Why Main Method is Static in Java?
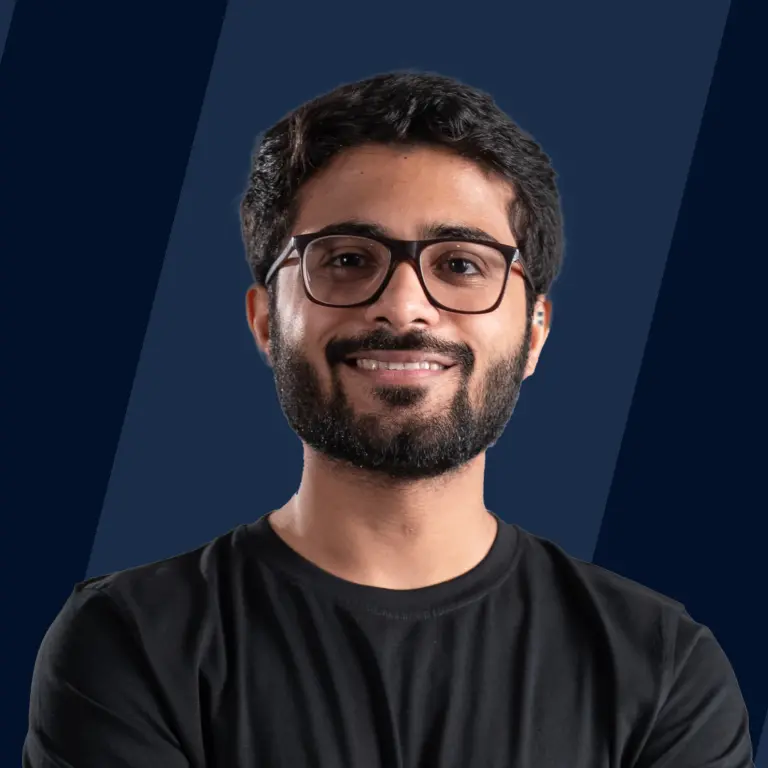
The main() method is the first method you will encounter when studying Java programming and is a standard method that the JVM uses to begin the execution of any Java application. There is no object of the class existing when the Java runtime starts. This is why the main() method must be static for the JVM to load the class into memory and call the main function. If the main method is not static, JVM will be unable to call it since no object of the class is present.
-
The main() method in Java programs is the point from which the program begins its execution, or simply the entrance point of Java programs. So, the compiler must call the main() method first.
-
The JVM or java compiler looks for a public static void main(String args[]) method in that class, and the signature of the main method must be in a specified format for the JVM to recognize it as its entry point. If we update the method's signature, the program will throw the error NoSuchMethodError:main and terminate. As a result, it is one of the most significant Java methods, and a good understanding of it is essential.
-
The java.exe program is used to execute the Java program. Java.exe subsequently makes JNI (Java Native Interface) calls, which load the JVM. The command line is parsed by java.exe, a new String array is created, and the main() method is called. The main method is attached to a daemon thread, which is destroyed only when the Java program terminates execution.
-
It is not possible to change the syntax of the main() method. The one and only thing we can modify is the String array argument's name. The main() method has the following syntax:
- The main() method should be declared static so that the JVM (Java Virtual Machine) can call it without having to create an instance of the class containing the main() method. An instance of a class in java is the object of that class itself. It is sometimes referred to as a class instance or a class object. An instance is a single object of a specific class. For example, an object obj that belongs to the class Circle is an instance (object) of the class Circle.
- If the main method is not declared static, the JVM has to create an instance of the main Class, and because the constructor might be overloaded and include arguments, there will be no reliable and consistent way for the JVM to find the main method in Java.
- Anything specified in a Java class is of the reference type and must be generated before being used. At the same time, static methods and static data are loaded into a separate memory inside the JVM called context, which is created when a class is loaded. The JVM context will load the main method if it is static, making it available for execution.
Example:
Output of the above code:
The given code has two classes Test and Java. The Test class contains the main method(which is the entry point for Java applications), which calls the static method getInfo() of the Java class. The Java class has a static method named getInfo(), which prints a message to the console. When the code is executed, the getInfo() method is called, and the message is printed to the console.
Divide the Syntax of the main() Method into Several Parts
public
- It is an access modifier of the main() method that specifies by whom and from where the method can be accessed. We create the main() method with a public access specifier so that it can be executed by any program (globally available). Since it is not a part of the current class, it is made public so that JVM can call it from outside the class. Therefore, it is necessary to make the main() method public.
- private, protected, and public are just a few of the access modifiers that Java specifies. Any method or variable can be accessed from outside the class if it has been declared as public. Since the main method is declared public in Java, JVM can easily access and execute. If we declare the main() method as non-public, it will throw the following error:
Example:
Output of the above code:
static
- The term static is used in the main() method to make it a class-related method. The main() method is declared static so that JVM can call it without creating an instance of the class containing the main() method. We must declare the main() function static as no class object is present when the java runtime starts. JVM can then load the class into the main memory and invoke the main() method.
- Initiating the main() method as static also prevents the JVM from wasting memory that would have been utilized by the object specified only for invoking the main() method. As a result, if we specify the main() method as non-static, JVM will be unable to invoke it and will throw the desired error:
Example:
Output of the above code:
void
- Each method in java has a different return type, such as Integer, Double, Boolean, String, etc. A Java main() function does not return anything to keep things simple and has the return type void.
- After executing the main() method, the program will be terminated, and returning anything from the main() method is useless because the JVM cannot do anything with the return value. The following error will be thrown if we do not provide the main() method with a void return type:
Example:
Output of the above code:
main()
It is considered the name of the main() method in Java and isn't a keyword. The JVM looks for this identifier as the starting point of the Java program. The method should be declared as main, and we cannot modify it. The following error will be thrown if we try to change the name of the method():
Example:
Output of the above code:
String[] args or String args[]
- It is an array of type java.lang.string class that accepts a single string array argument which is ofter known as Java command-line arguments. The args is the name of the String array, but it is not fixed as the user may specify any other name in its place.
- In addition to the above-mentioned signature, you can call the main method using either public static void main(String args[]) or public static void main(String... args), as both are acceptable. The main method gets invoked if the main method's formal parameter matches that of an array of Strings. Let's look at an example to see how the command-line option works.
Example:
Output of the above code:
Reasons behind Defining the main() Method as Static
It is not possible to invoke a function without constructing an instance of its class, as there is no object of a class when the JVM starts. The main() function is declared static such that the class can be loaded by JVM into the main memory.
- Every Java program begins with the main() function. The main() method is essential because the compiler begins executing a program from the main() function only.
- The JVM should instantiate the class if the main() function is non-static.
- JVM (Java Virtual Machine) can simply invoke static methods without generating an instance of the class by utilizing only the class name.
- The main() function must be declared static, public, and must have a void return type. The JVM will be unable to run the java program and will throw an error if we do not declare it as static and public or if we do not provide it with a void return type.
Conclusion
- The main method serves as the starting point for each Core Java program and is always executed first. If the JVM cannot find the main method of a class with a predefined signature, it throws NoSuchMethodException:main error.
- If the main method is not declared static, the JVM has to create an instance of the main Class, and because the constructor might be overloaded and include arguments, there will be no reliable and consistent way for the JVM to find the main method in Java.
- The main method is executed on a special thread known as the "main" thread. Your Java program will run until your main thread or any non-daemon thread generated from the main method exits.
- Since Java 1.5, you can declare the main method with varargs syntaxes, such as public static void main (String... args).