C++ Program to Check Whether a Character is Vowel or Consonant
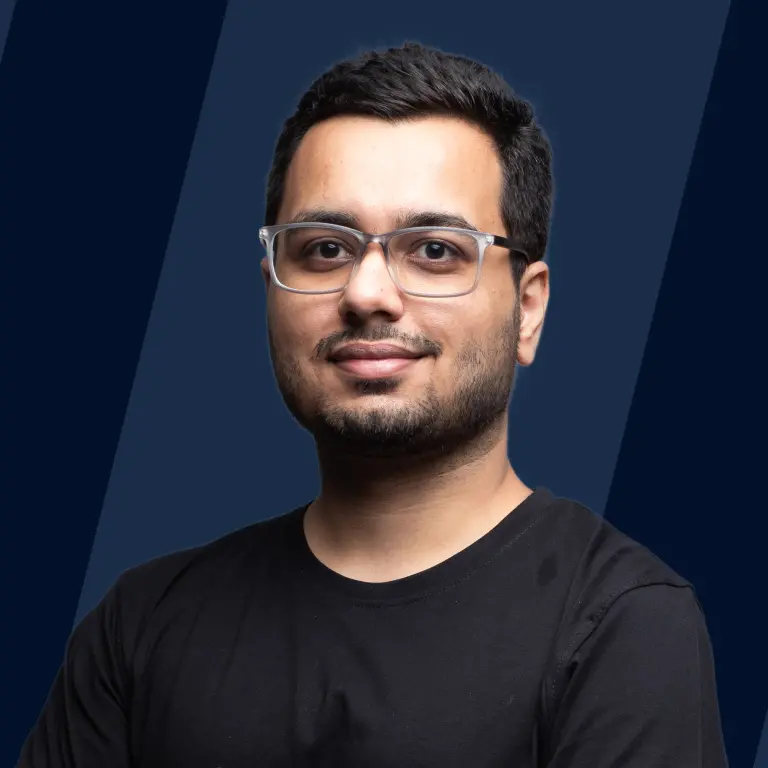
Overview
In the 26 letters of the English alphabet, there are five vowels: A, E, I, O, U, or a, e, i, o, u. Based on these five vowels, a C++ program can be created to determine whether input consists of vowels at runtime. The C++ program can be created using multiple ways like if-else, if-else ladder, and switch-case where the output console will print “Vowel” if the character entered by the user is the vowel and for consonants, the output console will print “Consonants”.
Introduction
We all know that there are 26 alphabets in the English language out of which 5 alphabets A,E,I,O, and U are vowels and all the other alphabets are called consonants. Therefore to identify this programmatically we are creating a C++ program that will check if the input alphabet entered by the user at runtime is consonant or vowel. The output console will print “Vowel” if the character entered by the user is the vowel and for consonants, the output console will print "Consonants".
There are multiple ways by which we can write this program, out of which a few are listed below -
- Using if-Else (Manually)
- Using If-Else Ladder
- Using Switch Case
Let's take a closer look at each type of program in brief.
1. C++ Program to Determine Whether an Alphabet Character is a Consonant or a Vowel Using If-Else(Manually)
Syntax
The Syntax to determine whether an alphabet character is a consonant or a vowel using IF-Else is -
Working
Here is a Flow Diagram showing how the program works -
Example
In this program, we are aiming to identify whether a character entered by a user during runtime is consonant or vowel by using an if-else statement in C++.
Let's take a look at the complete C++ program -
The Output of the above C++ program is -
Explanation
The above code is mainly divided into two parts. The first part is to accept user input in the form of characters. Now, in the second part of the code, the input alphabet is checked in an If-Else condition to determine whether the character contains any vowel of lower-case and upper-case. If the If condition is true then output Vowel will be printed else Consonant will be printed on the console as an Output.
2. C++ Program to Determine Whether an Alphabet Character is a Consonant or a Vowel Using If-Else Ladder.
Syntax
The Syntax to Check Whether a Character is Vowel or Consonant using IF-Else Ladder is -
Working
Here is a Flow Diagram showing how the program works -
Example
In this C++ program, we are aiming to identify whether a character entered by a user during runtime is consonant or vowel by using the if-else ladder. In this if-else ladder, each if and else-if statement has a conditional check for each vowel separately.
Let's take a look at the complete C++ code -
The Output of the above C++ program is -
Explanation
As the name suggests, an if-else ladder is a sequence of if statements that follow one another. In the above code first, we take character input from the user. This input is passed through the if-else ladder where in the sequence of if-else statements each if statement checks for one vowel in lower-case and upper-case. Since there are 5 vowels therefore in the program we have 5 conditions to check each vowel. If one of the If conditions is true then output Vowel will be printed else Consonant will be printed on the console as an Output.
3. C++ Program to Determine Whether an Alphabet Character is a Consonant or a Vowel Using Switch-Case.
Syntax
The Syntax to Check Whether a Character is a Vowel or Consonant using Switch-Case is -
Working
Here is a Flow Diagram showing how the program works -
Example
In this C++ program, we are aiming to identify whether a character entered by a user during runtime is consonant or vowel by using the switch-case. Here, each case statement has a conditional check for each vowel separately.
Let's have a look at the complete C++ code -
The Output of the above C++ program is -
Explanation
In the above code first, we take character input from the user. Using switch case the input is tested against the different cases for each vowel in upper-case and lower-case. If any of the case value matches with the input then Vowel will be printed else the default case will execute printing Consonant on the console as an Output.
4. Program to Check When a Character is Neither Vowel nor Consonant (when a Non-alphabet Character is Entered. Like ‘7’ or ‘[‘ etc)
Example
Sometimes we can have some different input than alphabets like numbers or symbols from the user but in the previous examples, we have not written any logic to handle input other than vowels and consonants. Therefore in this program, we are aiming to handle the character input if it is neither consonant nor vowel.
Let's take a look at the complete C++ code -
The Output of the above C++ program is
Explanation -
In the above code first, we take character input from the user. The user input is checked using the if condition if it is an upper-case or lower-case alphabet or not. If the output comes false then "neither vowel nor consonant" is printed on the console. If it is true then the conditional expression for the vowel is checked using the if-else statement. If the If condition is true then output Vowel will be printed else Consonant will be printed on the console as an Output.
Conclusion
Let's recall what we have learned in this article,
- Based on the 5 vowels in the English alphabet there are multiple ways to create a C++ Program to determine whether an alphabet character is a consonant or a vowel.
- Some of the ways by which the C++ program can be created are if-else, if-else ladder, and switch-case.
- While using an if-else ladder, each if and else-if statement has a conditional check for each vowel separately.
- In the switch-case approach to solve this program, each case statement has a conditional check for each vowel separately.
- Sometimes we can have some different input than alphabets like numbers or symbols from the user therefore to handle this we've written a program where user input is checked if it is an alphabet or not using the if-else statement.