What is a Function Prototype in C?
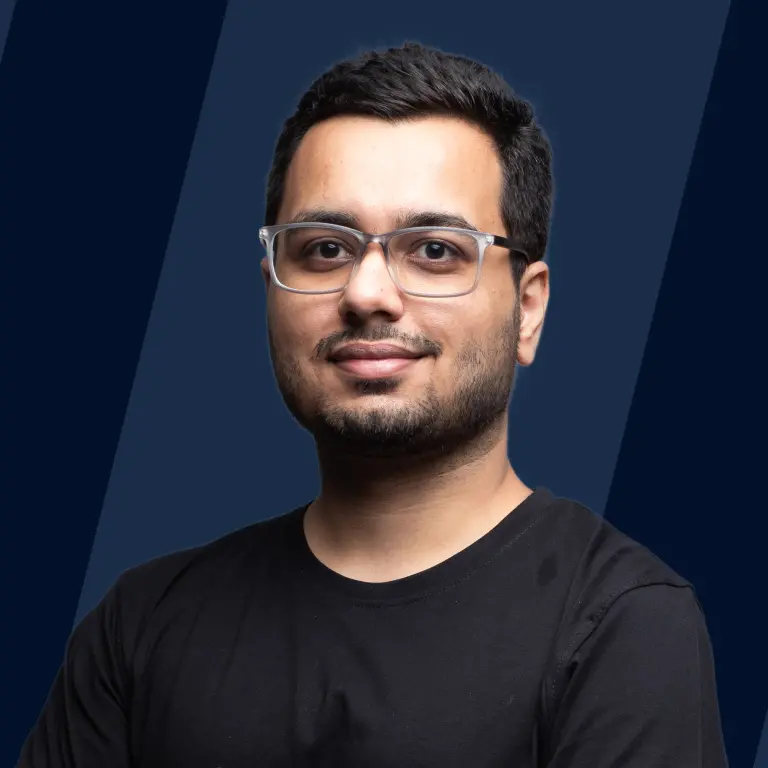
A function in C is a block of code that performs a specific task and function Prototype in C is the most important feature of C language that tells the compiler about the function return type, several parameters it accepts, the data type of parameters to avoid any warning and errors.
Syntax
For Example :
Here in this function prototype, we can easily understand that :
- Return type of the function = integer
- Number of parameters = 3
- DataType of Parameters = intger
Function Prototype in C Programming : Importance
In C language programs are compiled line by line and if we call a function before we have defined it in the program , we will get some warning like
[Warning] conflicting types for 'function' previous implicit declaration of 'function' was here.
So to avoid this we declare function prototypes at the start of our programs and may define the functions later on. So that the compiler gets to know that there exists a function with this method signature and hence doesn't give an error.
Program Without Function Prototype :
Here in this program, though we are getting the correct output we also get some warnings because we have not used the function prototype and have called the function before the function declaration in the main program. So to avoid this we will use a function prototype.
Code :
Output :
Warning :
Program With Function Prototype :
Here we have done the same program by using a function prototype and haven't gotten any warning. That's why function prototype is a very important feature of C language.
Code :
Output :
Examples of Function Prototype in C
Example - 1 : Odd Even Check Program in C using function prototype
Here in this example, we have created a function to check whether a given number is odd or even. We have written a function prototype for our odd-even check at the start of the program just after the header files and have defined the function later on.
Output :
Example - 2 : Program to Perform Multiplication of Two Numbers Using Function Prototype
This is a program to multiply 2 numbers. Here we have used a function prototype and have defined the multiply function first and defined it later in the program.
Output :
Difference between Function Prototype and Function Definition in C
Function Prototype :
In C language function prototype is used to provide function declaration. It defines the function's name, returns types, and parameters. The return types are the data types that the function returns after execution. If a function returns an integer, the return type is int. When a function returns a float value, the return type is also a float. Similarly, a void function does not return any value. The name of the function is used to uniquely identify it.
For Example :
Function Definition :
The actual implementation of the function is included in the function definition. It specifies what the function is expected to perform. The main logic of the function is written here. The control is handed to the function definition when the program calls the function and the control returns to the main function when the function is executed and completed.
For Example:
In a nutshell, we can say that a function prototype declares the function while a function defination contains the actual logic and steps for the operation to be performed.
Function Prototype in C : Scope and Conversion
The position of the function prototype in the program determines its scope. In most cases, the function prototype is placed in the program after the header files. The function prototype's scope is determined by its position in the program. It is regarded inside the scope of a matching function call if it is stated within the same block as the function call, any surrounding block, or at the source file's outermost level.
When a function is called, an argument is a value that is defined within the function. They are used in function call statements to transfer data from the caller function to the destination function. As a result, when we call a function, we must give parameters of the same data type as defined in the function prototype. Argument conversion comes into the picture when we call a function with arguments of different data types than those specified in the function declaration. This is also facilitated by the use of function prototypes.
The standard math library function sqrt, for example, contains a double-type argument in the function prototype. It may, however, be called with an integer parameter and works perfectly fine without any error.
Code :
In this program, we have shown that the sqrt function, although having a double type parameter in the function prototype, works correctly with integer datatypes.
Output :
Conclusion
- Function :
A block of code that performs a specific task. - Function Prototype :
It is used to provide function declaration. It defines the function’s name, returns types, and parameters. - Function Defination :
It specifies what the function is expected to perform and includes the actual code for implementation. - Scope of Function Prototype :
Considered Within the same block as the function call.