What is Keyword in Java?
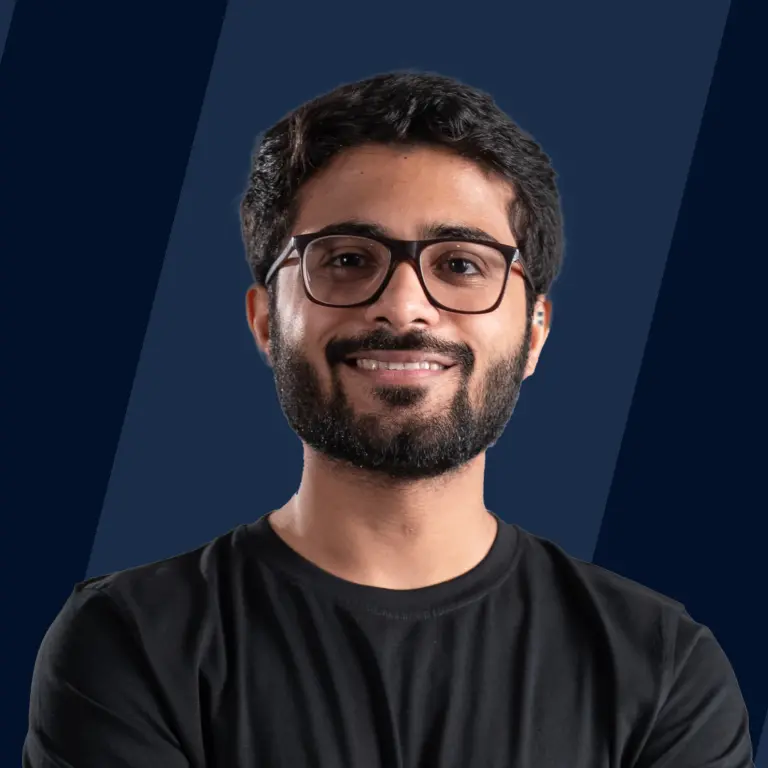
In Java, a keyword is a reserved word that conveys a specific meaning to the Java compiler.
Keywords are special words that have a predefined meaning to the Java compiler. They are reserved words and cannot be used as an identifier, function names, class names, etc in a programming language. However, if try to use them as an identifier, variable name, etc then we will be getting a compile-time error.
Java contains 50 such keywords that are reserved for specific purposes in the Java language. Some of the keywords are int, break, implements, float, etc. Since they are reserved words that convey a special meaning to the Java compiler, therefore, they are highlighted with different colors in any IDE or editor to differentiate them from the other words.
In this article, we will be discussing all the keywords one by one that is predefined in Java.
List of all Java Keywords
Keyword | Usage |
---|---|
abstract | It is used to declare a class or a method as abstract. A method with no definition is known as an abstract method whereas the class that contains that method is known as an abstract class. Declaring a class or method as abstract means that it would be implemented later in the subclass. |
assert | This keyword allows the developers to quickly verify the assumptions in a program. |
boolean | It is used to define a boolean variable that takes the value as only true or false. By default, its value is false. For example: boolean b = true |
break | It is a control statement that is used to break the execution of a loop and come out of the loop.For example: |
byte | This keyword is used as a data type that can hold 8-bit values. |
case | This keyword is used inside the switch statement to specify various matching cases. However, they also mark a block of text inside the switch statement. For example: |
catch | They are used in conjunction with the try block and an optional finally block. This keyword is used to catch an exception or, in other words, specify what to do when an exception is thrown by the try block. |
char | This keyword is used as a data type that can store any character that is defined in the Java character set.For example: char ch = 'a'; |
class | It is used to define a class. It is a type that defines a particular kind of object in Java. class A{ |
const | It is a reserved word in Java, however, it is not used and has no function in Java programming language. |
continue | It is used to send the control back to the loop skipping all the statements present after it in a program. For example: |
default | It can be optionally used in the switch statement which is executed when no case is matched with the specified value. For example: |
do | It is used in conjunction with the while loop to make a do-while loop in Java.For example: |
double | It is a data type in Java that can hold up to 64-bit floating-point numbers. For example: double d = 4.6786; |
else | It is used in conjunction with the if statement to test a boolean expression. For example: |
enum | It is used to declare an enumeration type. They extend the base class. |
extends | It is used in the class declaration to indicate that the class is inherited by another class or interface. For example: class A extends B. |
final | It is used to specify that an entity cannot be changed if declared final such as a final class cannot be subclassed, a final variable will hold only a constant value, or a final method cannot be overridden. |
finally | It refers to a block that is used with the try-catch structure and will always be executed. |
float | It is used as a data type in Java that can hold a 32-bit floating-point number. For example: float f = 4.5f; |
for | It is used for looping around a set of statements in a program. It is used to start a loop. For example: for(int i=0;i<100;i++){ |
goto | It is also a reserved keyword in Java, however, never used. |
if | It is used to specify an if statement which is used to test a boolean expression.For example: |
implements | It is used in a class definition to specify the interfaces that are implemented by the class. |
import | It is used to include the classes or the entire Java packages to be referenced later in the program without including the package name in the reference. For example: import java.util.Scanner; |
instanceof | It is used to check if an object is an instance of a particular class or if it implements an interface. |
int | It is used as a data type in Java that can hold a 32-bit signed integer. For example: int a = 5; |
interface | It declares a special class with contains only abstract methods, final fields, and static interfaces. However, they can later be implemented by the classes. |
long | It is also used as a data type in Java that holds a 64-bit integer. For example: long l = 400389l; |
native | It is used to specify that the methods are implemented in its native code using Java Native Interface. |
new | It is used to create new instances and objects of a class. |
package | It is used to declare a Java package. Packages are a group of classes and interfaces. |
private | It is an access specifier and is used to specify that the method/variable is accessed only in the class in which it is declared. |
protected | It is also an access specifier and is used to specify that the method or variable is accessible in its class, subclass, or the classes from another package. |
public | It is an access specifier that is used to specify that the method, variable, classes, or interfaces are accessible by the members of any class. |
return | It is used to indicate the end of the execution of a method and also returns a value back to the caller method.For example: int cal(int i){ |
short | It is used as a data type that is capable of holding a 16-bit integer. |
static | It is used to declare a method or variable to a class method rather than limiting it to a particular object. |
super | It is used to refer to the base class of the current class. However, it is used in a method and a constructor. |
switch | It is used to execute a code based on the test value, however, after which this test value matches with every case inside the switch statement and executes the matched test case. |
synchronized | It is used to specify critical sections in a multithreaded code in Java. |
this | It is used to represent an instance of a class in which it is used. |
throw | It is used to explicitly throw an exception, mainly custom exceptions. However, it is followed by an instance. |
throws | It is used to declare an exception and tells what exceptions will be thrown by a method in Java. |
transient | This keyword is used in serialization, if you define a data member as transient, it will not be serializable. |
try | It is used to define a block of code that would be checked for exception handling. |
void | It is a data type in Java and used to return nothing. For example: |
volatile | It is used to show that a variable may change asynchronously. |
while | It is used to execute a block of statements based on the truth and falsity of a particular condition.For example: |
What If We Use the Keywords as the Variable Name?
If we use the keywords as variable names in Java, they would give us a compile-time error as it is against the rules for naming a variable.
The rules for the naming convention of variables clearly mention that a variable name should not be a keyword. However, keywords are also reserved words for specific purposes and are not supposed to use for any other purpose in Java.
Let us see it with an example.
Output:
Learn More
You can refer to the following articles for more information on different keywords in Java.
Explore Scaler Topics Java Tutorial and enhance your Java skills with Reading Tracks and Challenges.
Conclusion
- Keywords are reserved words that convey a special meaning to the Java compiler.
- They are reserved for specific purposes in Java.
- There is a total of fifty keywords that are used in Java.
- The const and goto keywords are, however, reserved but are not used ever.
- If we use a keyword as a variable name, it would give us a compile-time error in Java.
- However, if you use keywords such as identifier, method_name, or class name, it would still give us a compile-time error.
- Therefore, they should be used just for the purpose they are assigned to and not anything else.