast a void pointer when we have to assign the void pointer to any other pointer type.
Let us look at an example to understand this concept.
Assigning a void pointer to a pointer of another type in C :
Output :
In the above example, we were able to assign the void pointer void_ptr to an integer pointer int_ptr without using typecasting.
Assigning a void pointer to a pointer of another type in C++ :
Output :
In the above example, we assigned the void pointer void_ptr to the integer pointer int_ptr by using typecasting. Had we not done that, we would have got an invalid conversion from 'void' to 'int' error.
Learn More
Conclusion
- A void pointer is a special pointer that can point to objects of any given data type.
- A void pointer can be converted into a pointer of another data type by using either C-style casting or static cast.
- We can not convert void pointers into constants.
- The malloc() function is used to allocate memory to a void pointer.
- Void pointers can be set to null values.
- We can not perform pointer arithmetic on void pointers.
- Void pointers can not be dereferenced.
- We can not create void references.