What is Wild Pointer in C?
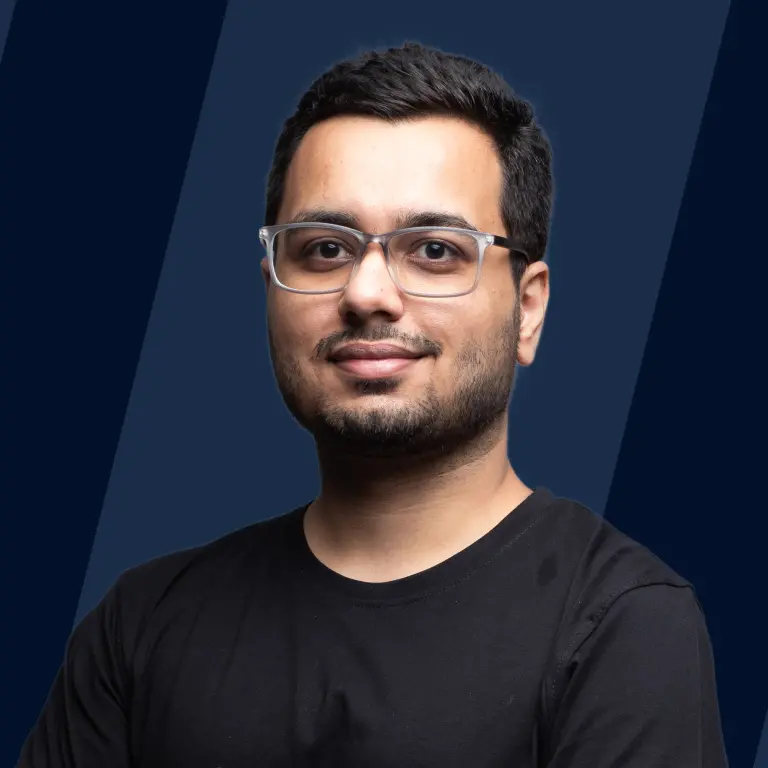
We know that pointers are a special kind of variable that stores the address of other variables, and how do they store the address? We initialize the pointer variable with the address of another variable, like this
Now ptr holds the address of the num variable, meaning it is storing some meaningful address, an address which if we dereference then we will get some meaningful value
The concept of wild pointers is they are almost the same as a normal pointer variable, the only difference is, that they are an uninitialized pointer variable, meaning they are not initialized at the time of their declaration, and with being uninitialized, they start to point to some arbitrary memory location and sometimes may cause the whole program to crash
In simple words,
A wild pointer is a pointer that is not initialized till its first use in the program hence by default they then hold some arbitrary memory location, and if we try to do any operation using them, then it can cause our program to crash
ie, a pointer that store neither any valid address in it nor the NULL value, then the pointer is a wild pointer
Syntax
We can see that, we just have declared a pointer, but have not given any value to it, so it starts pointing to some random arbitrary memory location, and hence is referred to as a wild pointer
Example
Wild Pointer in C
Output:
What we are doing here is, we are declaring an int pointer ptr but not assigning it to any address, so it becomes a wild pointer, now in the second line, we are trying to access that arbitrary address which ptr is pointing to and are trying assigning value there, which is an incorrect way as per the c standards, and then we are trying to print the value, which will result in unexpected behaviour or can cause the program to crash
Note - some compilers may allow the above code to execute and print the value, but this should be avoided
Instead of this, the above code can be written as
Output:
In simple words:
If we only execute line 1 and line 2 in the above code, then ptr will be a wild pointer here, as we are not storing any value in it, and hence will store an arbitrary memory location, But if we execute line 3 as well then now ptr will not be a wild pointer anymore, as we are making it point to a meaningful memory address, and therefore it will become a normal pointer.
Disadvantage of Wild Pointers
- As when not initialized, a pointer becomes a wild pointer, and starts pointing to some random memory address, which may lead our program to behave unexpectedly or sometimes it may crash as well
- If by mistake we ever tried dereferencing an uninitialized pointer in our program, then it can result in some unexpected behaviour
How to Avoid Wild Pointer in C?
We know that wild pointers mean a pointer storing some random memory location, so how to avoid it? It's simple, we should assign it to some meaningful address Let's understand this
1. We can assign the pointer with some valid memory address at the time of its declaration
Output:
2. We can directly use functions like malloc(), and calloc() to assign a pointer a valid memory address
Output:
A Quick Explanation:
- Functions like malloc() and calloc() are used to dynamically allocate the memory in C language, so here above we are dynamically requesting memory for our pointer ptr and then typecasting it to an int pointer, as both of these functions return a void pointer
- Then we are checking if the memory allocation was successful, as it may happen, the compiler was not able to fulfil our request due to many possible reasons, and hence we have not been allocated any memory, so we are checking, if the memory allocation was successful ie. if not, then we are showing the memory allocation failed message, else we are storing a value at that address with the help of the pointer and then printing it
3. If we do not want to assign any memory to our pointer at the time of its declaration, then we can initialize the pointer with NULL (null pointer)
Output:
Explanation:
- NULL is an assignment, that means "nothing", ie when we want to declare a pointer variable but are not intended to immediately make it point to something meaningful, and also we do not want that pointer to start pointing to some arbitrary memory location, then we can assign it with NULL In simple words, when we declare a pointer as NULL, we are saying, okay, we are declaring a pointer now, but we will use it later, and hence to prevent that pointer to point to some random memory location, we make it point to NULL
- Later in our program, when the use case came, we are assigning a memory to the pointer ptr
- If memory allocation was successful, then storing the value9 at that address, and then finally printing it
Dereferencing a Wild Pointer
As wild pointers point to some random memory location, so dereferencing them is not logical, as we cannot be sure about the data in the memory our pointer is pointing to ie, what type of data is stored there, is that data even meaningful or it is some garbage value? This is why it is advised not to dereference a wild pointer, and if we sometimes try to dereference them, then it may lead our program to crash or become unresponsive
To read more about Pointers, visit here
Conclusion
- Wild Pointers are a special type of pointer, which does not store any meaningful address nor NULL in them, they point to some arbitrary memory location
- They are created, when we just declare a pointer and do not initialize it right at the time of its declaration
- Syntax : int *ptr; // ptr is now a wild pointer
- Wild pointers can cause our program to behave unexpectedly and sometimes they might crash the program
- To avoid wild pointers, we can either make their point to some valid memory address or we should make it point to NULL
- int *ptr = # // ptr is storing a valid memory address, assuming num is already declared
- int *ptr = (int*)malloc(sizeof(int)); // dynamically allocating a valid memory address to ptr
- int *ptr = NULL; // making ptr point to NULL
- It is advised to avoid dereferencing a wild pointer, doing so can cause our program to be unresponsive