Void Pointer in C
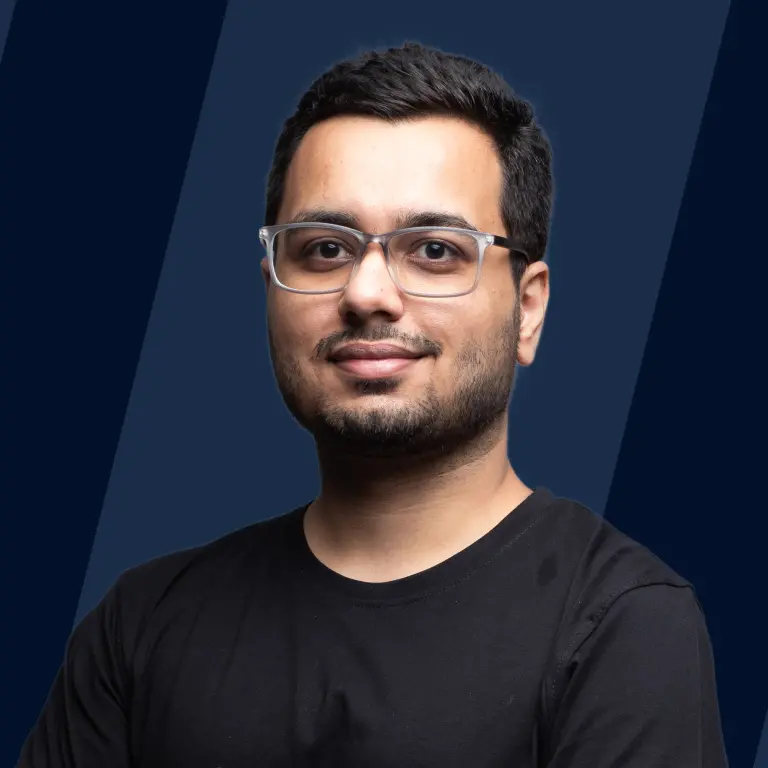
We all know that pointers in the C language are a special kind of variable that stores the addresses of other variables, which means to store the address of an int variable, we will need an int pointer, to store the address of a float we will be needing a float pointer, to store the address of a char variable, we will be needing a char pointer and so on.
That means the addresses stored in the pointer variable must be of the same type as specified with the pointer at the time of pointer declaration. This means if we declare an int pointer then this pointer cannot point to, or store the address of floating-point or char variables, it can store the address of an integer variable only
The concept of void pointer here is, we declare a pointer to point to void, meaning a generic pointer, a pointer that has no data type associated with its own, which can point to any data type and can store any datatype's address as will be required
In simple words, We can assign the address of any data type to a void pointer, and can later mold it as per our requirement
Syntax
Let's understand this better with the below examples
Example of Void Pointer
We know that a void pointer can store the address of any data type, but not only that, a void pointer can be assigned to any other type of pointer as well without any explicit typecasting
Examples:
Let's understand it properly with code
Output:
From the above program, we can see that void pointers are not associated with any specific datatype at the time of their declaration, but we can initialize, and re-assign them to any of the available data types as per our requirement
Size of the Void Pointer in C
The size of void pointers varies from system to system. For 32-bit systems, the size of a void pointer is 4 bytes, and if the system is 64-bit, then the size of the void pointer will be 8 bytes.
let's see this with the code
Output:
Note - On my system the size is coming as 4, it may differ on your system
The size of any type of pointer is almost the same, as, in the end, they all are just storing the memory addresses, which is almost constant in the number of bits for any particular system
We can verify it as
Output:
How Does Void Pointer Work in C?
As we have understood till now, a void pointer does not have any associated data type of its own and is capable of storing the address of any type
We should also know that void pointers can be typecasted into any type of pointer very easily and dynamic memory allocation also becomes convenient using void pointers.
In C language functions like malloc(), calloc() etc. are used to dynamically allocate the memory, they return void pointers, which we can then typecast into any other type of pointer as per our requirement, and can easily start using them
In simple words, any pointer which is declared with the keyword void is a void pointer in C language, and how it works is, that we can assign any type of address into that pointer and then later can typecast it into the appropriate data type and can use it
Now, why typecast?
Because a void pointer cannot be dereferenced, and as we know to get a value through a pointer, we first need to dereference it using the * operator, but void pointers cannot be dereferenced, as they are the generic pointers, the compiler in advance will not be able to get what type of variable is the void pointer pointing to, because there is no presence of a datatype associated to it at the time of its declaration, meaning compiler will not have any appropriate source to get an idea of what type of datatype the pointer is pointing to, so dereferencing it right away will result into an error.
This is why we first need to typecast a void pointer to the datatype of our requirement, and after that, we can dereference it and access its respective value
More Examples of Void Pointer in C
Example 1:
Output:
Example 2:
Output:
Example 3:
Output:
Example 4:
Output:
Advantages of Void Pointer
-
There are times, when we are not sure, what type of memory address a pointer variable will store, then it is best to declare that as a void pointer, and later we would typecast it as per our requirement
-
Another advantage is that dynamic memory allocation functions like malloc(), and calloc() returns a void pointer, which means we can use these functions to allocate memory dynamically to any data type, whatever the case is, they will be returning only a void pointer, and we can easily typecast it later as per our need
Important Facts Related to Void Pointer
1. Differencing a Void Pointer in C
As discussed above, we cannot directly difference void pointers, instead, we first need to typecast it into the appropriate data type then it will be possible to dereference it
Example:
Output:
Instead, we should write the above code as
Output:
2. Arithmetic Operation on Void Pointers
We cannot directly apply arithmetic operations on the void pointers, we need to typecast them first to apply the pointer arithmetic
Example:
Output:
Instead, we should write the above code as
Output:
Why Use Void Pointer in C?
- Void pointers can be used as a generic pointer, to store the address of different data types at different times
- Void pointers are helpful in dynamic memory allocations, as functions like malloc() and calloc() return a void pointer, which can easily be typecasted into any type of pointer, meaning with the help of void pointers, it is made possible for the functions like malloc() and calloc() to allocate memory to any datatype without doing any additional typecasting, because they can simply always written a void pointer, and then the user can typecast it as per their requirement
Limitations of Using Void Pointers in C
- We cannot dereference void pointers directly to access the values stored at those addresses
- Pointer arithmetic on void pointers is not possible directly, we first need to typecast it into an appropriate data type, and after that only, we will be able to apply basic arithmetic operations on it
To learn more about Pointers in C, visit here
Conclusion
- Void pointers in C are a special type of pointers that do not have any data type associated with them, they are generic pointers and can be used to store the address of any datatype
- Syntax : void *pointerName;
- The size of void pointers varies from machine to machine, but in general they are 4 bytes in 32-bit systems and 8 bytes in 64-bit systems
- Void pointers can not be dereferenced directly, they first are needed to be typecasted into an appropriate data type, after they can be dereferenced
- We can not apply pointer arithmetic directly on void pointers, they first must be typecasted into an appropriate datatype
- Void pointers allow functions like malloc(), and calloc() to dynamically allocate memory to any data type, as they always return a void pointer, which can be easily typecasted into the required datatype