What is String in Python?
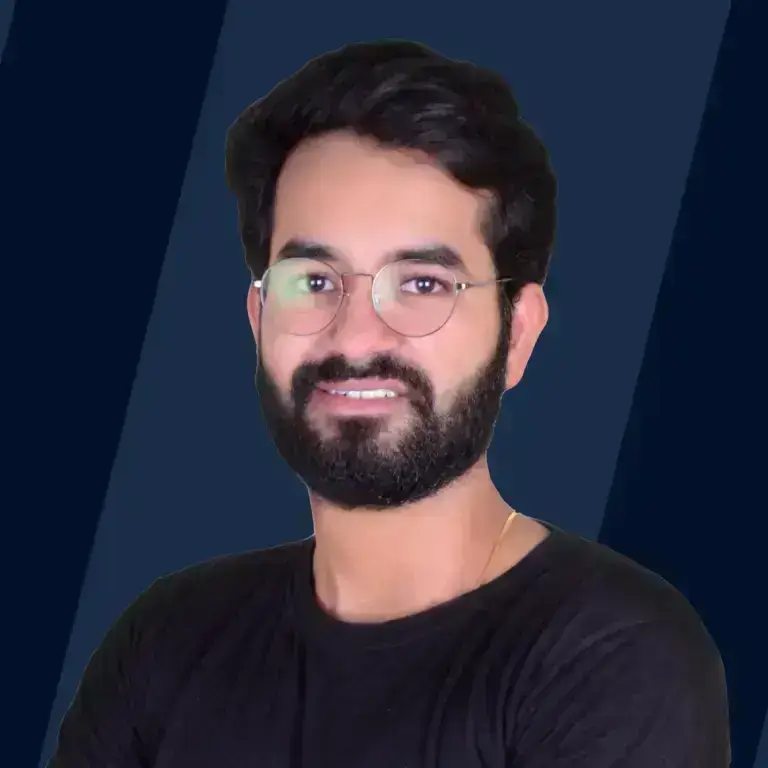
Strings are arrays containing bytes that represent Unicode characters. In Python, there is no data type named character. Characters are strings of length one.
For more basic information about what is a string in python please refer to Strings in Python
Note : str() is a keyword in python, so it is good not to use it as a variable name.
Creating a String
- Strings are created by enclosing characters inside single quotes, double quotes, or triple quotes.
- Triple Quotes declaration is used when we need to write multi-line strings.
Accessing Characters in Python
- We can access individual characters using indexing and the range of characters using slicing.
- Index starts from 0.
- Trying to access a character out of index range will raise an IndexError.
- Index must be an integer.
- Python allows negative indexing for its sequences.
- The last item has an index of -1, the second last item has an index of -2, etc.
String | H | e | l | l | o | W | o | r | l | d | |
---|---|---|---|---|---|---|---|---|---|---|---|
Positive Index | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
Negative Index | -11 | -10 | -9 | -8 | -7 | -6 | -5 | -4 | -3 | -2 | -1 |
String Slicing
To access a range of characters in the string, slicing is used. The Slicing operator is used to slice a String i.e. colon.
Check out this article to learn more about String Slicing in Python.
Deleting/Updating from a String
- We cannot update or delete characters of strings after declaration as strings in python are immutable.
- Deletion of an entire string is possible with the help of the del keyword.
Concatenation of Two or More Strings
We can concatenate two strings with the help of the '+' operator. The '*' operator can also be used to make a string repeat a certain number of times.
Iterating Through a string
There are two methods to iterate through the string with the help of for loop.
Python String Length
We can find string length using two methods
Method - 1 : Maintaining count variable
Method - 2 : Using built in len() function
Python String Formatting
String formatting can be performated using two methods :
Method - 1 : using format() method
- It specifies the values and inserts them inside the string's placeholder.
- Placeholder is defined using curly brackets : {}.
- Syntax : string.format(value1, value2, ....). Values are inserted in the string.
Method - 2 : formatting string using F-string
Conclusion
- Square brackets are used to access the string's elements.
- String in Python are immutable i.e. cannot be changed.
- Strings are created by enclosing characters inside single quotes, double quotes, or triple quotes.
- Python allows negative indexing for its sequences.
- We can concatenate two strings with the help of the '+' operator.
- The '*' operator can also be used to make a string repeat a certain number of times.
- len() function is used to find the length of a string
- String formatting can be done with the help of the format() method and F-string.