What is the Difference between range() and xrange() in Python?
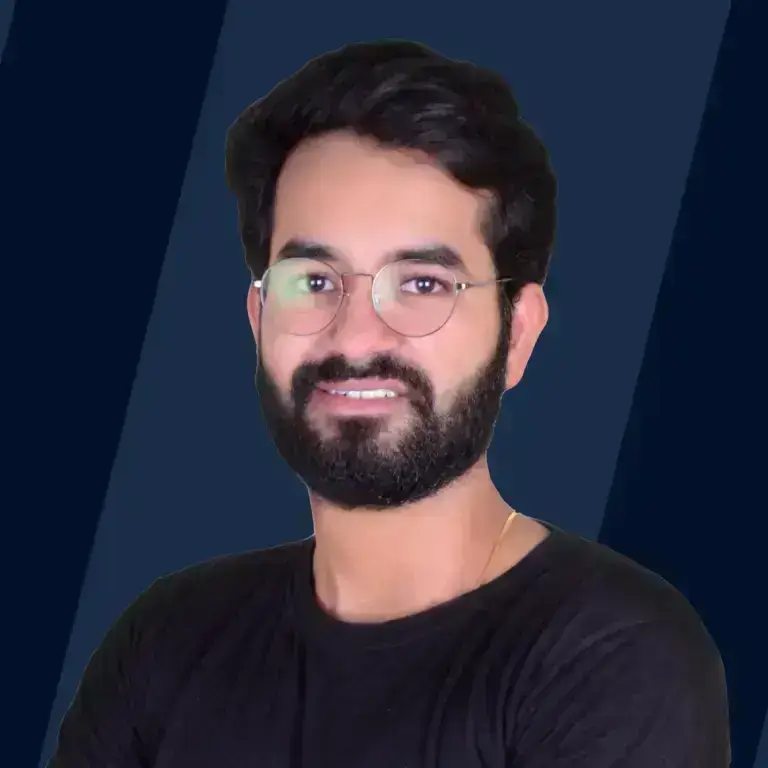
The range() and xrange() functions are built-in functions in Python programming that are used to iterate over a sequence of values. Both the range() and xrange() functions are used with the for() loops to iterate over certain number of times. Both the range() and xrange() functions create a list of the specified range of values. So, if we provide (1, 10) as the parameter to both the functions then it will create a list in the memory having 9 elements (1 to 10 excluding the 10th number).
The range() and xrange() functions are used in Python3 and Python2 respectively. As we know Python3 does not support backward compatibility, so the xrange() function (which was used in Python2) cannot be used in Python3 but the range() function can be used with Python2. Now, if we want our code to work on both the Python2 and Python3 interpreters then we should use the range() function.
Now, the difference between range and xrange in Python lies in their working speed and return values. The range() function is comparatively faster than the xrange() function. The range() function supports list slicing on the other hand, the xrange() function does not support list slicing. The range() function return a range a of iterable type objects on the other hand the xrange() function return the generator object.
Note:
- The range() function of Python-3 is basically a re-implementation of the xrange() function of Python2.
- List slicing is a way to get the subsets of any list or an array.
- Generator object is basically an iterator object having a sequence of values.
Let us now discuss both the range() and xrange() functions along with their example before getting into the detailed difference between range and xrange in Python.
range() Function
The range() function is a Python built-in function that is used to iterate over a sequence of values. Whenever the range() function is invoked, a sequence of numbers is created by the function and returned. The range() function helps us to iterate over a specific block of code certain number of times. The returned sequence of numbers starts with 0. By default the, numbers increments by 1 and the sequence ends before the specified number.
Note: range() is a data type in Python programming language. We can verify the same by using type() method.
The output of the print will be: <class 'range'>.
Syntax of range() Function
The syntax of range() function is very simple.
Parameters of the range() Function
The range() function takes three parameters in which two of them are optional and one is required. Let us briefly discuss the parameters of the range() function:
- start: It is an optional parameter. The start denotes the integer from where the iteration should start.
- end: It is a required parameter. The end denotes the integer where the iteration should stop. We should remember that the end integer is not included.
- steps: It is an optional parameter that denotes the number of increment(s) to be made in each iteration. By default the, steps value is set to 1.
The range() function in Python returns a sequence consisting of numbers that are immutables. These sequences of numbers can be easily converted into any sequential data types like tuples, lists, sets, etc.
Speed of range() function
The range() is more optimized than its ancestor xrange() function. The xrange() function needs to reconstruct the integer objects again and again, on the other hand, the range() function does not need to reconstruct the integer object hence it is faster than the xrange() function.
Note: The range() function only works with integers i.e. int data type. So, all the parameters of the range() function only accept integers but all the three parameters of the range() function can be negative as well.
Let us take a few examples to understand the usage of the range() function.
When Only One Parameter (stop) is Passed
Output:
When both the start and end Parameters are Passed
Output:
When all the Three Parameters are Passed
Output:
Let us now learn about the xrange() function before getting into the differences between the difference between range and xrange in Python.
xrange() Function
The xrange() function is a Python built-in function that is used to iterate over a sequence of values. It was used in Python2. Whenever the xrange() function is invoked, the generator object is created by the function and returned. Now, this generated object can be further used to display the values using iteration. The xrange() function helps us to iterate over a specific block of code certain number of times. The iteration starts at 0. By default, the numbers increments by 1, and the sequence ends before the specified number.
The xrange() function also only works with integers. So, all the parameters of the xrange() function accept integers but all the three parameters of the xrange() function can be negative as well. The negative steps denote the reverse order of iteration.
Syntax of xrange() Function
The syntax of xrange() function is very simple.
Parameters of the range() Function
The range() function takes three parameters in which two of them are optional and one is required. Let us briefly discuss the parameters of the range() function:
- start: It is an optional parameter. The start denotes the integer from where the iteration should start.
- end: It is a required parameter. The end denotes the integer where the iteration should stop. We should remember that the end integer is not included.
- steps: It is an optional parameter that denotes the number of increment(s) to be made in each iteration. By default, the step's value is set to 1.
The xrange() function in Python is the ancestor of the range() function. It returns a generator object of the xrange type. The generator object can be easily converted into any sequential data type like tuples, lists, sets, etc.
Speed of xrange() Function
As we have seen earlier, the xrange() function needs to reconstruct the integer objects again and again, on the other hand, the range() function does not need to reconstruct the integer object hence it is faster than xrange() function.
The xrange() function has a major advantage over the range() function as the xrange() function is more memory optimized.
Let us take a few examples to understand the usage of the range() function.
When Only one Parameter (stop) is Passed
Output:
When both the start and end Parameters are Passed
Output:
When all the Three Parameters are Passed
Output:
Let us now learn about the differences between the range() and xrange() function.
Comparison between range() and xrange()
We have already discussed the range() and xrange() functions in detail along with their various examples so let us now learn about the difference between range and xrange in Python.
range() function | xrange() function |
---|---|
The range() function is used in Python-3. | The xrange() function is used in Python-2. |
The range() function is faster. | The xrange() function is slower. |
The range() function returns a sequence (list) consisting of number that are immutables. | The xrange() function returns a generator object of xrange type. |
The range() function is less memory optimized. | The xrange() function is more memory optimized. |
The range() function supports arithmetic operations as it returns a list of integers. | The xrange() function does not support arithmetic operations as it returns a generator object. |
The range() function is supported by Python-2. | The xrange() function is not supported by Python-3. |
The syntax of range() function is: range(start, stop, steps). | The syntax of xrange() function is: xrange(start, stop, steps). |
Learn more
To understand more about Python programming language and important Python functions, refer to:
Conclusion
- The range() and xrange() functions are built-in Python functions that are used to iterate over a sequence of values.
- The difference between range and xrange in Python lies in their working speed and return values.
- The range() function is used in Python-3 and the xrange() function is used in Python-2.
- Both the range() and xrange() functions are used with the for() loops to iterate over certain number of times.
- The range() function of Python-3 is basically a re-implementation of the xrange() function of Python-2.
- Whenever the range() function is invoked, a sequence of numbers is created by the function and returned.
- The range() is more optimized as the xrange() function needs to reconstruct the integer objects again and again.
- The range() function only works with integers i.e. int data type.
- Whenever the xrange() function is invoked, the generator object is created by the function and returned. Now, this generated object can be further used to display the values using iteration.
- The xrange() function has a major advantage over the range() function as the xrange() function is more memory optimized.
- The range() function supports arithmetic operations as it returns a list of integers while the xrange() function does not support arithmetic operations as it returns a generator object.