Which Operator Cannot Be Overloaded in C++?
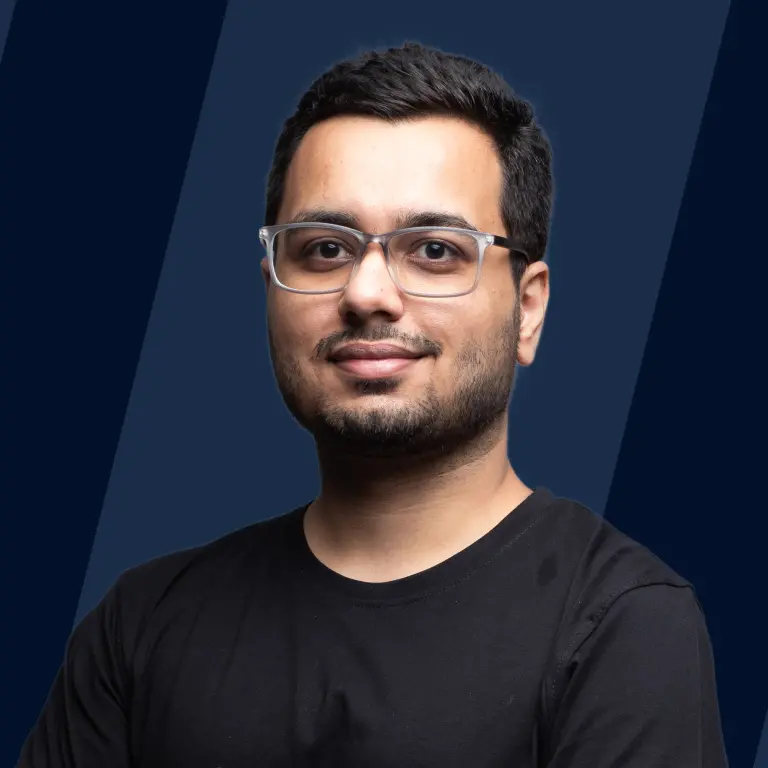
Before learning about the operators we cannot overload let's learn about what is Operator overloading and which operators can be overloaded.
Operator overloading in C++ is a type of compile-time polymorphism in which most of the built-in operators can be redefined or overloaded for user-defined data types. A user can redefine an operator with a special meaning. In simple words, Operator overloading means redefining a pre-defined operator to perform a special task rather than the operation it usually performs.
To learn more about the concepts of operator overloading, visit Operator Overloading in C++.
There are several ways used for implementing operator overloading in C++:
- Member Function
- Friend Function
- Non-member Function
By using operator overloading, we can redefine most of the C++ operators. It is used to perform different operations using one operator and also makes it possible for the templates to work equally well with classes and built-in types.
The list of operators which can be overloaded:
- Arithmetic Operators like %, +, -, *, /
- Unary Operators like ++, --,!
- Relational Operators like ==, !=, >=, <=
- Logical operators like && and ||
- Assignment operators like =, +=,*=, /=,-=, %=
- Subscript operator [ ]
- Bitwise operators like &, |, <<, >>, ^, ~
Note: Only existing operators in C++ can be overloaded and the overloaded operator must have an operand of the user-defined type.
Now, let's learn about which operator cannot be overloaded.
Operator overloading in C++ supports the overloading of any existing operator but there are some exceptions in operators which cannot be overloaded.
Generally, those operators cannot be overloaded because it can cause serious programming issues or errors, and also it is syntactically not possible.
For Example, the sizeof operator cannot be overloaded as it returns the size of an object or datatype entered as an operand which is assessed by the compiler and can not be evaluated during the runtime. If we still try to overload the sizeof operator this will throw an error.
Now, out of all the built-in operators, we will see which of the following operators cannot be overloaded.
List of Operators That Cannot Be Overloaded in C++
The list of operators which cannot be overloaded is as follows:
- Conditional or Ternary Operator (?:) cannot be overloaded.
- Size of Operator (sizeof) cannot be overloaded.
- Scope Resolution Operator (::) cannot be overloaded.
- Class member selector Operator (.) cannot be overloaded.
- Member pointer selector Operator (.*) cannot be overloaded.
- Object type Operator (typeid) cannot be overloaded.
Among the in-built operators which operators cannot be overloaded using the friend function but can be overloaded by member functions are as follows:
- Assignment Operator (=)
- Function call Operator (())
- Subscript Operator ([])
- Arrow Operator (->)
Now, let's see an example to discuss which operator can not be overloaded.
Example
Let's discuss an example of overloading a Dot(.) operator or class member selector operator which will throw an error as this operator has a pre-defined meaning which cannot be redefined by the user.
Code:
Output:
Example Explanation:
The class member selector operator or Dot(.) operator cannot be overloaded and it will throw an error as seen in the output.
Overloading the Dot(.) operator will arise a conflict in the meaning as the operator may be used for object overloading or to refer to an object of the class.
Why These Operators Cannot Be Overloaded in C++?
There is a fundamental reason for not allowing the overloading of some specific operators. As we have discussed earlier, overloading these operators may alter the pre-defined meaning of these operators. The specific operators are already overloaded to some sort. If we try to overload them it will arise serious syntactic and programming issues.
As we have seen earlier, which operator cannot be overloaded. Let's now find out why these operators cannot be overloaded by discussing them one by one:
-
sizeof()- The alteration in the meaning of the sizeof operator using its overloading causes a fundamental part of language to fail. The sizeof operator returns the size of the data type or the object used as its operand, and incrementing the pointer in an object array implicitly depends upon the sizeof operator.
-
Scope resolution(::)- The scope resolution operator is used to define or access a variable or member function outside the scope of the respective class and to refer to a class inside another class. They are also used in multiple inheritance, if a variable having the same name is declared in two-parent classes then we use the scope resolution operator to differentiate between the variables.
These operations are completely evaluated at runtime. The operand used for scope resolution operators are expressions with data types and there is no syntax for distinguishing them if they are overloaded.
-
Ternary Operator or conditional operator(?:)- The ternary conditional operator is a substitute method for an if-else statement. This operator takes three operands and that is why they are called the ternary operator.
According to the condition, the operator gives a true or false outcome. Overloading the ternary operator would alter the actual meaning and cannot guarantee that only one of the expressions will be evaluated.
-
Class member access operator(.)- The class member access operator is used to access the variables or member function of the class. So, to ensure error-free access to the class members it is essential to use a class member access or dot(.) operator. We cannot overload these operators as it may alter the actual meaning of the operator.
-
Object type operator(typeid)- The typeid operator is used to identify the type of the object. The object's actual derived type is identified by the typeid operator. If a user-defined type is defined as another type which can be done using polymorphism but there should not be any alteration in the meaning of the typeid operator otherwise it will cause serious programming issues.
Learn more
- Operator Overloading in C++- Operator Overloading is a compile-time polymorphism concept where a built-in operator is redefined for user-defined data types.
To learn more about the overloading of C++ operators, visit Operator Overloading in C++.
-
Polymorphism- The word polymorphism means many forms. The meaning of the same object can be manipulated in different contexts.
There are two types of polymorphism:
- Compile-time Polymorphism
- Run-time Polymorphism
To learn more about polymorphism in C++, visit Polymorphism in C++.
Conclusion
- Operator overloading is a method that allows redefining of the pre-defined operator for user-defined data types.
- Most of the operators used in C++ can be overloaded which includes Arithmetic Operators (%, ), Unary Operators , Relational Operators , Logical operators (&& and ||).
- Some operators cannot be overloaded which include sizeof operator, typeid, Scope resolution (::), Class member access operator (.), Ternary or conditional operator (?:).
- These operators cannot be overloaded because it can cause serious programming issues or errors and also it is syntactically not possible.
- Some operators cannot be overloaded using the friend functions but can be overloaded by member functions. Examples are, Assignment operator(=), Subscript operator [], Function call operator (()), Arrow operator or dereferencing operator (->).