Java Program to Check Whether the Character is Vowel or Consonant
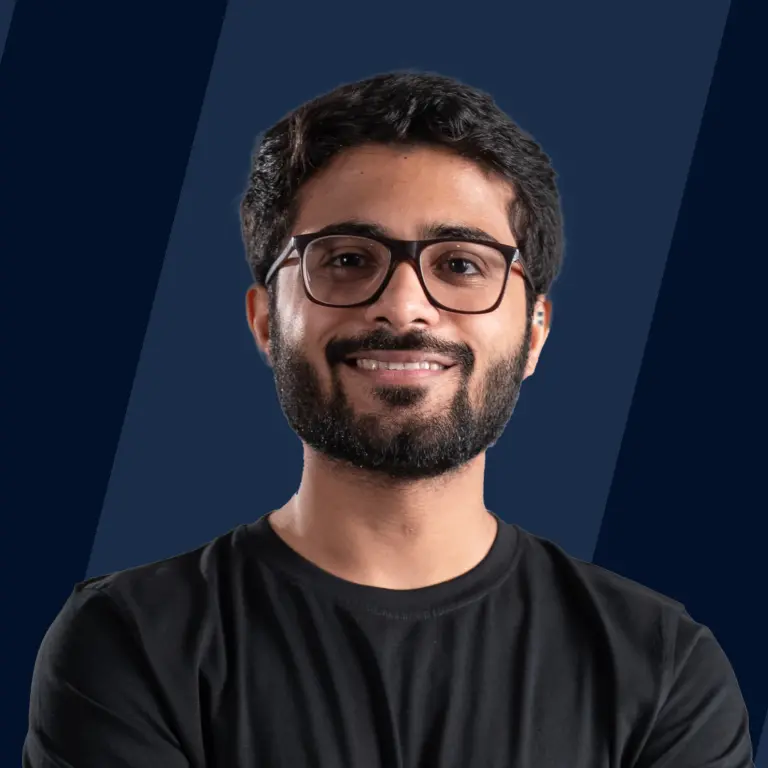
Overview
In Java programming, it is simple to tell if a letter is a vowel or a consonant. The letters 'a', 'e',' i','o', and ='us are vowels, whereas the remaining letters of the alphabet are consonants. You may write a basic Java program that accepts a letter as input and determines if it is a vowel or a consonant. You may conduct this categorization by utilizing conditional expressions such as if-else. This program can be useful for various applications, including text processing and language analysis, by guaranteeing that your code runs effectively with character-based operations.
Prerequisites
There are a few key criteria that you should be familiar with before plunging into the fascinating world of Java programming and learning about the vowels program in Java. Java, famed for its simplicity and robustness, provides an easy solution to complete this work. However, understanding these foundations will pave the way for a successful path into coding.
Java Basics:
To create the vowels program in java, you should have a solid grasp of the language's fundamentals. Recognize data types, variables, operators, and control structures such as loops and conditionals. These are the fundamental elements of every Java program.
JDK Installation: The Java Development Kit (JDK) is required. Download and install the most recent version. It contains the Java Compiler (javac) required to compile your code.
Fundamental Syntax: Java has tight syntactic requirements. Learn how to declare classes, methods, and variables properly. Keep name standards and indentation practices in mind.
Input/Output Concepts: It is critical to understand input and output management. You must read user input and present the results. Learn about classes like Scanner for input and System.out for output.
Conditional Statements:
You'll use conditional statements (if-else) to check whether a character is a vowel or consonant. Make certain you understand how to compose these sentences correctly.
By learning these requirements, you'll be ready to start your path toward developing a Java program that identifies whether a letter is a vowel or consonant. These fundamental abilities will help you not just with this project but also with future Java programming projects.
Checking Whether an Alphabet is Vowel or Consonant
In programming, determining whether a given alphabet is a vowel or a consonant is fundamental (vowels program in java). This task can be approached in several ways, each with merits. This section will explore three distinct methods to accomplish this task: an if-else statement, a switch statement, and the indexOf() method. To ensure you fully understand these concepts, we will give code examples, look at their results, and thoroughly explain them.
Using if else Statement
Most programming languages, including Java, C++, and Python, use the if-else statement as a basic component. We can use it in the vowels program in Java. It is employed for decision-making, which makes it an excellent candidate for categorizing alphabets as vowels or consonants.
Output:
Explanation:
- We begin by declaring a character variable inputChar and initializing it with the letter 'A'.
- The if-else statement in the code checks whether the inputChar is equal to any vowels in uppercase and lowercase.
- If the requirement is satisfied, the character is identified as a vowel; otherwise, it is identified as a consonant.
Using Switch Statement
We can also use the switch case in the vowels program in Java. The switch statement is another useful tool for the decision-making process in programming. It may appear less efficient for this activity, but it may still be used efficiently.
Output:
Explanation:
- We begin by assigning the letter 'B' to the character variable inputChar.
- The switch statement then compares the value of inputChar to various cases for uppercase and lowercase vowels.
- If a match is detected, it writes that the letter is a vowel; otherwise, it defaults to the consonant case.
Using indexOf() Method
The indexOf() method is typically associated with strings, but we can cleverly use it to determine if a character is a vowel or a consonant.
Output:
Explanation:
- We generate a vowel string that contains all capital and lowercase vowels.
- The character variableinputChar is then defined and set to 'C'.
- We use the indexOf() function to find the index of inputChar inside the vowels string.
- If the result is not -1 (indicating a successful find), the character is identified as a vowel; otherwise, it is identified as a consonant.
FAQs
Q. What is the aim of a Java program that determines if a character is a vowel or a consonant?
A. This program detects if a letter is a vowel or a consonant. It's a fundamental programming activity frequently used in text processing and validation.
Q. How does Java differentiate between vowels and consonants?
A. The program determines whether the character is one of the five vowels (A, E, I, O, or U). If it isn't one of these, it's classified as a consonant.
Q. Can this program be used for many characters or strings?
A. You certainly can. You may apply the same reasoning to each character in a string or array of characters.
Q. What if I enter a non-alphabet character, such as a number or a special symbol?
A. The program will treat Non-alphabet letters as consonants. You'd need to implement extra logic to handle non-alphabet characters differently.
Conclusion
- In this Java program, we successfully demonstrated how to detect whether a given letter is a vowel or consonant based on its ASCII (American Standard Code for Information Interchange) value.
- We've devised a compact and quick approach for character categorization by utilizing conditional statements and the logical OR operator.
- This program illustrates how Java uses its robust syntax and standard libraries to handle basic character operations.
- Understanding the categorization of letters into vowels and consonants is critical in many text processing jobs, and this program provides developers with a core ability.
- Developers can expand and incorporate this program into applications involving string manipulation or language analysis, adding to Java's adaptability in real-world circumstances.