What are Const Member Functions in C++?
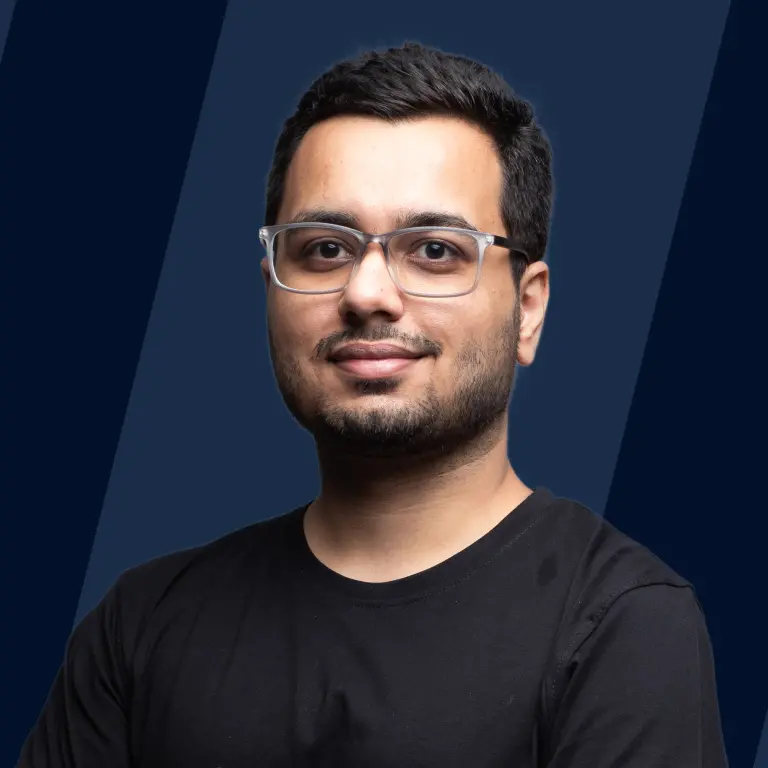
Constant member functions do not permit any change in the values of their class's data members. To make a member function constant, the term "const" is applied to both the function prototype and the function definition header. The const keyword signifies that a member function is a "read-only" function that does not alter the object for which it is called.
A class can also specify its objects as const, like member functions and member function arguments. A const object cannot be modified and can only invoke const member functions, ensuring that the object is not modified. A const object is constructed by prefixing the object declaration with the const keyword. Any attempt to alter the data member of a const object results in a compile-time error.
Syntax
Inside a class.
Outside a class as a standalone function.
Outside a class as its member
Example
Example of a Const Member Function Defined Inside a Class
Output
This program gives an error because we try to update a constant member function value.
Example of a Const Member Function Defined Outside a Class as a standalone Function
For a standalone function in C++, we use the concept of the friend function.
Output
This program will give an error if we try to change any value in the fun() function.
Example of a Const Member Function Defined Outside a Class as its Member Function.
Output
This program will give an error if we try to update the value of x in the get_value() function.
Why are const Functions used in C++?
We use const member functions in C++ to avoid accidental object changes. It is highly recommended to make as many functions const as possible so that the chances of error in the program are minimal.
Const Member Function Use Cases
The two use cases of the const member function are as follows:
- A const member function is called by any type of object, i.e., either by a const or a non-const object.
- A non-const function can only be called by a non-const object, and a const function cannot call it.
Calling Const & Non-Const Member Functions
Let's look at the program given below to understand the calling of const and non-const member functions.
Output
In this program, obj1 is a non-const function while obj2 is const object also get_square() is a non-const function while get_value() is a const function. As we know from the use cases of the const member function, the non-const function cannot be called by a const object. This is why the last cout gives an error while all the three don't.
Const Member Function Arguments
If you pass an argument to the non-const function by reference and you don't want that, the function changes the value of the variable. So we add the const keyword to the function's argument so that the function does not change its value. In the program given below, we add the const keyword to variables a and b so that the get_value() function can never manipulate the values of the variables.
Program
Output
This program gives an error as we try to update a const argument of the set_value() function.
Use of Const Keywords with Different Parameters
Use const variable
It is a const variable that is used to define variable values that will never change during program execution, and when we attempt to change the value results in an error.
Syntax
Program to use a const variable
Output
This program gives an error because we try to update the value of the const variable.
Note: In C++ programming, we must assign the value of the declared variables at the same time as we declare the const variable; otherwise, a compile-time error is displayed.
Use const with Pointers
To make a const pointer, precede the pointer's name with the const keyword. We cannot modify the address of the const pointer after it has been created. Therefore the pointer will always point to the same address once it has been initialized as a const pointer.
Syntax
Program to use const keyword with pointers
Output
This program generates an error as we try to update the address of the const pointer.
Use const pointer with variables
To use a const pointer with a variable we put the const keyword before the pointer variable. In this, we are allowed to update the address but we cannot change the value.
Syntax
Program to use const pointer with variables
Output
This program successfully updated the address stored in the const ptr pointer. This program will generate an error if we try to update the value stored in the pointer.
Use const with function arguments
We can use the const keyword to declare the function arguments as constant arguments. Furthermore, if the value of a function argument is marked const, it cannot be changed.
Syntax
Program to use const keyword with function argument
Output
The above program will generate an error if we try to modify the value of the arguments of the fun() function.
Use const with Class Member Functions
Constant member functions do not permit any change in the values of their class's data members. To make a member function constant, the term "const" is applied to both the function prototype and the function definition header. The const keyword signifies that a member function is a "read-only" function that does not alter the object for which it is called.
Syntax
- The function prototype is a function declaration that tells the program about the type of value returned by a function and the number and types of arguments. It is just like a function definition, except it has no body.
- A function definition is nothing but where we define the body of a function.
Program to use const keyword with class member functions
Output
This program will give an error if we try to update any value in the sum() function because it is a const member function of the Example class.
Use const with Class Data Members
Data members are similar to variables declared within a class, but once initialized, they never change, even in the constructor or destructor. The const keyword comes before the data type inside the class to initialize the constant data member. Const data members cannot be assigned values during their declaration, but they can be assigned constructor values.
Program to use const with class data members
Output
Use const with class objects
When we use the const keyword to build an object, the value of data members can never change during the object's lifetime in a program. Const objects are also referred to as read-only objects.
Syntax
Program to use const keyword with class objects
Output
This program will give an error if we try to update the value of the data member of class Example.
C and C++ Const Differences
- There is no difference in declaring the const variable.
- In C: const data_type variable_name=value;
- In C++: const data_type variable_name=value;
- There is a difference in syntax when you want to use a const variable in another module.
- In C: extern const data_type variable_name;
- In C++: extern const data_type variable_name=value;
- If you want to declare an extern variable in a C++ source code file for usage in a C source code file, use the format shown below to avoid name mangling by the C++ compiler. extern "C" const data_type variable_name=value;
Learn more
Also, read these articles on the scaler topics
Conclusion
- Constant member functions do not permit any change in the values of their class's data members.
- To make a member function constant, the term "const" is applied to both the function prototype and the function definition header.
- A class can also specify its objects as const, like member functions and member function arguments.
- We use const member functions in C++ to avoid accidental object changes.
- The two use cases of the const member function are:
- A const member function is called by any type of object, i.e., either by a const or a non-const object.
- A non-const function can only be called by a non-const object, and a const function cannot call it.
- We can also use the const keyword in the function argument. Doing this will make the function "read-only."
- There is no difference in declaring the const variable in C and C++.