What is Static Data Members in C++?
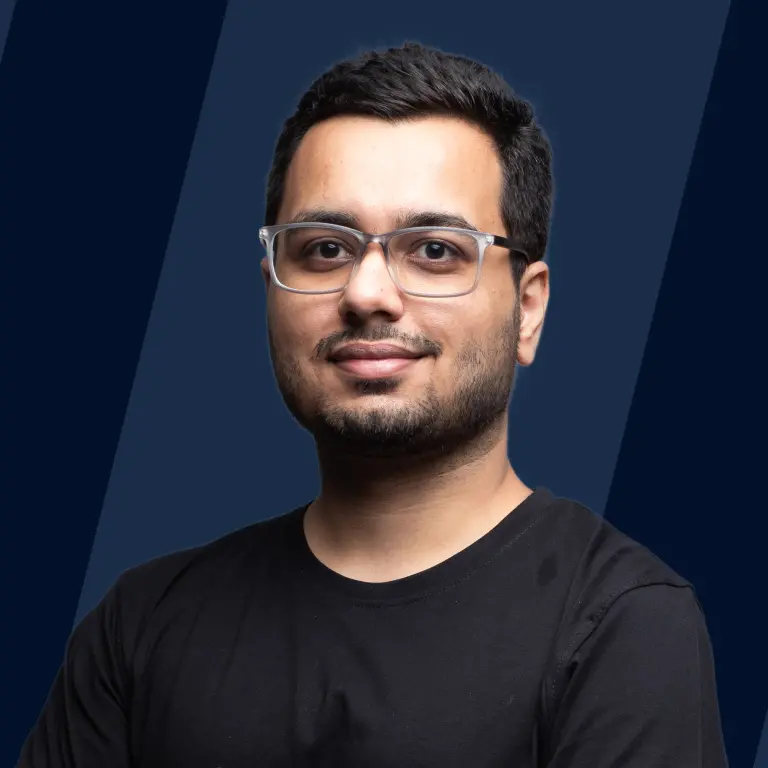
A static data member in C++ is a data member defined in a class that is not instantiated with each object created of the class. Data members defined in a class are usually instantiated with every object created of the class. That is, each object of the class will have their own instances or copies of the data members defined in the class. However, if a data member is initialized with the static keyword, that data member will not be instantiated and there shall exist only one copy of that data member for all objects or instances of the class.
A static data member in C++ can be defined by using the static keyword. Outside of classes, the static keyword is used to define elements of the static storage class. A static data member in C++ is declared inside a class but is defined within static member functions or outside the class using a scope resolution operator(::).
Syntax
A static data member in C++ can be defined by using the static keyword. The static keyword appears before other specifiers like data type, etc. A static data member can be declared in the following way:
For example:
The example above declares a static data member x within class A. A non-const, that is, a static data member which is not declared as const in C++ cannot be defined or initialized within the class in which it is declared. It is instead defined outside the class using the scope resolution operator as in the following example:
Static Member Functions
A static member function is a member function of a class that is defined with the static keyword. For example:
The sayHello() function above is a static member function of class A.
- Static data members of a class can only be defined within static member functions and a static member function can call other static member functions of the class.
- A static member function cannot define a non-static data member and cannot call a non-static member function.
- A static member function need not be instantiated and can be called without creating any object of the class by using the scope resolution operator(::).
Static Data Members
A static data member in C++ is a data member defined in a class that is not instantiated with each object created of the class. In C++, each object or instance of a class consists of its own set of data members that are defined within the class. However, data members that are specified with the static keyword are not instantiated for each object of the class and the class will have only one instance of that data member.
Static data members in C++ are not associated with any object and can be accessed even without the creation of any object. Static data members belong to the static storage class in C++ and as such have a lifetime equal to the lifetime of the program.
Static data members cannot be declared or manipulated by normal member functions and cannot be accessed directly through an object of the class. A static data member is defined with the help of the scope resolution operator and is defined outside the class. A static data member in C++ can only be manipulated with the help of a static member function as in the following example.
Output:
In the above example, the data member x of class A is declared as a static data member. It is defined outside the class with the help of the scope resolution operator(::) and is accessed and manipulated with the help of a static member function.
Constant Static Members
A non-const static data member in C++ cannot be initialized within the class in which it is declared. However, if a static data member of integral or enumeration type is declared const it can be initialized inside the class definition as in the following example:
For static data members of LiteralType which are declared using the constexpr keyword, they must be initialized with an initializer right inside the class definition as in the following example:
Accessing Static Data Member Without Static Member Function
As mentioned above, a static data member in C++ can be defined with the help of the scope resolution operator(::). Similarly, static data members can also be directly accessed with the help of the scope resolution operator(::) as in the following example:
Output:
Examples
Example 1:
Output:
In the example above, the static member function getStatic() is used to access the static data member x of class A.
Example 2:
Output:
In the example above, we have used the scope resolution operator to access and increment the static member x of class A. It is important to note that in this example, there are no objects of class A created and yet we are able to manipulate the value of the static data member.
Example 3:
Output:
The above example is an important use case of static data member in C++. The constructor of the class A increments the value of the static data member x. Thus, we can use the static data member to keep count of the number of times an object of class A is created in the program.
Transform your passion for coding into C++ expertise. Join Scaler Topics certification course and become a proficient C++ developer.
Conclusion
- Static data members in C++ are not instantiated for each object of a class and instead, only one copy of the data member exists for the entire class.
- A static data member in C++ is declared within a class and is defined outside the class.
- A static data member in C++ can be accessed with the help of the scope resolution operator(::) or a static member function.
- A constant static data member in C++ can be initialized within the same class in which it is defined.