Volatile In C
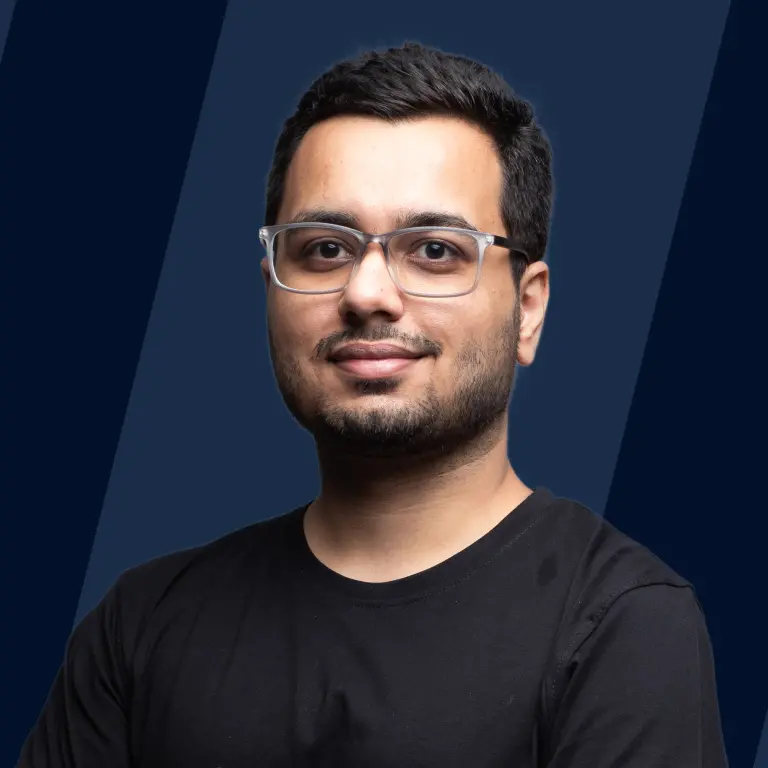
Overview
The volatile keyword In C Language indicates to the compiler that the value of a variable can change at any point without any action being performed by the code found nearby. It prevents some optimizations from causing unexpected behaviour in programs that rely on the variable's value. Essentially, it instructs the compiler to retrieve the variable's value from memory each time it is referred, ensuring that real-time modifications are taken into account. This is especially important in situations where variables may be updated by external sources such as hardware registers or concurrent processes.
What is a Volatile Keyword in C Language?
In C Language, the volatile keyword is a subtle yet powerful technique that is critical in maintaining trustworthy communication between a programme and external entities. Variables are commonly kept in registers or memory In C Language programming, and the compiler optimizes code assuming that variable values do not change unexpectedly. This assumption, however, might have unforeseen effects in particular cases involving external hardware, interrupts, or shared data in a multi-threaded system.
The volatile keyword informs the compiler that the variable can be changed at any moment, outside of the program's normal flow. It prohibits the compiler from assuming the variable's value, guaranteeing that every access to the variable happens in an actual read or write operation.
Consider it a signal to the compiler that says, "Hey, this variable is a bit unpredictable, so don't get too comfortable with your optimizations". This is especially important when working with memory-mapped hardware registers, interrupt service routines or any other circumstance in which the variable's value might change asynchronously to programme execution. In essence, volatile maintains communication channels open, allowing your code to adapt to the dynamic nature of certain program settings smoothly.
Syntax of Volatile Keyword in C Language
The volatile keyword In C Language is critical for ensuring that the compiler does not perform unnecessary optimizations, especially when dealing with variables that can be updated outside of the program's flow, such as hardware registers or shared variables in a multi-threaded environment.
Declaring a variable as 'volatile' In C Language syntax indicates to the compiler that the variable's value may change at any moment without any action being performed by the code found nearby. This stops the compiler from making assumptions about the stability of the variable and, as a result, optimizes away what it considers superfluous reads or writes.
Here's a simple declaration example:
This line informs the compiler that sensorValue might be changed at any time, possibly by an interrupt service procedure or external hardware. As a consequence, the compiler assures that each access to'sensorValue' corresponds to a valid read or write operation, avoiding unexpected behaviour caused by optimizations.
Understanding the volatile keyword is critical in situations where variables might change asynchronously to the programme flow, ensuring that your C code operates predictably and consistently in dynamic contexts.
How does Volatile Keyword in C Language Work?
The volatile keyword is small but significant In C Language programming language. It can be considered as a flag that instructs the compiler to handle a variable differently. When a variable is designated as "volatile", the compiler knows that its value might change at any time—outside of the program's normal flow.
Here's the trick: Without the volatile keyword, the compiler may optimize code based on the assumption that a variable remains constant unless changed within the code. In the actual world, however, variables might change owing to external sources such as hardware interrupts or other threads.
The volatile keyword serves as a reminder to the compiler: "Hey, don't make assumptions about this variable's value because it might change unexpectedly". It stops the compiler from using certain optimizations, guaranteeing that the variable is always read from memory rather than cached.
Using the volatile keyword notifies the compiler to be attentive and react dynamically to the program's unanticipated change, whether dealing with hardware registers, multithreading, or instances where a variable could change outside of the program's control. It's a basic keyword, but it's effective in keeping our code in sync with the unpredictable nature of the programming.
Examples
Consider the following scenario: You're working with a microcontroller that handles sensor inputs. These sensors may be recording real-time data, and the readings may vary owing to external circumstances outside the control of your program. In such instances, you might specify the variables involved as 'volatile.'
In this case, the variable sensorValue is declared volatile to inform the compiler that its value may change asynchronously. The compiler is not allowed to optimize or cache the variable, ensuring that each access obtains the most current value.
The volatile keyword signals the compiler to be careful and avoid making assumptions about variable stability. By using the volatile keyword carefully, you may improve the stability of your C programmes, especially when variables are subject to external changes outside of the usual programme flow.
Advantages of Using Volatile Keywords in C Language
The volatile keyword In C Language may appear trivial, yet it has a significant influence on programme behaviour. Consider it a superhero cape for variables, signalling that their values might change suddenly. This keyword is game-changing in situations where variables can be changed by external sources such as hardware interrupts or concurrent threads.
In embedded systems, one advantage of using the volatile keyword is obvious. Hardware interactions can quickly modify variable values in this domain, and the compiler's optimization may ignore these changes. By marking a variable as 'volatile', we effectively instruct the compiler to retrieve its value from memory each time it is requested, eliminating any accidental caching that may result in unpredictable behaviour.
Moreover, 'volatile' demonstrates its worth in multithreading situations. Because of caching, changes to variables in one thread may not be instantly reflected in another thread in a multithreaded program. The use of 'volatile' guarantees that the most recent value is always obtained, improving data consistency across threads.
The volatile keyword effectively helps functions to get data integrity and hence it guarantees that your variables retain their proper values despite the unexpected changes in the running processes.
Disadvantages of Using Volatile Keywords in C Language
The volatile Keyword In C Language provides real-time benefits but also has downsides. This term advises the compiler that the value of a variable can change at any point, not merely as a result of the logic of the program. While this is extremely useful for performing hardware-related activities and assuring correct data in embedded systems, it does have certain drawbacks.
For starters, the term "volatile" can impede compiler optimization. Because the compiler considers that the variable may change outside, it refrains from doing some optimisations, reducing the overall efficiency of the programme.
Furthermore, debugging might become more difficult. Traditional debugging tools are predicated on the premise that variables change while the program runs. Variables that are "volatile" may change suddenly, making it difficult to track down the source of an issue.
Furthermore, usage of the term "volatile" may result in less maintainable code. As the rules governing variable alterations loosen, it becomes critical to use them wisely to avoid unwanted problems.
While the volatile keyword might be useful in some cases, its excessive use may negatively impact code efficiency, debugging efforts, and overall code maintainability. It's required that developers must carefully consider its usage as per the specific needs of their project.
FAQs
Q. In what situations should I utilize the volatile keyword in my code?
A. When dealing with variables that can be changed outside of the regular flow of control, such as by an interrupt service procedure, the volatile keyword is used. Declaring a variable volatile informs the compiler that its value can change at any time, prohibiting certain optimizations that could result in improper behaviour.
Q. In C Language, can I use the volatile keyword with any type of variable?
A. The volatile keyword can be used on any sort of variable, whether it be a basic integer, a floating-point number, or a more complicated data structure. Its goal is the same across data types: to ensure that the compiler does not make assumptions about the variable's value at any stage.
Q. What effect does the volatile keyword have on multithreaded programming?
A. The volatile keyword is very important in multithreaded programming. When many threads access a shared variable, the compiler may optimize code as if it were static, resulting in unexpected behaviour. The compiler is informed when a variable is marked as volatile that the variable can be affected by external influences, prohibiting certain optimizations and guaranteeing the variable is always read from and written to memory.
Q. Can you use the volatile keyword in C Language with const variables?
A. The volatile and const keywords are not mutually exclusive. You may combine them to get a variable that is both constant and volatile. This combination is especially beneficial when a variable should not be updated within the program but can be changed by external sources such as hardware registers.
Q. Is thread-safety guaranteed by the volatile keyword in C Language?
A. It is important to recognize that, while the volatile keyword is useful in some multithreading circumstances, it does not provide a complete answer for thread safety. Volatile guarantees that the variable is not optimized in such a way that it may result in unexpected behaviour due to changes outside of the programme flow. Additional synchronization techniques, such as locks or mutexes, are frequently necessary for strong thread safety.
Q. Is it possible to utilize the volatile keyword In C Language with static variables?
A. The volatile keyword may be used with static variables. The usage of the volatile keyword is unaffected by whether a variable is static or not. If a static variable is likely to change outside of the usual programme flow, utilizing volatile is a wise decision to avoid compiler optimizations that might damage the variable's integrity.
Conclusion
- The volatile keyword works as a monitor in multi-threaded or embedded systems, alerting the compiler to fetch the variable's value from memory each time it is accessed. This prevents unexpected optimizations from damaging data integrity.
- When working with interrupt-driven applications, employing volatile guarantees that variables shared by the main programme and ISRs are updated correctly. This is critical to avoiding data discrepancies in time-critical processes.
- Compilers may optimize code without volatility by assuming a variable's value remains constant between accesses. Using the volatile keyword explicitly instructs the compiler to fetch the variable from memory each time, reducing unwanted optimizations.
- Hardware registers are frequently changed asynchronously in embedded system programming. When interacting with these registers, using volatile guarantees that the compiler retrieves the most recent values, eliminating any synchronization difficulties.
- When variables are shared between threads, volatile helps with synchronization. It prevents cached values from being used in various threads, ensuring that all threads use the most up-to-date information.