What is Method in JavaScript?
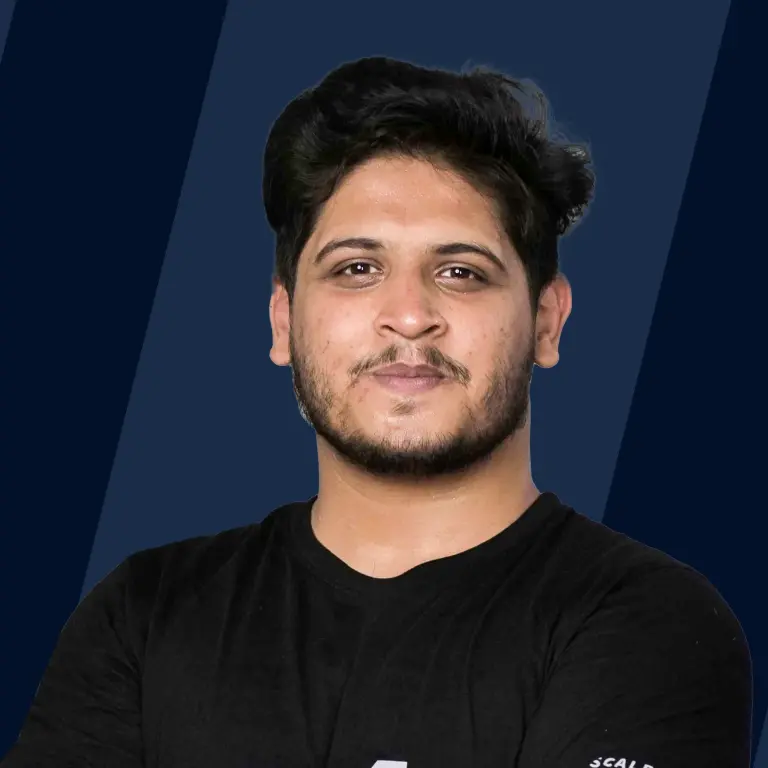
Methods and functions are useful in cases where you need to perform some operation again and again and your code becomes repetitive. They are chunks of code that perform specific tasks and return some values. A method is a function that is a property of an object. Some methods are built-in while others must be created based on our specific needs.
What is a Function in JavaScript?
A function in JavaScript is defined as a chunk of code that is used to perform some specific operation in a program. A function works on some input values provided by the user and returns the output after performing the task. The purpose of making a function in JavaScript is to avoid the repetition of code in a program. Functions in JavaScript improve code reusability and hence, increase the overall efficiency of the code.
Imagine a coaching academy with 50 students, and the task is to create a program for recording the total marks for each student. To streamline the process for all 50 students, we leverage the concept of functions. A function, designed to receive input for each student's marks in every subject, eliminates code redundancy. By defining functions once, we can easily reuse them throughout the program, making it more efficient and concise.
To create a function in JavaScript, you need to use the keyword "function" before any function name.
Syntax
The syntax of the JavaScript function is as follows:
There are some rules regarding the working and behavior functions in JavaScript which are as follows:
- The function gets executed only when it gets invoked or called in the program.
- The function name can be declared using letters, digits, dollar signs, and underscore.
- The parameters that we provide inside a JavaScript function should be enclosed within the parenthesis.
- Arguments are the values that a JavaScript function receives while functioning.
Example
Let us take an example of a function in JavaScript for a better understanding.
Output:
Explanation:
In the above example, you can see the function addition is declared using the keyword "function" which performs "x+y". Here, x and y are the arguments/input parameters passed into the function.
What is a Method in JavaScript?
A method in JavaScript is defined as a function that belongs to some class. In other words, a method is a function that is a property of an object. In JavaScript, methods are functions that are associated with an object and are called using the dot notation.
Think of a car mechanic who specializes in repairing engines. The mechanic is like a standalone function. You can take any engine (object), and the mechanic (function) will inspect, diagnose, and repair it. Now, let's say your car has a "start" button. The "start" function is like a method associated with the car object. You don't just press a generic "start" button; you press the "start" button on your specific car.
Let us see the syntax of methods in JavaScript.
Syntax
The syntax of the method in JavaScript is as follows:
Example
Let us see an example of methods in JavaScript to understand the working of it:
Output:
Chaining Method
Method chaining in programming, including in JavaScript, is a technique where multiple methods are invoked on the same object in a single line of code. This results in a more concise and readable code structure. Each method in the chain operates on the object and returns the modified object, allowing the next method in the chain to be called. Let us see the syntax of this method.
Example:
Let's create a hypothetical MyClass with three methods (method_1, method_2, and method_3) that return modified instances of the class. Here's an example:
Output:
How to Add Method to an Object in JavaScript?
As we know, an object in JavaScript is defined as an entity having some properties. This property of the object can be many things such as a variable, or some methods. This method will decide how the object will function in the JavaScript program.
The syntax that is used to ass a method to the object in JavaScript is as follows:
In the above syntax,
- functionName: It is the predefined function that has been created in the program by the user.
- newMethodName: This is the name of the method that is going to be added to the JavaScript object.
- parameter_1..: These are the different parameters of the method that will be passed in the new method.
Example:
Output:
What are Built-In JavaScript Methods?
Built-in methods in JavaScript are the pre-defined methods that come along with the programming language package. They can be directly used by passing the input parameters, without any need for explicit implementation. They are designed to perform common operations, simplify coding tasks, and enhance the functionality of the language. Like any other programming language, JavaScript also offers some built-in methods. For example:
Explanation:
In the example above, toUpperCase()converts a string to uppercase. We can complete this task without even defining the function!
There are many such built-in methods that you can directly use in your program. These are:
- Date()
- Date.now()
- Math.round(num)
- string.length()
- string.toLowerCase()
- array.length
- Math.floor(num)
- array.push()
Difference between Functions and Methods in JavaScript
Function and methods are two very commonly used and also confusing. Let us see the differences between the functions and methods and compare them using a table.
Function | Method |
---|---|
A function in JavaScript is a chunk of code that is designed to perform a specific task. | A method in JavaScript is defined as a property of an object that has some functional value. |
The data passed into a function is explicit. | Methods operate on data contained in a class. |
A JavaScript function can be called directly in the program by using its name. | A JavaScript method can be called by the object of the class. You can use the dot notation or square bracket notation. |
The following code snippet demonstrates both the use of a function with a basic argument and a method associated with an object.
This demonstrates the difference between a function that operates independently with its arguments and a method associated with an object that can access the object's properties using the this keyword.
FAQs
Q1. What is the basic difference between a function and a method in JavaScript?
A. The primary difference is that a "function" is a standalone block of code that can be invoked independently or as part of an object, while a "method" is a function that is associated with an object and is invoked on that object using the dot notation. All methods are functions, but not all functions are methods.
Q2. What are built-in methods?
A. Built-in methods in programming refer to functions or procedures that are provided by the programming language itself or are part of a standard library. These methods are pre-defined and can be used without the need for explicit implementation.
Q3. What are the benefits of chaining?
A. Method chaining in programming, when used appropriately, can contribute to code readability and maintainability, but it doesn't inherently improve the efficiency of a program in terms of execution speed or resource usage. Instead, the benefits of method chaining are more related to code organization and expressiveness.
Conclusion
- A method in JavaScript is defined as a function that belongs to some class.
- Built-in methods are the methods that come along with the programming language package. You do not need to implement them, you can directly use them in your program.
- You can use the dot (.) operator to call the method via the object.
- Methods work on the data contained within the class.
- Chaining refers to the process of calling a method on another method of the same object.