C Program to Check Whether a Character is a Vowel or Consonant
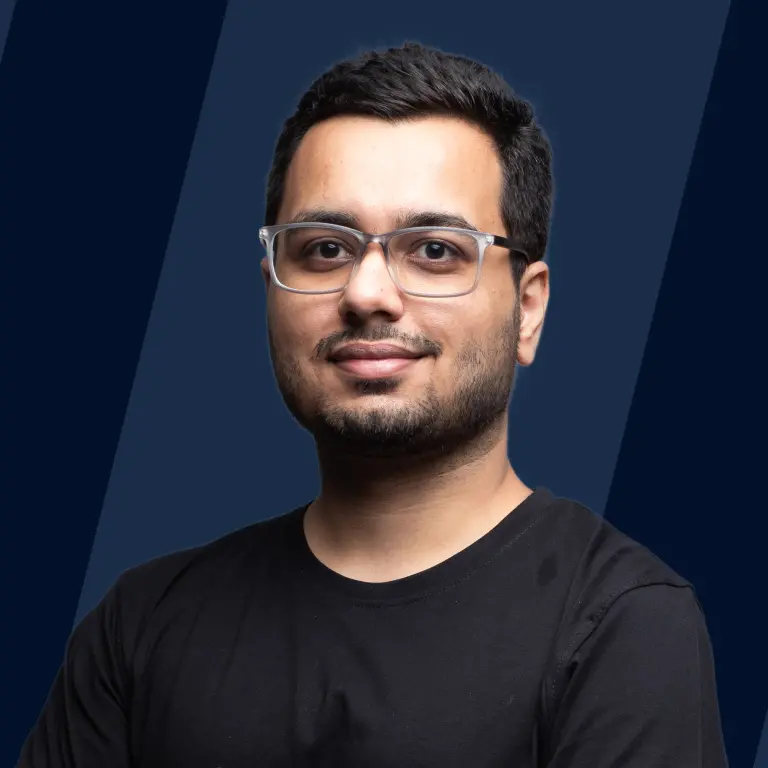
There are 5 vowels in the English language. These are a, e, i, o, and u. We can use the C programming language to check if a given letter is a consonant or a vowel. This can be done using an if statement, a switch statement, a function, or the ASCII values of the vowels and consonants.
What are Vowels and Consonants?
Of the 26 letters in the alphabet, 5 are vowels, and the remaining 21 letters are consonants. The five vowels in the English language are:
- a (or A)
- e (or E)
- i (or I)
- o (or O)
- u (or U)
C Program to Check Vowel or Consonant Using if else
We can use the if-else statement to check if a letter is a vowel or consonant. The vowel or consonant program in C uses the if-else statement.
Code :
Output :
In the above program, we used the if statement to check whether the given letters were either 'a', 'A', 'e', 'E', 'i', 'I', 'o', 'O', 'u', or 'U'. If the if statement holds, we print the given letter as a vowel. Otherwise, we printed that the given letter was a consonant.
Check Vowel Using Switch Statement
The switch statements are similar to the if-else statements. Following is the vowel or consonant program in C using the switch statement.
Code :
Output :
In the above program, we used the switch statement to check if a character was a consonant or a vowel. We created cases for all the vowels in the English language. If any of the cases were true, we printed that the given character was a vowel, and then we used the break keyword to exit the switch statement. If none of the cases was true, the default statement ran, which printed that the given character was a consonant.
Function to Check Vowel
When we want to check multiple times while different letters are vowels or consonants, it is better to define a function to avoid code repetition. The following code explains how to create a function to check vowels or consonants in C.
Output :
In the above program, we defined a function named check_vowel() to check if any given character is a vowel or a consonant. Inside the function, we have written an if-else statement. The if statement checks whether the given character is any of the 5 vowels in English. In case the ** if** statement holds, the function returns 1. Else, the function returns 0.
We have also created another function named print_is_vowel() that identifies whether a character is a vowel or a consonant. We used these two functions to check and print whether the given characters were consonants or vowels.
C Program to Check Vowel or Consonant Using ASCII Values
Each letter (both lowercase and uppercase) has an ASCII value. We can use vowels' ASCII values instead of the characters to check whether a letter is a vowel or consonant.
The ASCII values of a, e, i, o, and u are 97, 101, 105, 111, and 117, respectively. On the other hand, the ASCII values of A, E, I, O, and U are 65, 69, 73, 79, and 85 respectively. Following is the vowel or consonant program in C using ASCII values.
Output :
In the above program, we used the ASCII values of every vowel inside the if conditional instead of the vowel letters. In the else if statement, we checked whether the ASCII value of the given character was greater than or equal to 97 and less than or equal to 122 or if the value was greater than or equal to 65 and less than or equal to 90. If the if statement holds, we will print the given character is a vowel. If the else if statement holds, we would print that the given character is a consonant. If either of these statements does not hold, the compiler will not print anything.
Conclusion
- 'A', 'E', 'I', 'O', and 'U' (or 'a', 'e', 'i', 'o', and 'u') are the five vowels in the English language.
- We can use an if statement or a switch statement to check whether a given letter is a vowel or a consonant.
- If we have to check whether the given letters are vowels or consonants, we should use a function that checks this condition to avoid code repetition.
- We can use the ASCII values of vowels and consonants to check whether any given letter is a vowel or a consonant.